Using C++ to realize remote control function of embedded system
Using C to realize the remote control function of embedded systems
With the rapid development of the Internet of Things, embedded systems are becoming an indispensable part of our daily lives. For developers of embedded systems, how to implement remote control functions is an important issue. Using C programming language, we can easily implement the remote control function of embedded systems. This article will describe how to write code in C to implement this functionality, as well as give some sample code.
First, we need to prepare some hardware equipment. As an embedded system, we will use the Arduino development board as an example. Arduino is a very popular open source hardware platform that can easily interface with various sensors and actuators. In order to implement the remote control function, we need to connect a WiFi module to the Arduino development board to enable it to receive and send data over the network.
The following is a sample C code for connecting the WiFi module and implementing the remote control function:
#include <WiFi.h> const char* ssid = "your_SSID"; const char* password = "your_PASSWORD"; WiFiServer server(80); void setup() { Serial.begin(115200); delay(10); // 连接到WiFi网络 Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected."); // 启动服务器 server.begin(); Serial.println("Server started."); } void loop() { // 等待客户端连接 WiFiClient client = server.available(); if (!client) { return; } // 读取客户端请求 String request = client.readStringUntil(''); Serial.println(request); client.flush(); // 发送响应给客户端 client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println(""); client.println("<h1 id="Hello-world">Hello, world!</h1>"); // 关闭连接 delay(1); client.stop(); }
In the above code, we first define the name and password of the WiFi network, and then Connect to a WiFi network. We use the WiFiServer object to start a server and listen on port 80. In the loop() function, we wait for the client to send a request, then read the request and send the response to the client. This is a very simple example that will send a simple HTML page to the client when a request is received.
By burning the above code to the Arduino development board, we can set it up as a remotely controlled embedded system. We can then access the Arduino's IP address through a browser to control it remotely.
Of course, the above code is just a simple way to achieve remote control. In actual applications, we can add more functions and interaction methods according to specific needs. For example, we can use GET and POST requests to receive and send more data, we can use JSON format to pass data, we can use encryption technology to protect the security of data transmission, and so on.
To sum up, using the C programming language, we can easily implement the remote control function of the embedded system. By connecting the WiFi module and writing simple server code, we can achieve the basic functions of remote control. Of course, in practical applications, we need to make more customizations and improvements according to specific needs. I hope this article will be helpful to developers who want to learn about remote control of embedded systems.
Reference link:
- [Arduino official website](https://www.arduino.cc/)
- [ESP32 WiFi library](https:/ /github.com/espressif/arduino-esp32/blob/master/libraries/WiFi/examples/WiFiWebServer/WiFiWebServer.ino)
The above is the detailed content of Using C++ to realize remote control function of embedded system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
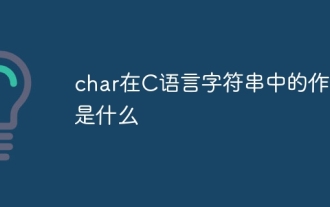
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
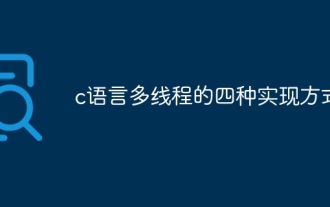
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
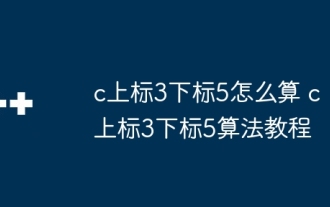
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
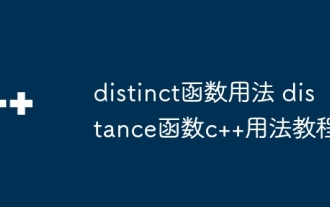
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
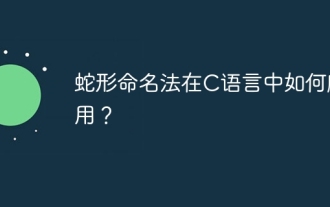
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
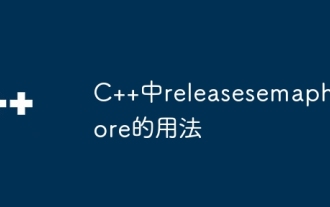
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
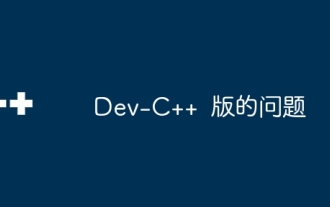
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
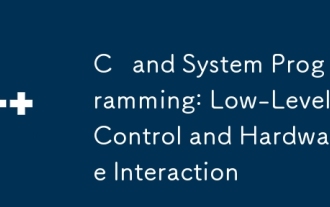
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
