How to implement robot control and robot navigation in C++?
How to implement robot control and robot navigation in C?
Robot control and navigation are a very important part of robotics technology. In the C programming language, we can use various libraries and frameworks to implement robot control and navigation. This article will introduce how to use C to write code examples for controlling robots and implementing navigation functions.
1. Robot control
In C, we can use serial communication or network communication to control the robot. The following is a sample code that uses serial port communication to control robot movement:
include
include
include
int main() {
std::string portName = "/dev/ttyUSB0"; // 串口设备名称 SerialPort serialPort(portName); if (!serialPort.isOpen()) { std::cerr << "Failed to open serial port." << std::endl; return -1; } std::cout << "Serial port is open." << std::endl; // 发送控制指令 std::string command = "FWD"; // 向前运动指令 serialPort.write(command); // 接收机器人状态 std::string status = serialPort.read(); std::cout << "Robot status: " << status << std::endl; serialPort.close(); return 0;
}
In the above code, we first create an instance of the SerialPort class and specify the name of the serial port device to be used. Then, we use the isOpen() function to check whether the serial port is opened successfully. If it is opened successfully, we can use the write() function to send control instructions to the robot and the read() function to receive status information from the robot. Finally, we use the close() function to close the serial port.
2. Robot Navigation
Implementing robot navigation usually requires the help of some navigation algorithms and sensor data. The following is a code example that uses the A* algorithm to implement robot path planning:
include
include
include
struct Node {
int x, y; // 节点坐标 int f, g, h; // f值、g值、h值 Node* parent; // 父节点指针 Node(int x, int y) : x(x), y(y), f(0), g(0), h(0), parent(nullptr) {} bool operator<(const Node& other) const { return f > other.f; // 优先级队列按f值从小到大排序 }
};
std::vector
std::vector<Node> path; std::priority_queue<Node> openList; std::vector<Node> closedList(map.size(), std::vector<Node>(map[0].size())); openList.push(start); while (!openList.empty()) { Node current = openList.top(); openList.pop(); closedList[current.x][current.y] = current; if (current.x == end.x && current.y == end.y) { // 找到目标节点 Node* node = &closedList[current.x][current.y]; while (node != nullptr) { path.push_back(*node); node = node->parent; } std::reverse(path.begin(), path.end()); return path; } // 生成周围节点 for (int dx = -1; dx <= 1; ++dx) { for (int dy = -1; dy <= 1; ++dy) { if (dx == 0 && dy == 0) { continue; } int newX = current.x + dx; int newY = current.y + dy; if (newX >= 0 && newX < map.size() && newY >= 0 && newY < map[0].size() && map[newX][newY] == 0) { Node neighbor(newX, newY); neighbor.g = current.g + 1; neighbor.h = abs(newX - end.x) + abs(newY - end.y); neighbor.f = neighbor.g + neighbor.h; neighbor.parent = &closedList[current.x][current.y]; if (closedList[newX][newY].f == 0 || closedList[newX][newY].f > neighbor.f) { openList.push(neighbor); closedList[newX][newY] = neighbor; } } } } } return path; // 没有找到路径
}
int main() {
std::vector<std::vector<int>> map = { {0, 0, 0, 0, 0}, {0, 1, 1, 1, 0}, {0, 0, 0, 1, 0}, {0, 1, 1, 1, 0}, {0, 0, 0, 0, 0}, }; Node start(0, 0); Node end(4, 4); std::vector<Node> path = findPath(map, start, end); for (const auto& node : path) { std::cout << "(" << node.x << ", " << node.y << ")" << std::endl; } return 0;
}
In the above code, we define a Node structure represents the nodes in the map. Using the A* algorithm, we find a path from the start point to the end point in the map. Among them, the map is represented by a two-dimensional array, 0 represents the path that can be passed, and 1 represents obstacles. The function findPath() will return the path from the starting point to the end point, and save the path in the path vector by traversing the parent node pointer. Finally, we output the coordinates of each node on the path.
Summary:
Through the above sample code, we have learned how to use C to implement the control and navigation functions of the robot. Robot control can be achieved using serial communication or network communication, and the robot is controlled by sending control instructions and receiving robot status information. Robot navigation can realize the robot's navigation function through path planning with the help of various navigation algorithms and sensor data. I hope this article can help readers implement robot control and robot navigation in C.
