


How to remove elements from an array until the passed function returns true in JavaScript?
In JavaScript, there are various ways to remove elements from an array until the passed function returns true. In this tutorial we will go over 3 methods in detail.
Using Array.prototype.filter()
The Array.prototype.filter() method can be used to remove elements from an array until the passed function returns true. See the Array filter() method for more details.
Example 1
The following code shows how to use this method -
<html> <head> <title>Examples</title> </head> <body> <div id="array"></div> <div id="result"></div> <script> var arr = [1,2,3,4,5,6,7,8,9,10]; function remove(item) { return item < 5; } var newArr = arr.filter(remove); document.getElementById("array").innerHTML = "Array: " + arr; document.getElementById("result").innerHTML = "Removed Elements: " + newArr; </script> </body> </html>
As you can see, we have created an array containing numbers from 1 to 10. Then use the filter() method to remove all elements less than 5. Finally, we show the new array with the deleted elements.
Using a for loop
Another way to remove elements from an array until the passed function returns true is to use a for loop.
< h2>Example 2The following code shows how to use this method -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> var arr = [1,2,3,4,5,6,7,8,9,10]; var newArr = []; for(var i=0; i < arr.length; i++) { if(arr[i] < 5) { newArr.push(arr[i]); } else { break; } } document.getElementById("result").innerHTML = newArr </script> </body> </html>
As you can see, we have again created a array. We then use a for loop to iterate over each element in the array. If the current element is less than 5, we add it to the new array. Otherwise, we are out of the loop. Finally, we display the new array.
Using Array.prototype.slice()
Another way to remove elements from an array until the passed function returns true is to use the Array .prototype.slice() method. Please refer to the array slicing method for details.
Example 3
The following code shows how to use this method -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> var arr = [1,2,3,4,5,6,7,8,9,10]; var newArr = arr.slice(0,5); document.getElementById("result").innerHTML = newArr </script> </body> </html>
As you can see, we have again created a array. We then use the slice() method to extract the first 5 elements from the array. Finally, we display the new array.
The above is the detailed content of How to remove elements from an array until the passed function returns true in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


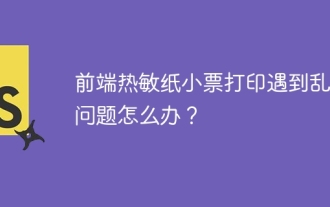
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
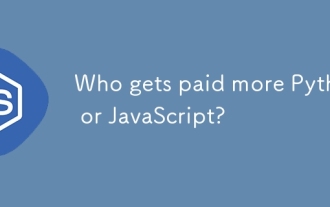
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
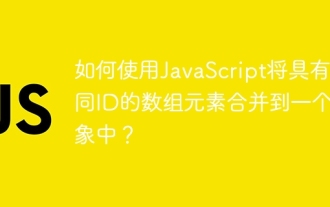
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
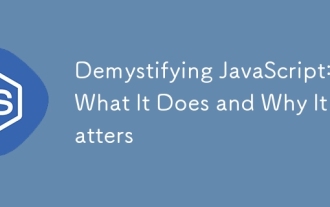
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
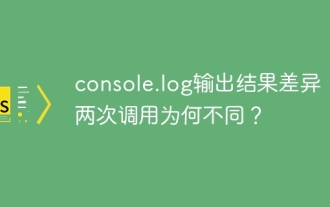
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
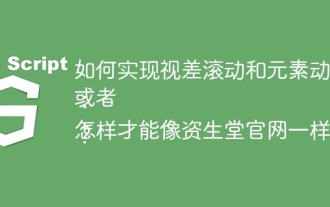
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
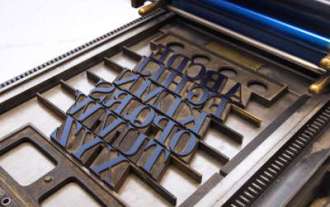
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
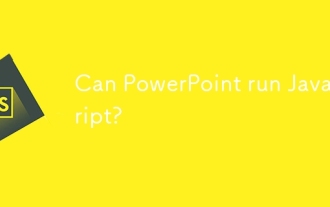
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
