Solve C++ compilation error: 'incompatible types', how to solve it?
Solve C compilation error: 'incompatible types', how to solve it?
During the development process of C, we often encounter error messages given by the compiler. One common type of error is "incompatible types". This error message indicates that there is a type mismatch in the program, which may be inconsistent variable types, mismatched function parameter types, etc. This article will introduce several common type incompatibility errors and give corresponding solutions.
Error type one: Variable type mismatch
A type mismatch error occurs when we assign a variable to another variable of a different type. For example, assigning an integer to a string variable will cause an "incompatible types" error.
The following is a sample code:
int num = 10; string str = num; // 这里会产生'incompatible types'错误
To solve this error, type conversion is required. In C, you can use the static_cast
function for type conversion. In this example, we can convert an integer to a string as follows:
int num = 10; string str = static_cast<string>(num);
Error type two: Function parameter type mismatch
When we call a function, if the incoming actual participation If the formal parameter types of the function declaration do not match, the "incompatible types" error will also occur.
The following is a sample code:
void printNumber(string num) { cout << "Number: " << num << endl; } int main() { int num = 10; printNumber(num); // 这里会产生'incompatible types'错误 return 0; }
To solve this error, you need to ensure that the actual parameter type passed in is consistent with the formal parameter type declared by the function. In this example, we need to convert an integer to a string as follows:
void printNumber(int num) { cout << "Number: " << num << endl; } int main() { int num = 10; printNumber(num); return 0; }
Error type three: Array type mismatch
When we try to assign an array to another array of a different type , the "incompatible types" error will also occur.
The following is a sample code:
int main() { int arr1[] = {1, 2, 3, 4, 5}; char arr2[] = arr1; // 这里会产生'incompatible types'错误 return 0; }
To solve this error, you can use a loop to traverse the original array and copy each element to the new array. In this example, we can copy the integer array to the character array as shown below:
int main() { int arr1[] = {1, 2, 3, 4, 5}; char arr2[sizeof(arr1)]; // 新数组的大小与原数组一致 for (int i = 0; i < sizeof(arr1); i++) { arr2[i] = static_cast<char>(arr1[i]); } return 0; }
Through the above example, we can see the common ways to solve the "incompatible types" error. Whether it is a variable type mismatch, a function parameter type mismatch, or an array type mismatch, it can all be solved through type conversion. However, be aware that type conversions may lose some precision or information, so use type conversions with caution.
In C development, mastering these methods to solve "incompatible types" errors will help make it easier to debug and fix type mismatch problems in the code and improve development efficiency. Hope this article is helpful to you!
The above is the detailed content of Solve C++ compilation error: 'incompatible types', how to solve it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


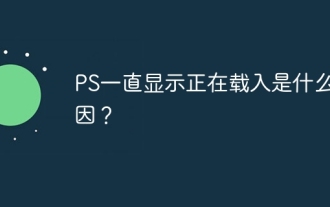
PS "Loading" problems are caused by resource access or processing problems: hard disk reading speed is slow or bad: Use CrystalDiskInfo to check the hard disk health and replace the problematic hard disk. Insufficient memory: Upgrade memory to meet PS's needs for high-resolution images and complex layer processing. Graphics card drivers are outdated or corrupted: Update the drivers to optimize communication between the PS and the graphics card. File paths are too long or file names have special characters: use short paths and avoid special characters. PS's own problem: Reinstall or repair the PS installer.
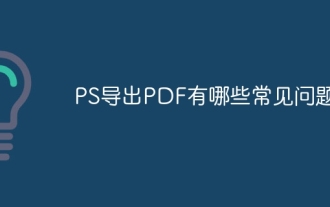
Frequently Asked Questions and Solutions when Exporting PS as PDF: Font Embedding Problems: Check the "Font" option, select "Embed" or convert the font into a curve (path). Color deviation problem: convert the file into CMYK mode and adjust the color; directly exporting it with RGB requires psychological preparation for preview and color deviation. Resolution and file size issues: Choose resolution according to actual conditions, or use the compression option to optimize file size. Special effects issue: Merge (flatten) layers before exporting, or weigh the pros and cons.
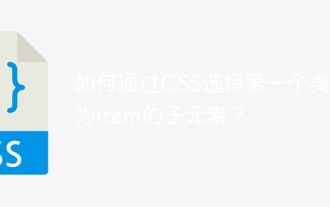
When the number of elements is not fixed, how to select the first child element of the specified class name through CSS. When processing HTML structure, you often encounter different elements...
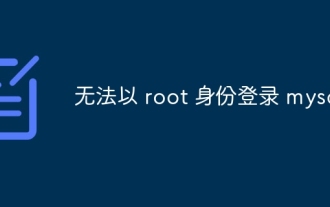
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
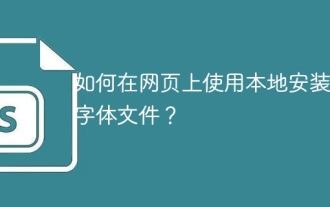
How to use locally installed font files on web pages Have you encountered this situation in web page development: you have installed a font on your computer...
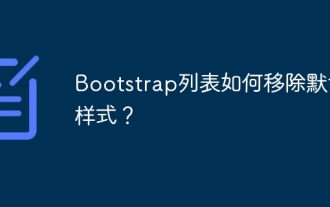
The default style of the Bootstrap list can be removed with CSS override. Use more specific CSS rules and selectors, follow the "proximity principle" and "weight principle", overriding the Bootstrap default style. To avoid style conflicts, more targeted selectors can be used. If the override is unsuccessful, adjust the weight of the custom CSS. At the same time, pay attention to performance optimization, avoid overuse of !important, and write concise and efficient CSS code.
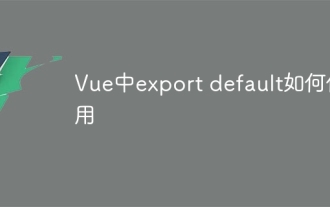
Export default in Vue reveals: Default export, import the entire module at one time, without specifying a name. Components are converted into modules at compile time, and available modules are packaged through the build tool. It can be combined with named exports and export other content, such as constants or functions. Frequently asked questions include circular dependencies, path errors, and build errors, requiring careful examination of the code and import statements. Best practices include code segmentation, readability, and component reuse.
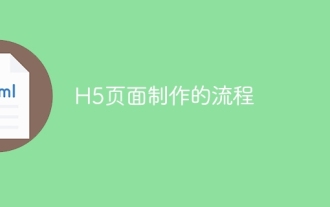
H5 page production process: design: plan page layout, style and content; HTML structure construction: use HTML tags to build a page framework; CSS style writing: use CSS to control the appearance and layout of the page; JavaScript interaction implementation: write code to achieve page animation and interaction; Performance optimization: compress pictures, code and reduce HTTP requests to improve page loading speed.
