


Selection and application of various functional modules of C++ in embedded system development
C Selection and application of various functional modules in embedded system development
With the continuous advancement of technology, embedded systems have been widely used in various fields. Including personal electronics, industrial automation, automobiles, etc. As an object-oriented programming language, C has also been widely used in embedded system development. This article will introduce the selection and application of various functional modules of C in embedded system development, and attach corresponding code examples.
- Hardware access module
The core of an embedded system is to interact with hardware, so the hardware access module is an important part of the development of embedded systems. In C, hardware can be accessed by using an underlying hardware abstraction layer library. For example, you can use the Arduino library to access various hardware interfaces of the Arduino development board, such as GPIO, analog input and output, etc. Here is a sample code that uses the Arduino library to access GPIO:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
- Communication Module
Embedded systems often need to communicate with external devices or other systems. C provides a variety of communication modules, such as serial communication, network communication, etc. The following is a sample code using serial port communication:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
- Data storage module
In the development of embedded systems, data needs to be stored and managed. C provides a variety of data storage modules, such as file systems, databases, etc. The following is a sample code that uses the file system for data storage:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
- Control module
Embedded systems usually need to control various devices. C provides a variety of control modules, such as timers, interrupts, etc. The following is a sample code that uses a timer for periodic task control:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
The above is a sample code for the selection and application of some functional modules of C in embedded system development. Of course, specific application scenarios and needs may vary, and we need to adjust and expand based on specific circumstances. By cleverly using C's functional modules, we can develop embedded systems more efficiently and achieve more functions.
The above is the detailed content of Selection and application of various functional modules of C++ in embedded system development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
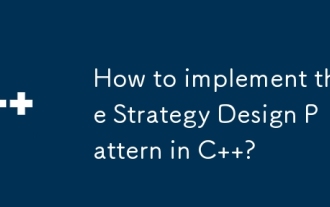
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
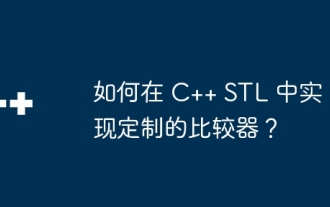
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
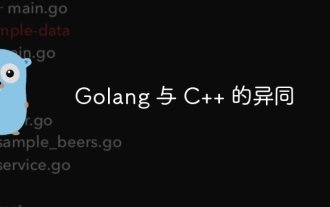
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
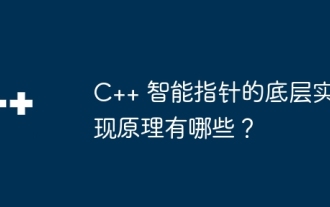
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
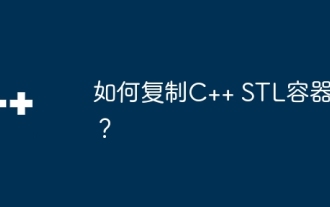
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
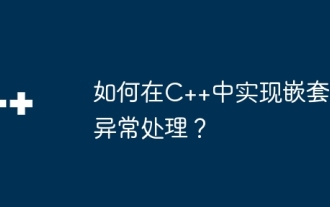
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
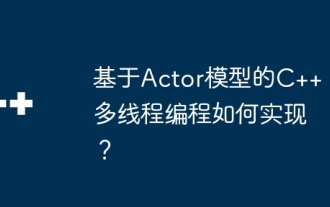
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
