Solve C++ compilation error: 'function' does not take 'n' arguments
Solution to C compilation error: 'function' does not take 'n' arguments
In C programming, various compilation errors are often encountered. One of the common errors is: "'function' does not take 'n' arguments", which means that the function does not take n arguments.
This error generally occurs when a function is called. The actual parameters passed in are inconsistent with the number of parameters required when the function is declared, or the types do not match. There are several ways to resolve this error.
- Check the number and type of parameters of the function call
First, we need to check whether the number and type of parameters of the function call are consistent with the function declaration. If the function declaration requires n parameters, then n parameters must be passed in when calling the function, and the parameter types must be consistent with the declaration. For example, in the following sample code, the function add() requires two parameters of type int when it is declared, but only one parameter is passed in when it is called.
#include <iostream> int add(int a, int b) { return a + b; } int main() { int result = add(1); std::cout << result << std::endl; return 0; }
When compiling this code, an error occurs: "'add' does not take 1 arguments". The way to solve this error is to pass in two int type parameters when calling the add() function.
int result = add(1, 2);
- Check whether the function declaration and definition are consistent
If the function declaration and definition are inconsistent, it will also cause a compilation error. The declaration of a function is usually placed in a header file, while the definition of a function is usually placed in a source file. If the function declared in the header file is inconsistent with the number or type of function parameters defined in the source file, a compilation error will occur.
The way to solve this problem is to ensure that the declaration and definition of the function are consistent. For example, in the following sample code, the declaration and definition of the function add() are inconsistent with the number of parameters. It requires two parameters of type int when declaring it, but there is only one parameter when it is defined.
// 头文件 add.h int add(int a, int b); // 源文件 add.cpp int add(int a) { return a + 2; } // 主程序 #include <iostream> #include "add.h" int main() { int result = add(1, 2); std::cout << result << std::endl; return 0; }
When compiling this code, an error occurs: "'add' does not take 2 arguments". The way to solve this error is to pass in two int type parameters when the function is defined, making it consistent with the declaration.
// 源文件 add.cpp int add(int a, int b) { return a + b; }
- Use function overloading
In C, you can use function overloading to solve the problem of inconsistent number of parameters. Function overloading refers to defining multiple functions with the same name in the same scope, but with different number or types of parameters.
For example, we can overload the function add() so that it can accept either two parameters or three parameters. The following is a sample code:
#include <iostream> int add(int a, int b) { return a + b; } int add(int a, int b, int c) { return a + b + c; } int main() { int result1 = add(1, 2); std::cout << result1 << std::endl; int result2 = add(1, 2, 3); std::cout << result2 << std::endl; return 0; }
Using function overloading can flexibly adapt to function calls with different numbers of parameters and avoid compilation errors with inconsistent number of parameters.
Various compilation errors are often encountered in programming. For the error "'function' does not take 'n' arguments", we can ensure that the function is declared and defined by checking the number and type of parameters in the function call. Consistent, and use methods such as function overloading to solve it. Timely error handling and debugging can improve programming efficiency and help us write more robust and reliable code.
The above is the detailed content of Solve C++ compilation error: 'function' does not take 'n' arguments. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The mouse is one of the most important computer peripherals. However, during use, the mouse wheel will inevitably fail or jump up and down, which greatly affects the user's operation. Is there any way to solve this problem? Let’s take a look at three ways to solve the problem of random jumping of the mouse wheel. Method 1: Check the mouse. Mouse produced by different brands have their own characteristics. Some mouse wheels have high sensitivity or low damping, which may cause the wheel to jump erratically. To determine if the problem is with the mouse itself, you can lightly touch the mouse wheel and watch the page move on the screen. If you find that your mouse scrolls too sensitively, you may want to consider buying a new mouse to fix the problem. Method 2: Check the mouse settings Improper mouse settings may cause the mouse wheel to jump randomly.