The above is the detailed content of How to implement robot control and robot navigation in C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


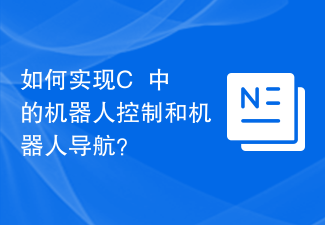
How to implement robot control and robot navigation in C++? Robot control and navigation are very important parts of robotics technology. In the C++ programming language, we can use various libraries and frameworks to implement robot control and navigation. This article will introduce how to use C++ to write code examples for controlling robots and implementing navigation functions. 1. Robot control In C++, we can use serial communication or network communication to realize robot control. The following is a sample code that uses serial communication to control robot movement: inclu
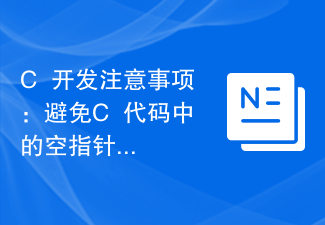
In C++ development, null pointer exception is a common error, which often occurs when the pointer is not initialized or is continued to be used after being released. Null pointer exceptions not only cause program crashes, but may also cause security vulnerabilities, so special attention is required. This article will explain how to avoid null pointer exceptions in C++ code. Initializing pointer variables Pointers in C++ must be initialized before use. If not initialized, the pointer will point to a random memory address, which may cause a Null Pointer Exception. To initialize a pointer, point it to an
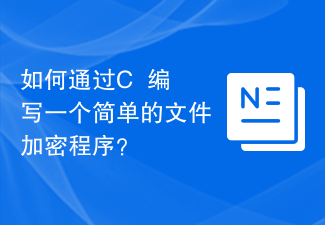
How to write a simple file encryption program in C++? Introduction: With the development of the Internet and the popularity of smart devices, the importance of protecting personal data and sensitive information has become increasingly important. In order to ensure the security of files, it is often necessary to encrypt them. This article will introduce how to use C++ to write a simple file encryption program to protect your files from unauthorized access. Requirements analysis: Before starting to write a file encryption program, we need to clarify the basic functions and requirements of the program. In this simple program we will use symmetry
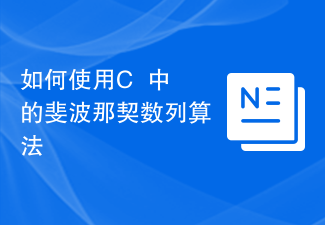
How to use the Fibonacci sequence algorithm in C++ The Fibonacci sequence is a very classic sequence, and its definition is that each number is the sum of the previous two numbers. In computer science, using the C++ programming language to implement the Fibonacci sequence algorithm is a basic and important skill. This article will introduce how to use C++ to write the Fibonacci sequence algorithm and provide specific code examples. 1. Recursive method Recursion is a common method of Fibonacci sequence algorithm. In C++, the Fibonacci sequence algorithm can be implemented concisely using recursion. under
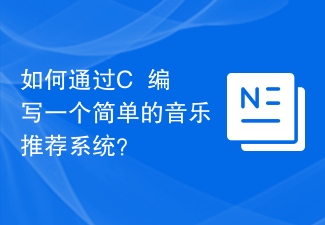
How to write a simple music recommendation system in C++? Introduction: Music recommendation system is a research hotspot in modern information technology. It can recommend songs to users based on their music preferences and behavioral habits. This article will introduce how to use C++ to write a simple music recommendation system. 1. Collect user data First, we need to collect user music preference data. Users' preferences for different types of music can be obtained through online surveys, questionnaires, etc. Save data in a text file or database

How to use Go language to develop and implement robot control and simulation Introduction: With the continuous development of science and technology, robotics technology has begun to be widely used in various fields. In the robot development process, simulation and control are two important links. This article will introduce how to use Go language to develop and implement robot control and simulation, and provide corresponding code examples. 1. Introduction to Go language Go language (or Golang) is an open source programming language developed by Google. It has the characteristics of efficiency, simplicity and strong concurrency.
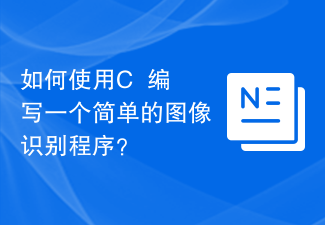
How to write a simple image recognition program using C++? In the development of modern science and technology, image recognition technology plays an increasingly important role. Whether it is face recognition, object detection or autonomous driving, image recognition plays a key role. This article will introduce how to use C++ to write a simple image recognition program to help readers understand the basic principles and implementation process of image recognition. First, we need to install and configure OpenCV (open source computer vision library). OpenCV is a widely used computer vision library for
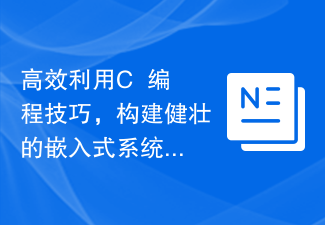
Efficiently utilize C++ programming skills to build robust embedded system functions. With the continuous development of technology, embedded systems play an increasingly important role in our lives. As a high-level programming language, C++ is flexible and scalable and is widely used in embedded system development. In this article, we will introduce some C++ programming techniques to help developers efficiently use C++ to build robust embedded system functions. 1. Use object-oriented design Object-oriented design is one of the core features of the C++ language. In the embedded system
