How to deal with data redundancy issues in C++ big data development?
How to deal with the data redundancy problem in C big data development?
Data redundancy refers to storing the same or similar data multiple times during the development process. This results in a waste of data storage space and seriously affects the performance and efficiency of the program. In big data development, the problem of data redundancy is particularly prominent. Therefore, solving the problem of data redundancy is an important task to improve the efficiency of big data development and reduce resource consumption.
This article will introduce how to use C language to deal with data redundancy issues in big data development, and provide corresponding code examples.
1. Use pointers to reduce data copy
When processing big data, data copy operations are often required, which consumes a lot of time and memory. To solve this problem, we can use pointers to reduce data copying. The following is a sample code:
#include <iostream> int main() { int* data = new int[1000000]; // 假设data为一个大数据数组 // 使用指针进行数据操作 int* temp = data; for (int i = 0; i < 1000000; i++) { *temp++ = i; // 数据赋值操作 } // 使用指针访问数据 temp = data; for (int i = 0; i < 1000000; i++) { std::cout << *temp++ << " "; // 数据读取操作 } delete[] data; // 释放内存 return 0; }
In the above code, we use the pointer temp to replace the copy operation, which can reduce the number of data copies and improve the execution efficiency of the code.
2. Use data compression technology to reduce storage space
Data redundancy leads to a waste of storage space. In order to solve this problem, we can use compression technology to reduce data storage space. Commonly used data compression algorithms include Huffman coding, LZW compression algorithm, etc. Following is the sample code for data compression using Huffman coding:
#include <iostream> #include <queue> #include <vector> #include <map> struct Node { int frequency; char data; Node* left; Node* right; Node(int freq, char d) { frequency = freq; data = d; left = nullptr; right = nullptr; } }; struct compare { bool operator()(Node* left, Node* right) { return (left->frequency > right->frequency); } }; void generateCodes(Node* root, std::string code, std::map<char, std::string>& codes) { if (root == nullptr) { return; } if (root->data != '') { codes[root->data] = code; } generateCodes(root->left, code + "0", codes); generateCodes(root->right, code + "1", codes); } std::string huffmanCompression(std::string text) { std::map<char, int> frequencies; for (char c : text) { frequencies[c]++; } std::priority_queue<Node*, std::vector<Node*>, compare> pq; for (auto p : frequencies) { pq.push(new Node(p.second, p.first)); } while (pq.size() > 1) { Node* left = pq.top(); pq.pop(); Node* right = pq.top(); pq.pop(); Node* newNode = new Node(left->frequency + right->frequency, ''); newNode->left = left; newNode->right = right; pq.push(newNode); } std::map<char, std::string> codes; generateCodes(pq.top(), "", codes); std::string compressedText = ""; for (char c : text) { compressedText += codes[c]; } return compressedText; } std::string huffmanDecompression(std::string compressedText, std::map<char, std::string>& codes) { Node* root = new Node(0, ''); Node* current = root; std::string decompressedText = ""; for (char c : compressedText) { if (c == '0') { current = current->left; } else { current = current->right; } if (current->data != '') { decompressedText += current->data; current = root; } } delete root; return decompressedText; } int main() { std::string text = "Hello, world!"; std::string compressedText = huffmanCompression(text); std::cout << "Compressed text: " << compressedText << std::endl; std::map<char, std::string> codes; generateCodes(compressedText, "", codes); std::string decompressedText = huffmanDecompression(compressedText, codes); std::cout << "Decompressed text: " << decompressedText << std::endl; return 0; }
In the above code, we are using Huffman coding to compress the text. First count the frequency of each character in the text, and then build a Huffman tree based on the frequency. Then the code of each character is generated, and 0 and 1 are used to represent the code to reduce the storage space occupied. Finally, the text is compressed and decompressed, and the results are output.
Summary:
By using pointers to reduce data copying and data compression technology to reduce storage space, we can effectively solve the data redundancy problem in big data development. In actual development, it is necessary to choose appropriate methods to deal with data redundancy according to specific circumstances to improve program performance and efficiency.
