Minimum number of adjacent swaps required to reverse a string
Given a string str, we can reverse the string by just swapping adjacent characters. We need to find the minimum number of moves required to reverse the string, just by swapping adjacent characters. We will implement two methods to find the required solution and provide an explanation and implementation of the code.
ExampleExample
Input1: string str1 = “shkej”
Output: 10
Explanation
is:Explanation
First, we will move the last character to the first position, which will require 4 swaps, and then the string will become "jshke". Then we will move the 'e' to the second position, which will require 3 swaps. Similarly, for 'k', we need two swaps, while for 'h', only 1 swap is required, and the final answer is 10.
Input2: string str1 = “abace”
Output: 6
Explanation
is:Explanation
First we will swap the character at the 2nd index and move it to the last index, this will take 2 swaps and the string will become "abcea". Then we swap 'b' for 'e', it will take 2 swaps and the string will become "aceba". Then perform 2 more exchanges to get the final reversed string, which requires a total of 6 exchanges.
The Chinese translation ofNaive Approach
is:Naive Approach
We've looked at the example above, now let's look at the steps required to implement the correct code.
First, we will create a function that will take the given string as parameter and will return the minimum number of steps required as the return value.
In this function we will create a copy of the string and then reverse it to compare with the original string.
We will create three variables, the first two are used to iterate through the string and the last one is used to store the required number of steps.
By using a while loop, we will iterate through the given string and continue skipping the number of iterations that the current index value is the same as the reversed string.
We will then use a while loop to swap adjacent characters until 'j' reaches 'i', and increment the count on each swap.
Finally, we will return the value of the count and print it out in the main function.
Example
#include <bits/stdc++.h> using namespace std; // function to get the minimum number of swaps int minSwaps(string str){ string temp = str; reverse(temp.begin(), temp.end()); // reversing the string int i = 0, j = 0; int ans = 0; int len = str.size(); while (i <len) { j = i; // find the character that is equal to the current element while (str[j] != temp[i]) { j++; } // iterating util the current i is equal to j while (i < j) { char tempc = str[j]; str[j] = str[j - 1]; str[j - 1] = tempc; j--; ans++; } i++; } return ans; } int main(){ string str = "efabc"; // given string // calling the function to find the minimum number of steps required cout<<"The minimum number of steps required to reverse the given string by swapping the adjacent characters is "<<minSwaps(str)<<endl; return 0; }
Output
The minimum number of steps required to reverse the given string by swapping the adjacent characters is 10
Time and space complexity
The time complexity of the above code is O(N^2), where N is the length of the given string. We use nested while loops to give factors of N during the iteration.
The space complexity of the above code is O(N) because we create an extra string there to store the inversion of the given string.
Note - An alternative approach is possible here, but requires the use of very advanced data structures. The concept of this method is that we can start from the last character and check until the first character meets the condition. Then in theory we could move that character to the last position and mark that position as completed and store that value in a high-level data structure.
Then for each character, we will find the same character appearing from behind, which is not yet tagged, and then increase the count to the total number of elements after it minus the number of tagged elements.
in conclusion
In this tutorial, we implemented a code to find the minimum number of steps required to reverse a given string by swapping only adjacent characters. We used nested while loops and reversed the copy of the given string to find the solution. The time complexity of the above code is O(N^2), where N is the size of the string, and the space complexity is O(N).
The above is the detailed content of Minimum number of adjacent swaps required to reverse a string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


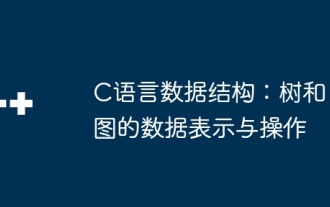
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
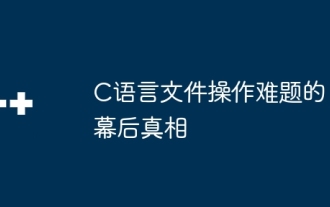
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
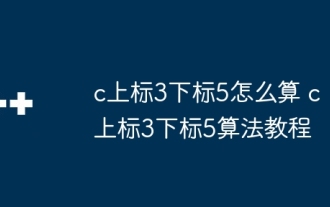
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
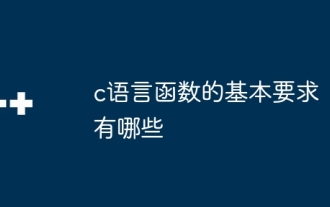
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
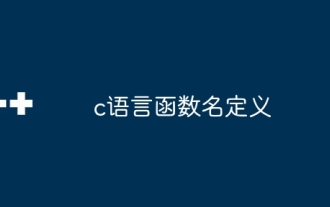
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
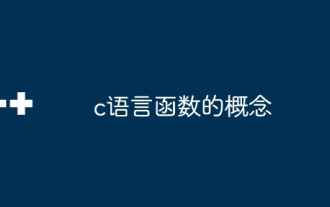
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
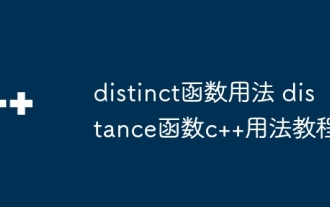
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
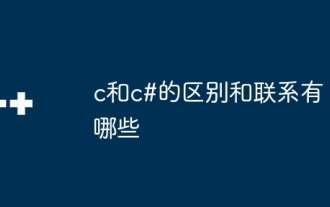
Although C and C# have similarities, they are completely different: C is a process-oriented, manual memory management, and platform-dependent language used for system programming; C# is an object-oriented, garbage collection, and platform-independent language used for desktop, web application and game development.
