How to shape-mark images using Python
How to use Python to mark images with shapes
Introduction:
In the fields of image processing and computer vision, objects in images are marked and Identification is an important task. The shape of the marked object can be used for applications such as object detection, edge detection, and contour extraction. This article will introduce how to use Python and the OpenCV library to label images with shapes.
Step 1: Install the required libraries
Before we begin, we need to install the Python and OpenCV libraries. You can install the OpenCV library through the pip command. The specific command is as follows:
pip install opencv-python
Step 2: Import the required libraries
In the code, we need to import the OpenCV library and some other auxiliary libraries. The specific code is as follows:
import cv2 import numpy as np
Step 3: Read the image file
Use the imread()
function of the OpenCV library to read the image file. The specific code is as follows:
img = cv2.imread('image.jpg')
Step 4: Convert the image to grayscale image
Before doing shape labeling, we need to convert the image to grayscale image. This can be achieved using the cvtColor()
function of the OpenCV library. The specific code is as follows:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
Step 5: Threshold segmentation
Threshold segmentation of the grayscale image can obtain a binary image, which facilitates subsequent shape marking operations. This can be achieved using the threshold()
function of the OpenCV library. The specific code is as follows:
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
Step 6: Find contours
Use the findContours()
function of the OpenCV library to find contours in the image. The specific code is as follows:
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
Step 7: Draw the contour
Use the drawContours()
function of the OpenCV library to draw the contour on the image. The specific code is as follows:
cv2.drawContours(img, contours, -1, (0, 255, 0), 3)
Step 8: Display the results
Use the imshow()
function of the OpenCV library to display the processed image. The specific code is as follows:
cv2.imshow('Contours', img) cv2.waitKey(0) cv2.destroyAllWindows()
Full code example:
import cv2 import numpy as np # 读取图像文件 img = cv2.imread('image.jpg') # 将图像转换为灰度图像 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 进行阈值分割 _, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY) # 查找轮廓 contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) # 绘制轮廓 cv2.drawContours(img, contours, -1, (0, 255, 0), 3) # 显示结果 cv2.imshow('Contours', img) cv2.waitKey(0) cv2.destroyAllWindows()
Conclusion:
This article introduces how to use Python and the OpenCV library to mark images with shapes. By implementing the code example, the contours of objects in the image can be extracted and marked to facilitate subsequent image processing and computer vision applications. Readers can make corresponding adjustments and expansions according to their own needs and actual conditions. Hope this article is helpful to readers!
The above is the detailed content of How to shape-mark images using Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


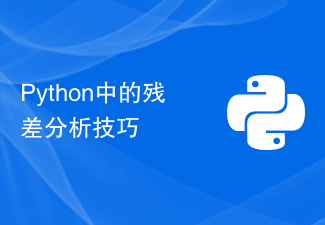
Python is a widely used programming language, and its powerful data analysis and visualization capabilities make it one of the preferred tools for data scientists and machine learning engineers. In these applications, residual analysis is a common technique used to evaluate model accuracy and identify any model bias. In this article, we will introduce several ways to use residual analysis techniques in Python. Understanding Residuals Before introducing residual analysis techniques in Python, let us first understand what residuals are. In statistics, the residual is the difference between the actual observed value and
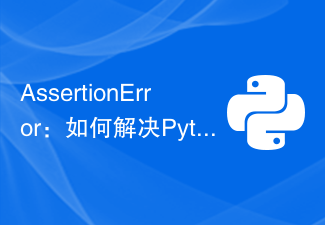
