


JavaScript program to find lexicographically smallest string rotation
We will find the lexicographically minimal string rotation in JavaScript. The method involves concatenating the original string with itself and then using the built-in "sort" function to sort the concatenated string in ascending order. Finally, we will return the smallest substring of the sorted concatenated string with the same length as the original string. This will be the smallest string rotation in lexicographic order.
We will implement this logic by using string manipulation techniques and built-in functions available in JavaScript. The result of our implementation will be a string representing the lexicographically minimal rotation of the input string. This is useful for comparing and sorting strings in an efficient way.
In the future, we will continue to improve the algorithm to make it faster and more efficient to find the lexicographically smallest string rotation.
method
Here is explained how to find the lexicographically smallest string rotation in 5 lines -
Concatenate the original string with itself to ensure all possible rotations are considered.
Find the first character that is not equal to the next character, which will be used as the starting point of the minimum rotation.
If no such character is found, the original string is returned as it is already minimally rotated.
Returns the substring in the concatenated string starting from the found character to the end of the string as the minimum rotation.
The resulting substring will be the lexicographically smallest rotation of the string.
Example
The lexicographically smallest rotation of a string can be found by concatenating the original string with itself and finding the smallest substring that starts with the first character of the original string.
This is an example implemented in JavaScript -
function findLexicographicallyMinimumStringRotation(str) { let strDouble = str + str; let len = str.length; let minRotation = strDouble.substring(0, len); for (let i = 1; i < len; i++) { let currRotation = strDouble.substring(i, i + len); if (currRotation < minRotation) { minRotation = currRotation; } } return minRotation; } const str = 'eadbc'; console.log(findLexicographicallyMinimumStringRotation(str));
illustrate
First, we concatenate the original string with itself to get strDouble.
We also define a variable len to store the length of the original string.
Then we initialize minRotation with the first substring of length len in strDouble, that is, strDouble >.Substring(0, len). This is our starting point for finding the lexicographically smallest string rotation.
-
We then use a for loop to iterate over all possible substrings of length len in strDouble starting from the second character.
For each iteration, we find the current rotation currRotation by getting a substring of length len from the strDouble, starting from the current Location i.
If currRotation is less than minRotation, we will update minRotation with the current rotation.
Finally, after the for loop ends, we return the value of minRotation, which is the lexicographically smallest string rotation.
The above is the detailed content of JavaScript program to find lexicographically smallest string rotation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


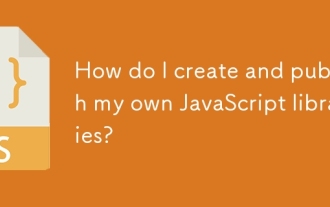
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
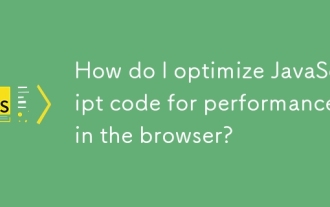
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
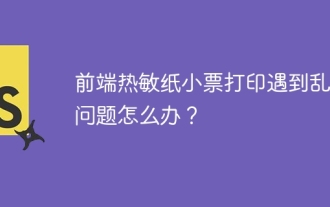
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
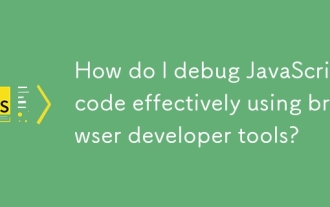
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
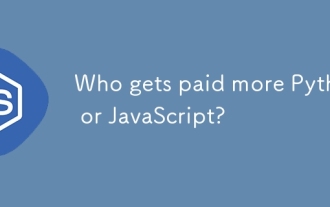
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
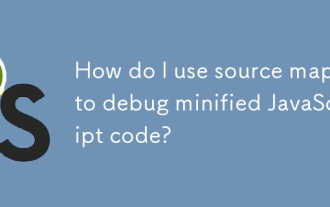
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
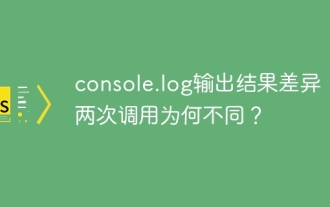
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
