


How to use Webman for user authentication and permission management on the website
How to use Webman for user authentication and permission management of the website
In modern web applications, user authentication and permission management are very important parts. Webman is a popular PHP framework that provides a set of simple and powerful tools that can help us implement user authentication and permission management functions for the website. This article will introduce how to use Webman to implement these functions and provide relevant code examples.
- Implementation of user authentication function:
User authentication refers to verifying whether the user's identity is legal. User name and password can be used for verification. In Webman, we can use Session to record the user's login status.
First, we need to create a login page to allow users to enter their username and password. Next, we need to write a controller method that handles login requests. In this method, we can determine whether the user has successfully logged in by comparing the username and password stored in the database.
The sample code is as follows:
// 登录页面 public function login() { return view('login'); } // 处理登录请求 public function doLogin() { $username = $_POST['username']; $password = $_POST['password']; // 比对用户名和密码 if ($username == 'admin' && $password == '123456') { // 登录成功,将用户信息存入Session $_SESSION['user'] = ['username' => $username]; // 跳转到首页 redirect('/'); } else { // 登录失败,返回错误信息 return view('login', ['error' => '用户名或密码错误']); } }
In other pages that require authentication, we can determine whether the user has logged in by checking whether user information exists in the Session:
// 需要认证的页面 public function userPage() { // 检查Session中是否存在用户信息 if (isset($_SESSION['user'])) { return view('user_page'); } else { // 用户未登录,跳转到登录页面 redirect('/login'); } }
- Implementation of authority management function:
In addition to user authentication, authority management is also an important function. Webman provides a set of simple and flexible permission management tools that can help us implement various permission control strategies.
First, we need to create a table in the database to store the relationship between users and permissions. For example, we can create a table named "users_roles" to store the correspondence between users and roles.
Next, we need to write a controller method to check whether the user has a specific permission. In this method, we can query the database to determine whether the user has the corresponding permissions.
The sample code is as follows:
// 检查用户权限 public function checkPermission($permission) { if (isset($_SESSION['user'])) { $username = $_SESSION['user']['username']; // 查询用户角色的权限 $permissions = DB::table('users_roles') ->join('roles_permissions', 'users_roles.role_id', '=', 'roles_permissions.role_id') ->join('permissions', 'roles_permissions.permission_id', '=', 'permissions.id') ->where('users_roles.username', $username) ->pluck('permissions.name') ->toArray(); // 检查用户是否具有权限 if (in_array($permission, $permissions)) { return true; } } return false; }
In other places where permission control is required, we can call this method to determine whether the user has the corresponding permissions:
// 需要权限控制的页面 public function adminPage() { // 检查用户是否具有"admin"权限 if ($this->checkPermission('admin')) { return view('admin_page'); } else { // 没有权限,跳转到首页 redirect('/'); } }
Through the above method, We can use Webman to implement the user authentication and rights management functions of the website. Webman provides simple yet powerful tools to help us accomplish these tasks easily. Hope this article helps you!