The above is the detailed content of How to deal with data redundancy issues in C++ big data development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


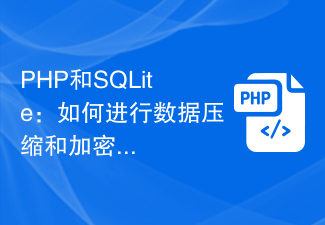
PHP and SQLite: How to Compress and Encrypt Data In many web applications, data security and storage space utilization are very important considerations. PHP and SQLite are two very widely used tools, and this article will introduce how to use them for data compression and encryption. SQLite is a lightweight embedded database engine that does not have a separate server process but interacts directly with applications. PHP is a popular server-side scripting language that is widely used to build dynamic
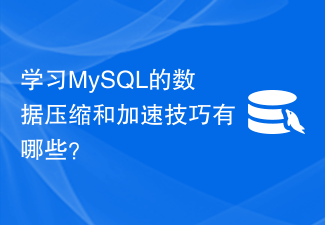
What are the data compression and acceleration techniques for learning MySQL? As a commonly used relational database management system, MySQL is widely used in large-scale data storage and processing. However, as data volume grows and query load increases, database performance optimization becomes an important task. Among them, data compression and acceleration techniques are one of the key factors to improve database performance. This article will introduce some commonly used MySQL data compression and acceleration techniques and provide relevant code examples. Data Compression Tips: Compression Storage Engine
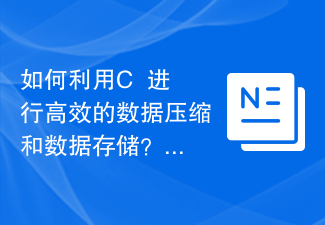
How to use C++ for efficient data compression and data storage? Introduction: As the amount of data increases, data compression and data storage become increasingly important. In C++, there are many ways to achieve efficient data compression and storage. This article will introduce some common data compression algorithms and data storage technologies in C++, and provide corresponding code examples. 1. Data compression algorithm 1.1 Compression algorithm based on Huffman coding Huffman coding is a data compression algorithm based on variable length coding. It does this by pairing characters with higher frequency
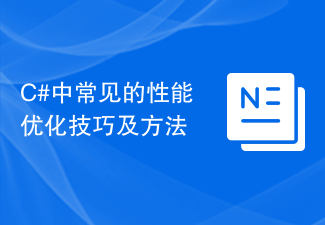
Introduction to common performance optimization techniques and methods in C#: Performance is a very important indicator in software development. Optimizing code to improve system performance is an essential skill for every developer. This article will introduce some common performance optimization techniques and methods in C#, along with specific code examples to help readers better understand and apply them. 1. Avoid frequent object creation and destruction. In C#, object creation and destruction are relatively resource-consuming operations. Therefore, we should try to avoid creating and destroying objects frequently. Here are some common optimization methods:
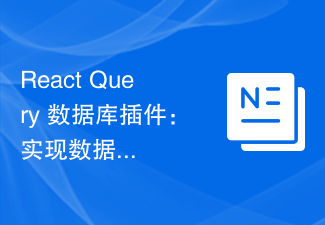
ReactQuery is a powerful data management library that provides many functions and features for working with data. When using ReactQuery for data management, we often encounter scenarios that require data deduplication and denoising. In order to solve these problems, we can use the ReactQuery database plug-in to achieve data deduplication and denoising functions in a specific way. In ReactQuery, you can use database plug-ins to easily process data
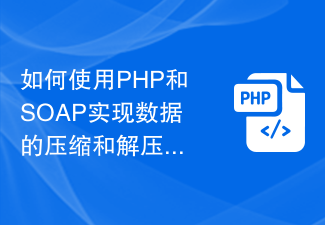
How to use PHP and SOAP to compress and decompress data Introduction: In modern Internet applications, data transmission is a very common operation. However, with the continuous development of Internet applications, the increase in data volume and the requirements for transmission speed, reasonable The use of data compression and decompression techniques has become a very important topic. In PHP development, we can use the SOAP (SimpleObjectAccessProtocol) protocol to achieve data compression and decompression. This article will show you how to
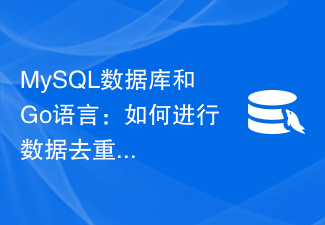
MySQL database and Go language: How to deduplicate data? In actual development work, it is often necessary to deduplicate data to ensure the uniqueness and correctness of the data. This article will introduce how to use MySQL database and Go language to deduplicate data, and provide corresponding sample code. 1. Use MySQL database for data deduplication. MySQL database is a popular relational database management system and has good support for data deduplication. The following introduces two ways to use MySQL database to perform data processing.
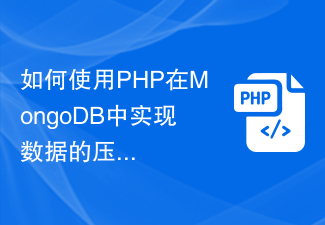
How to use PHP to implement data compression and decompression in MongoDB When processing large amounts of data, data compression and decompression is very important. In MongoDB, we can use some functions provided by PHP to achieve this function. This article will introduce how to use PHP and MongoDB to compress and decompress data, and provide corresponding code examples. 1. Install the extension First, we need to install the MongoDB extension for PHP. This can be done via the following