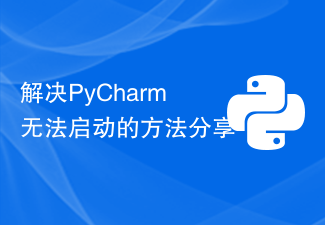
PyCharm is a powerful Python integrated development environment (IDE) that is widely used in the daily work of Python developers. However, sometimes we may encounter the problem that PyCharm cannot be opened normally, which may affect the progress of our work. This article will introduce some common problems and solutions when PyCharm cannot be opened, and provide specific code examples, hoping to help you solve these problems smoothly. Problem 1: PyCharm crashes or becomes unresponsive. Possible reasons: PyCh
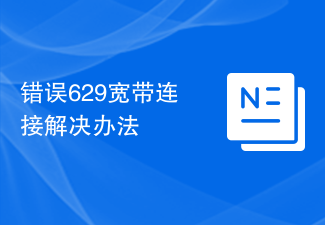
Solution to Error 629 Broadband Connection With the rapid development of Internet technology, broadband has become an indispensable part of our daily lives. However, sometimes we may encounter some problems while using broadband, such as Error 629 Broadband Connection Error. This error usually causes us to be unable to access the Internet normally and brings a lot of inconvenience. In this article, we will share some methods to solve Error 629 Broadband Connection to help you solve this problem quickly. First, Error 629 broadband connection issues are usually caused by network configuration or driver issues
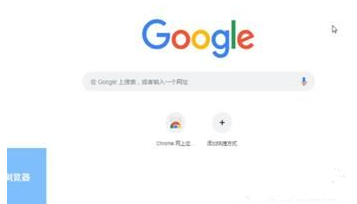
When users use the Edge browser, they may add some plug-ins to meet more of their needs. But when adding a plug-in, it shows that this plug-in is not supported. How to solve this problem? Today, the editor will share with you three solutions. Come and try it. Method 1: Try using another browser. Method 2: The Flash Player on the browser may be out of date or missing, causing the plug-in to be unsupported. You can download the latest version from the official website. Method 3: Press the "Ctrl+Shift+Delete" keys at the same time. Click "Clear Data" and reopen the browser.
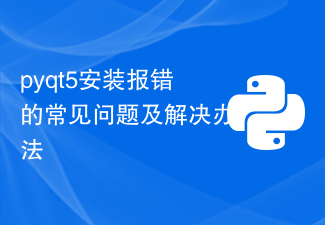
With the popularity of Python, PyQt5 has become one of the first choice tools for many people for rapid GUI development. However, installation problems are inevitable. The following are several common problems with PyQt5 installation and their solutions. The error message when installing PyQt5 is that the sip module cannot be found. This problem usually occurs when using pip to install PyQt5. The reason for this error is the lack of dependencies on the sip module, so you need to install the sip module manually first. Enter the following code on the command line: pipinsta
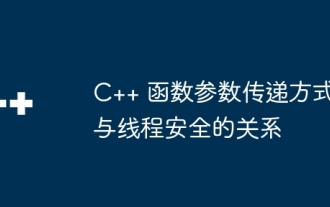
Function parameter passing methods and thread safety: Value passing: Create a copy of the parameter without affecting the original value, which is usually thread safe. Pass by reference: Passing the address, allowing modification of the original value, usually not thread-safe. Pointer passing: Passing a pointer to an address is similar to passing by reference and is usually not thread-safe. In multi-threaded programs, reference and pointer passing should be used with caution, and measures should be taken to prevent data races.

Washing machines play an important role in our daily lives. However, occasionally the buttons of the washing machine are found to be malfunctioning. This situation may bother us because it affects our normal washing operations. However, don’t worry, this article will introduce you to some methods to solve the problem of malfunctioning buttons on your washing machine. 1. Check the power connection - the plug is plugged in properly: Make sure the power plug of the washing machine is firmly inserted into the socket. -Whether the power switch is on: Check whether the power switch is on. 2. Clean the surface of the buttons - shut down: first turn off the washing machine and unplug the power plug to ensure safety. Washing Machine Button Failure Troubleshooting Warm Water Cleaning Use warm water and a neutral detergent to gently wipe the surface of the button. Button adjustment and restart adjustment button position: check whether the button is
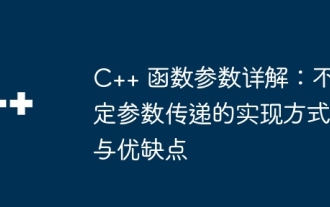
C++ indefinite parameter passing: implemented through the... operator, which accepts any number of additional parameters. The advantages include flexibility, scalability, and simplified code. The disadvantages include performance overhead, debugging difficulties, and type safety. Common practical examples include printf() and std::cout, which use va_list to handle a variable number of parameters.
