How to crop and zoom images using PHP
How to crop and zoom images using PHP
In today’s digital age, working with images is a very common task. Whether for website development or mobile applications, images often need to be cropped and scaled. This article will introduce how to use PHP for image cropping and scaling, and provide relevant code examples.
Overview
Before we begin, we need to make sure that the GD library extension for PHP is installed. The GD library is a popular graphics library that provides a set of functions to process images. You can use the phpinfo() function to confirm whether the GD library is installed.
Picture cropping
Picture cropping refers to the process of cutting out a specified area from the original picture. With cropping, we can cut out the specific parts we want. Below is a sample code that demonstrates how to use the GD library to crop an image.
<?php // 原始图片路径 $sourceImagePath = 'path/to/source/image.jpg'; // 创建一个新的图片资源 $sourceImage = imagecreatefromjpeg($sourceImagePath); // 剪裁的起始坐标 $x = 100; $y = 100; // 剪裁的宽度和高度 $width = 200; $height = 200; // 创建一个新的剪裁后的图片资源 $croppedImage = imagecreatetruecolor($width, $height); // 剪裁图片 imagecopy($croppedImage, $sourceImage, 0, 0, $x, $y, $width, $height); // 保存剪裁后的图片 $savePath = 'path/to/save/cropped/image.jpg'; imagejpeg($croppedImage, $savePath); // 释放资源 imagedestroy($sourceImage); imagedestroy($croppedImage); echo '图片剪裁成功,新图片保存路径为:' . $savePath; ?>
In the above sample code, we first created an original image resource through the imagecreatefromjpeg()
function. Then, we specify the starting coordinates and width height to be clipped. Next, we create a new cropped image resource using the imagecreatetruecolor()
function. Finally, we use the imagecopy()
function to crop the original image into a new image resource, and use the imagejpeg()
function to save the cropped image.
Picture scaling
Picture scaling refers to the process of changing the size of the picture. With zoom, we can resize the image as needed. Below is a sample code that demonstrates how to use the GD library to scale an image.
<?php // 原始图片路径 $sourceImagePath = 'path/to/source/image.jpg'; // 创建一个新的图片资源 $sourceImage = imagecreatefromjpeg($sourceImagePath); // 缩放后的宽度和高度 $newWidth = 400; $newHeight = 400; // 创建一个新的缩放后的图片资源 $scaledImage = imagescale($sourceImage, $newWidth, $newHeight); // 保存缩放后的图片 $savePath = 'path/to/save/scaled/image.jpg'; imagejpeg($scaledImage, $savePath); // 释放资源 imagedestroy($sourceImage); imagedestroy($scaledImage); echo '图片缩放成功,新图片保存路径为:' . $savePath; ?>
In the above sample code, we first created an original image resource through the imagecreatefromjpeg()
function. Then, we specify the scaled width and height. Next, we use the imagescale()
function to create a new scaled image resource. Finally, we use the imagejpeg()
function to save the scaled image.
Summary
By using the GD library extension and corresponding functions, we can easily use PHP for image cropping and scaling. This article provides relevant code examples, hoping to help you when processing images. If you want to learn more about other functions and methods of the GD library, please consult the official documentation.
The above is the detailed content of How to crop and zoom images using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


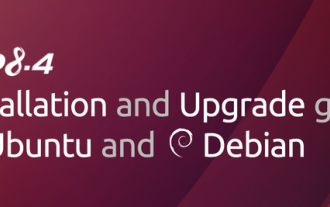
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
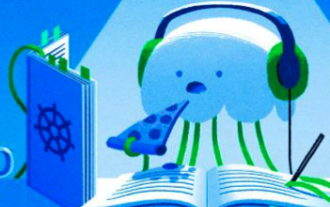
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
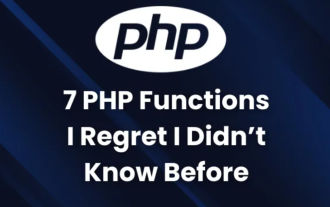
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
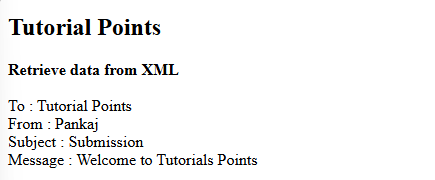
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
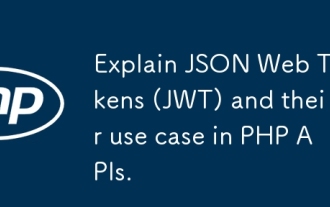
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
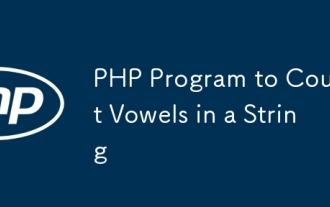
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
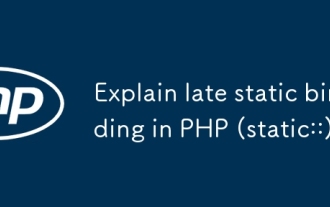
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
