


JavaScript program for writing a function to get the Nth node in a linked list
A linked list is a linear data structure in which all nodes are connected to each other by storing the address of the next node. Finding the nth node in a linked list means getting the value of the nth node in a given linked list, which can be done by both iterative and recursive methods.
Example
Given linked list: 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> null Node to find: 3
Output
3
Explanation: The value of the third node is 3.
Iteration method
In this method, we will directly traverse the linked list using while loop until we reach the last node or the desired node.
Example
// class to create the structure of the nodes class Node{ constructor(data){ this.value = data; this.next = null; } } // function to print the linked list function print(head){ var temp = head; var ans = "" while(temp.next != null){ ans += temp.value; ans += " -> " temp = temp.next } ans += temp.value ans += " -> null" console.log(ans) } // function to add data in linked list function add(data, head, tail){ return tail.next = new Node(data); } // function to find the nth node function findNode(head, node){ var temp = head; while(node > 1 && temp != null){ node--; temp = temp.next; } return temp; } // defining linked list var head = new Node(1) var tail = head tail = add(2,head, tail) tail = add(3,head, tail) tail = add(4,head, tail) tail = add(5,head, tail) tail = add(6,head, tail) tail = add(7,head, tail) tail = add(8,head, tail) // printing linked list console.log("The given linked list is: ") print(head) // defining node to get var node = 5; // calling function to get the nth node; var nth_node = findNode(head,node) if(nth_node == null){ console.log("The given linked list does not have the Nth Node"); } else{ console.log("The value present at the nth node is: " + nth_node.value); }
Output
The given linked list is: 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> null The value present at the nth node is: 5
Time and space complexity
The time complexity of the above code is O(N), where N is the size of the given linked list. The number given here may be smaller than the size of the given linked list, but in the worst case we will only traverse the length of the linked list.
Here we are not using any extra space, which means the time complexity of the above code is O(1).
Recursive method
In this method, we will use recursive calls instead of while loops to traverse the linked list and implement the previous logic.
Example
// class to create the structure of the nodes class Node{ constructor(data){ this.value = data; this.next = null; } } // function to print the linked list function print(head){ var temp = head; var ans = "" while(temp.next != null){ ans += temp.value; ans += " -> " temp = temp.next } ans += temp.value ans += " -> null" console.log(ans) } // function to add data in linked list function add(data, head, tail){ return tail.next = new Node(data); } // function to find the nth node function findNode(head, node){ if(node == 1){ return head; } else if(head == null){ return null; } else{ return findNode(head.next, node-1); } } // defining linked list var head = new Node(1) var tail = head tail = add(2,head, tail) tail = add(3,head, tail) tail = add(4,head, tail) tail = add(5,head, tail) tail = add(6,head, tail) tail = add(7,head, tail) tail = add(8,head, tail) // printing linked list console.log("The given linked list is: ") print(head) // defining node to get var node = 9; // calling function to get the nth node; var nth_node = findNode(head,node) if(nth_node == null){ console.log("The given linked list does not have the " + node + " number of nodes"); } else{ console.log("The value present at the nth node is: " + nth_node.value); }
Output
The given linked list is: 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> null The given linked list does not have the 9 number of nodes
Time and space complexity
The time and space complexity of the above code are the same, both are O(N), where N is the number of nodes in the given linked list. The space here is due to recursive calls.
in conclusion
In this tutorial, we implemented a JavaScript program to find the nth node in a given linked list. If the nth node does not exist, then we print that it does not exist, otherwise we print the value that exists at that node. We implemented two methods, one is iterative using while loop and the other is recursive method. The time complexity of both is O(N), but iteration is better since it requires no extra space.
The above is the detailed content of JavaScript program for writing a function to get the Nth node in a linked list. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
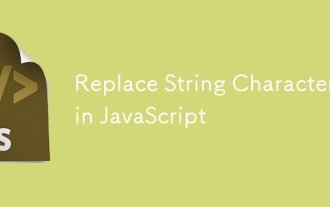
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
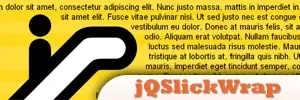
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
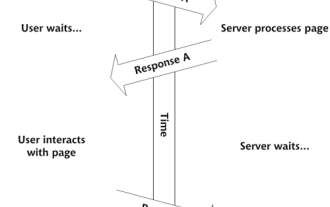
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
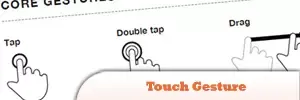
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
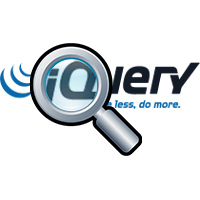
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
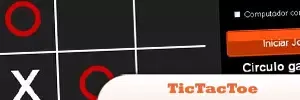
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
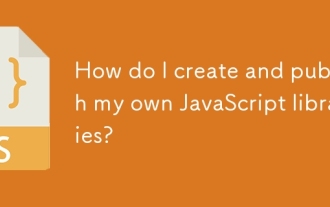
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
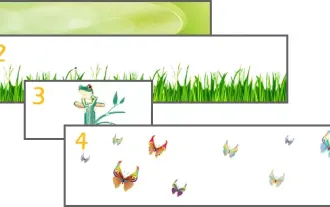
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
