Laravel Best Practices: Building Maintainable and Scalable Applications
Laravel Best Practices: Building Maintainable and Scalable Applications
Introduction:
Laravel is a popular PHP framework that provides many Powerful features and tools help developers build high-quality web applications. However, to ensure the maintainability and scalability of the application, we need to follow some best practices. This article will introduce some best practices regarding the Laravel framework and show how to implement them with code examples.
- Using Laravel’s naming convention
The Laravel framework has a set of naming conventions that can help us read and understand the code more easily. For example, model classes should use the singular form, and controller classes should use the plural form. Following these conventions will make your code more consistent and make it easier for other developers to understand and maintain your application.
The following is a code example using Laravel's naming convention:
// 模型类 class User extends Model { // ... } // 控制器类 class UsersController extends Controller { // ... }
- Using Laravel's routing function
Laravel provides a powerful routing function that can help us Define and manage your application's routes. When designing routes, you should follow a RESTful style and use a meaningful URL structure. Additionally, related routes can be organized into a controller to improve code readability and maintainability.
The following is a code example that takes advantage of Laravel's routing capabilities:
// 定义路由 Route::get('/users', 'UsersController@index'); Route::post('/users', 'UsersController@store'); Route::get('/users/{id}', 'UsersController@show'); Route::put('/users/{id}', 'UsersController@update'); Route::delete('/users/{id}', 'UsersController@destroy'); // UsersController类 class UsersController extends Controller { public function index() { // ... } public function store() { // ... } public function show($id) { // ... } public function update($id) { // ... } public function destroy($id) { // ... } }
- Using Laravel's validation capabilities
Input validation is an important component of building secure web applications part. Laravel provides powerful validation features that can help us easily validate user input. Using Laravel's validation features can reduce code redundancy and improve code maintainability.
The following is a code example using Laravel's validation function:
// 定义验证规则 $rules = [ 'name' => 'required|string|max:255', 'email' => 'required|email|unique:users', 'password' => 'required|string|min:6|confirmed', ]; // 执行验证 $validator = validator(request()->all(), $rules); if ($validator->fails()) { // 验证失败的逻辑 } else { // 验证成功的逻辑 }
- Database migration and population using Laravel
Database migration is a way to manage database schema changes method, while fill is used to add basic data to the database. Laravel provides database migration and population functions that can help us manage database structure and data more easily.
The following is a code example using Laravel database migration and population functionality:
// 创建迁移文件 php artisan make:migration create_users_table // 编辑迁移文件 public function up() { Schema::create('users', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } // 执行迁移 php artisan migrate // 创建填充文件 php artisan make:seeder UsersTableSeeder // 编辑填充文件 public function run() { DB::table('users')->insert([ 'name' => 'John Doe', 'email' => 'john@example.com', 'password' => bcrypt('password'), ]); } // 执行填充 php artisan db:seed --class=UsersTableSeeder
Conclusion:
By following the above Laravel best practices, we can build maintainable and Scalable applications. These practices include using Laravel's naming conventions, leveraging routing features, using validation features, and database migration and population features. I hope this article can provide valuable guidance to developers and help them better utilize the Laravel framework to build high-quality web applications.
The above is the detailed content of Laravel Best Practices: Building Maintainable and Scalable Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
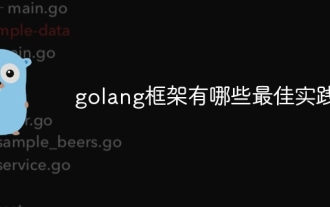
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.
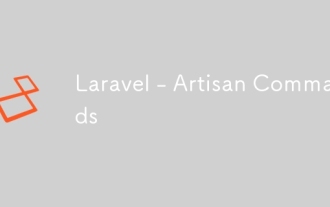
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
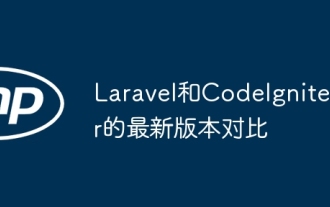
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
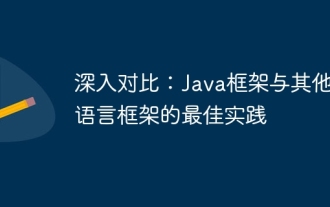
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
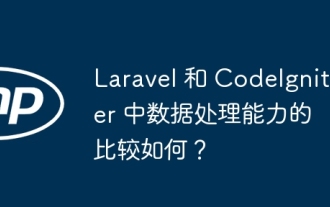
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
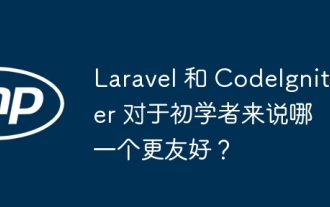
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
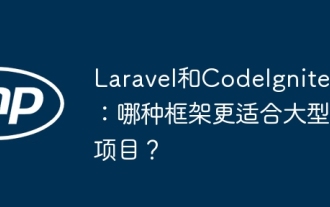
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
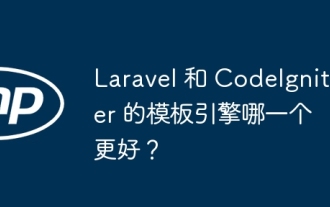
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