The above is the detailed content of How to use Webman for user authentication and permission management on the website. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


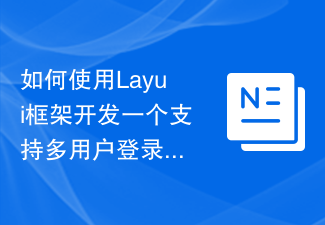
How to use the Layui framework to develop a permission management system that supports multi-user login Introduction: In the modern Internet era, more and more applications need to support multi-user login to achieve personalized functions and permission management. In order to protect the security of the system and the privacy of data, developers need to use certain means to implement multi-user login and permission management functions. This article will introduce how to use the Layui framework to develop a permission management system that supports multi-user login, and give specific code examples. Preparation before starting development
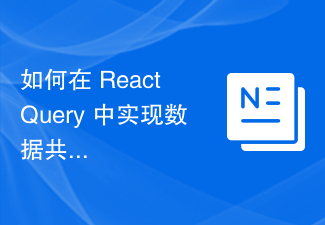
How to implement data sharing and permission management in ReactQuery? Advances in technology have made data management in front-end development more complex. In the traditional way, we may use state management tools such as Redux or Mobx to handle data sharing and permission management. However, after the emergence of ReactQuery, we can use it to deal with these problems more conveniently. In this article, we will explain how to implement data sharing and permissions in ReactQuery
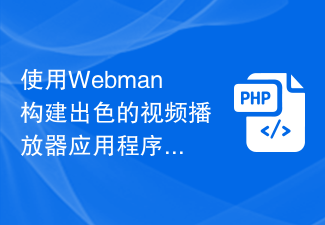
Build an excellent video player application using Webman With the rapid development of the Internet and mobile devices, video playback has become an increasingly important part of people's daily lives. Building a powerful, stable and efficient video player application is the pursuit of many developers. This article will introduce how to use Webman to build an excellent video player application, and attach corresponding code examples to help readers get started quickly. Webman is a lightweight web based on JavaScript and HTML5 technology
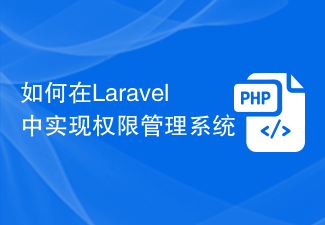
How to implement a permission management system in Laravel Introduction: With the continuous development of web applications, the permission management system has become one of the basic functions of many applications. Laravel, as a popular PHP framework, provides a wealth of tools and functions to implement permission management systems. This article will introduce how to implement a simple and powerful permission management system in Laravel and provide specific code examples. 1. Design ideas of the permission management system When designing the permission management system, the following key points need to be considered: roles and
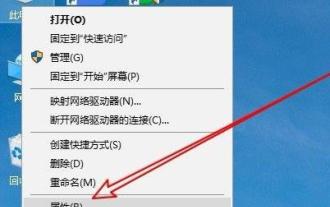
The problem that temporary folders cannot be installed without write permissions is a headache for many users. In fact, the operation is not very troublesome. You only need to enter your advanced menu to make changes. Let’s see how to solve the problem of no write permissions. The temporary folder cannot be installed without write permission: 1. First, right-click This Computer on the desktop, and then click "Properties". 2. Then click "Advanced System Settings" below. 3. Then click "Environment Variables" at the bottom of the window. 4. After that, you can open the environment variables window, click on the tmp file and select "Edit". 5. Then click "Browse Files" in the window that opens. 6. Set the new variable folder and click OK. 7. Finally wait until success.
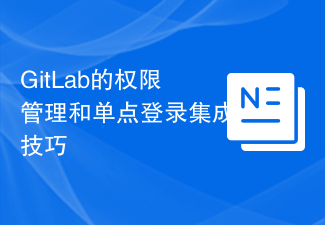
GitLab's permission management and single sign-on integration tips require specific code examples Overview: In GitLab, permission management and single sign-on (SSO) are very important functions. Permission management can control users' access to code repositories, projects, and other resources, while single sign-on integration can provide a more convenient user authentication and authorization method. This article will introduce how to perform permission management and single sign-on integration in GitLab. 1. Permission Management Project Access Permission Control In GitLab, projects can be set to private
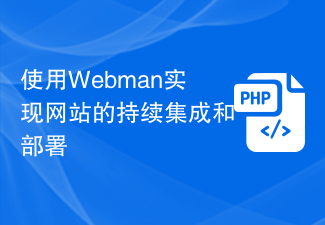
Using Webman to achieve continuous integration and deployment of websites With the rapid development of the Internet, the work of website development and maintenance has become more and more complex. In order to improve development efficiency and ensure website quality, continuous integration and deployment have become an important choice. In this article, I will introduce how to use the Webman tool to implement continuous integration and deployment of the website, and attach some code examples. 1. What is Webman? Webman is a Java-based open source continuous integration and deployment tool that provides
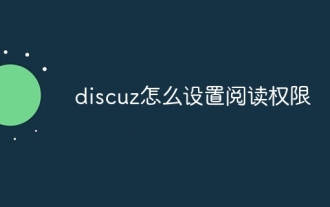
Set reading permissions in discuz: 1. Click on the section to enter the section management interface; 2. Click the "Edit" button behind a certain section; 3. In the opened interface, click "Permissions Related" and check the "Browse Section" column and Check box crossing the "User Group" row; 4. Click Submit to complete the setting of reading permissions.