Assertions in Python are a useful tool for programmers to debug their code. It is used to verify that the internal state of the program meets expectations and raise an assertion error (AssertionError) when these conditions are false. During the development process, assertions are used during testing and debugging to check whether the status of the code matches the expected results. This article will discuss the causes, solutions, and how to correctly use assertions in your code. Cause of assertion error Assertion error pass
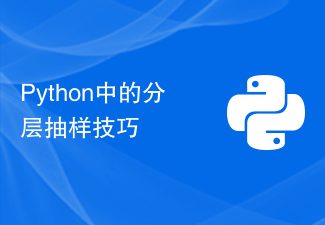
Stratified Sampling Technique in Python Sampling is a commonly used data collection method in statistics. It can select a portion of samples from the data set for analysis to infer the characteristics of the entire data set. In the era of big data, the amount of data is huge, and using full samples for analysis is both time-consuming and not economically practical. Therefore, choosing an appropriate sampling method can improve the efficiency of data analysis. This article mainly introduces stratified sampling techniques in Python. What is stratified sampling? In sampling, stratified sampling
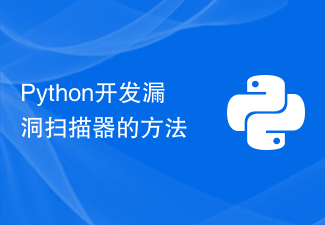
Overview of how to develop a vulnerability scanner through Python In today's environment of increasing Internet security threats, vulnerability scanners have become an important tool for protecting network security. Python is a popular programming language that is concise, easy to read and powerful, suitable for developing various practical tools. This article will introduce how to use Python to develop a vulnerability scanner to provide real-time protection for your network. Step 1: Determine Scan Targets Before developing a vulnerability scanner, you need to determine what targets you want to scan. This can be your own network or anything you have permission to test
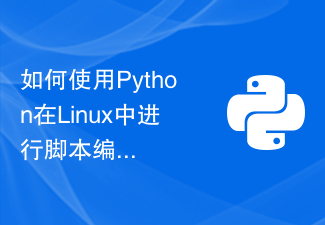
How to use Python to write and execute scripts in Linux In the Linux operating system, we can use Python to write and execute various scripts. Python is a concise and powerful programming language that provides a wealth of libraries and tools to make scripting easier and more efficient. Below we will introduce the basic steps of how to use Python for script writing and execution in Linux, and provide some specific code examples to help you better understand and use it. Install Python
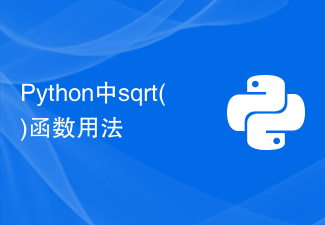
Usage and code examples of the sqrt() function in Python 1. Function and introduction of the sqrt() function In Python programming, the sqrt() function is a function in the math module, and its function is to calculate the square root of a number. The square root means that a number multiplied by itself equals the square of the number, that is, x*x=n, then x is the square root of n. The sqrt() function can be used in the program to calculate the square root. 2. How to use the sqrt() function in Python, sq
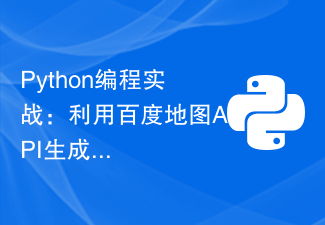
Python programming practice: How to use Baidu Map API to generate static map functions Introduction: In modern society, maps have become an indispensable part of people's lives. When working with maps, we often need to obtain a static map of a specific area for display on a web page, mobile app, or report. This article will introduce how to use the Python programming language and Baidu Map API to generate static maps, and provide relevant code examples. 1. Preparation work To realize the function of generating static maps using Baidu Map API, I
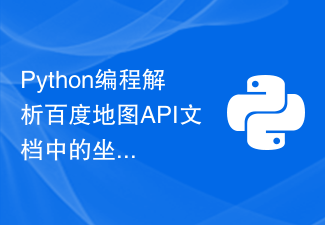
Python programming to analyze the coordinate conversion function in Baidu Map API document Introduction: With the rapid development of the Internet, the map positioning function has become an indispensable part of modern people's lives. As one of the most popular map services in China, Baidu Maps provides a series of APIs for developers to use. This article will use Python programming to analyze the coordinate conversion function in Baidu Map API documentation and give corresponding code examples. 1. Introduction In development, we sometimes involve coordinate conversion issues. Baidu Map AP
