


Use PHP to implement scheduled and delayed message sending of real-time chat function
Use PHP to implement scheduled and delayed message sending of real-time chat function
With the rapid development of the Internet, real-time chat function has been widely used in many websites and applications Applications. This article will introduce how to use PHP language to implement scheduled and delayed message sending in the real-time chat function.
Before implementing the scheduled message sending and delayed sending functions, we first need to build a basic real-time chat system. You can use third-party libraries or frameworks to quickly build a simple chat system, such as using the Laravel framework.
- Building a real-time chat system
In this article, we use the Laravel framework to quickly build a simple real-time chat system. First, we need to install the Laravel framework, which can be installed using the composer command:
composer create-project --prefer-dist laravel/laravel realtime-chat
Next, we create a message model and controller. Enter the following command in the terminal:
php artisan make:model Message -mc
This command will generate a Message model and a MessageController controller.
In the Message model, we need to define the fields and relationships of the message. For example, a message may contain fields such as sender, receiver, and content. Add the following code to the Message model:
class Message extends Model { public function sender() { return $this->belongsTo(User::class, 'sender_id'); } public function receiver() { return $this->belongsTo(User::class, 'receiver_id'); } }
In the MessageController controller, we need to implement the functions of sending messages and obtaining message history. The specific code implementation will be determined according to specific needs and will not be detailed here.
- Implementation of scheduled message sending function
In the real-time chat function, sometimes we need to implement the scheduled message sending function. For example, in a business application, we may need to send a reminder message to a customer at a specified time. Below, we introduce how to use PHP to implement the scheduled sending function of messages. We can use Crontab tasks to implement scheduled sending of messages.
First, we need to create a Crontab task. You can enter the following command through the terminal:
crontab -e
Then, add the following content in the open file:
* * * * * php /path/to/artisan schedule:run >> /dev/null 2>&1
In this example, the Crontab task will be executed once every minute, and the executed command isphp /path/to/artisan schedule:run
.
Next, we need to configure scheduled tasks in Laravel. Open the app/Console/Kernel.php file, find the schedule
method, and then add the configuration of the scheduled task in it. For example, if we want to send a message at 9 o'clock every morning, we can add the following code:
protected function schedule(Schedule $schedule) { $schedule->call(function () { // 获取所有需要发送的消息 $messages = Message::where('scheduled_at', now())->get(); foreach ($messages as $message) { // 发送消息的逻辑 } })->dailyAt('9:00'); }
In this example, the dailyAt('9:00')
method means 9 o'clock every morning Execute a scheduled task.
- Implementation of delayed message sending function
In addition to sending messages regularly, we often need to implement delayed sending function of messages. For example, in a social application, we may need to send a blessing message to the user on their birthday. Below, we introduce how to use PHP to implement the delayed sending function of messages.
In Laravel, we can use queues to implement the delayed sending function of messages. First, we need to configure a message queue. In Laravel, you can use Redis or other queue services to configure a message queue. Here we take Redis as an example to illustrate.
First, we need to install the Redis extension. You can use the following command to install:
pecl install redis
Then, open the configuration file config/database.php
, find the redis
configuration item, in connections
Add the following content to the array:
'redis' => [ 'cluster' => false, 'default' => [ 'host' => env('REDIS_HOST', 'localhost'), 'password' => env('REDIS_PASSWORD', null), 'port' => env('REDIS_PORT', 6379), 'database' => 0, ], ],
Next, we need to create a message queue task. You can run the following command in the terminal:
php artisan make:job SendMessage
This command will generate a new SendMessage
task in the app/Jobs
directory.
In the SendMessage
task, we need to implement the message sending logic. Open the SendMessage
task file and add the following code:
public function handle() { // 获取需要延迟发送的消息 $messages = Message::where('scheduled_at', now())->get(); foreach ($messages as $message) { // 发送消息的逻辑 } }
Next, we need to use a queue in the controller to send messages. Open the MessageController controller file and add the following code in the method of sending the message:
public function sendMessage(Request $request) { // 创建一个新的消息 $message = new Message; $message->content = $request->content; $message->scheduled_at = Carbon::now()->addMinutes($request->delay); $message->save(); // 加入消息队列 SendMessage::dispatch($message); return response()->json(['status' => 'success', 'message' => 'Message sent.']); }
In this example, $request->delay
represents the delay time of the message, which can be determined according to the specific Need to be adjusted.
Through the above code, we realize the scheduled sending and delayed sending functions of messages. Using PHP language and related frameworks and libraries, we can quickly build a stable and reliable real-time chat system and implement various functional requirements to meet the needs of users. At the same time, by using queues and scheduled tasks, we can better manage the sending of messages and provide a better user experience.
The above is the detailed content of Use PHP to implement scheduled and delayed message sending of real-time chat function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


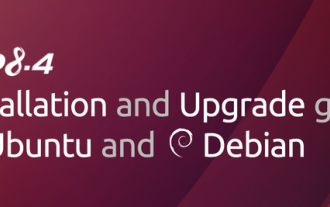
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
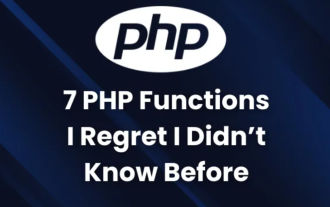
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
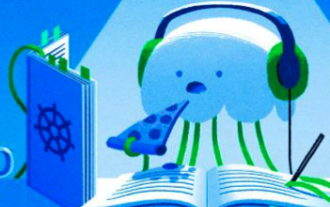
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
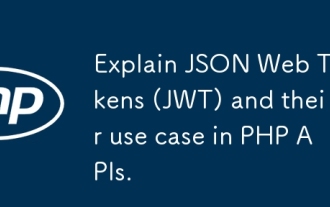
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
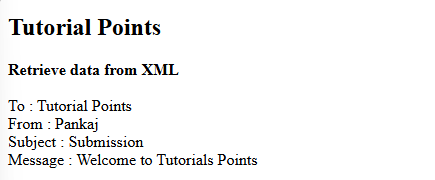
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
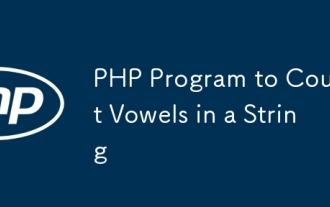
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
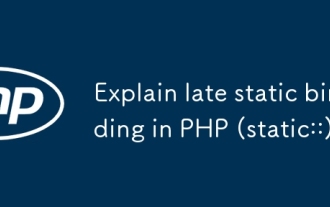
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
