How to compress and format images in Vue?
How to compress and format images in Vue?
In front-end development, we often encounter the need to compress and format images. Especially in mobile development, in order to improve page loading speed and save user traffic, it is critical to compress and format images. In the Vue framework, we can use some tool libraries to compress and format images.
- Use compressor.js library for compression
compressor.js is a JavaScript library for compressing images. It can compress images according to specified configuration items and return the compressed results. We can install compressor.js through npm:
npm install --save compressorjs
Introduce compressor.js into the Vue component:
import Compressor from 'compressorjs';
Then, we can use compressor.js to compress the image. The following is a simple sample code for compressing images:
export default { methods: { compressImage(file) { new Compressor(file, { quality: 0.6, // 压缩质量,取值范围为0到1 success(result) { // 压缩成功后的回调函数 console.log('压缩成功:', result); }, error(err) { // 压缩失败后的回调函数 console.error('压缩失败:', err); }, }); }, }, };
In the above code, we created a Compressor object through the new keyword and specified the compression quality as 0.6. When the compression is successful, the success callback function is called; when the compression fails, the error callback function is called.
- Use exif-js library for image rotation
Photos taken on the mobile terminal may be rotated due to device orientation issues. In order to keep the image in the correct orientation, we can use the exif-js library to read the Exif information of the image and rotate the image based on the Exif information.
We can install exif-js through npm:
npm install --save exif-js
Introduce exif-js into the Vue component:
import EXIF from 'exif-js';
Then, we can use the exif-js library to read Get the Exif information of the picture and rotate it based on the Exif information. The following is a simple sample code for image rotation:
export default { methods: { rotateImage(file) { EXIF.getData(file, function() { const orientation = EXIF.getTag(this, 'Orientation'); const canvas = document.createElement('canvas'); const ctx = canvas.getContext('2d'); const img = new Image(); img.src = URL.createObjectURL(file); img.onload = function() { const degree = getDegreeByOrientation(orientation); canvas.width = img.width; canvas.height = img.height; ctx.rotate((degree * Math.PI) / 180); ctx.drawImage(img, 0, 0); const rotatedImage = canvas.toDataURL(file.type, 1.0); console.log('旋转后的图片:', rotatedImage); }; }); }, getDegreeByOrientation(orientation) { switch (orientation) { case 3: return 180; case 6: return 90; case 8: return 270; default: return 0; } }, }, };
In the above code, we use the EXIF.getData method to obtain the Exif information of the image, and obtain the Orientation (direction) attribute of the image through the getTag method. Then, calculate the angle that needs to be rotated based on the direction attribute. Then, create a canvas element, use the rotate method of canvas to rotate the image, and finally use the toDataURL method of canvas to convert the rotated image to Base64 format.
Through the above two examples, we can compress and format images in Vue. This can help us improve page loading speed and save user traffic. Of course, according to specific needs, we can also combine other tool libraries to further process images, such as cropping, watermarking, etc.
The above is the detailed content of How to compress and format images in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


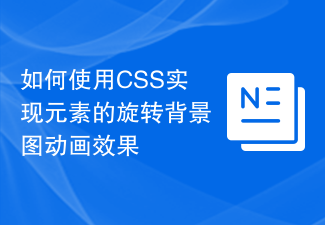
How to use CSS to implement rotating background image animation effects of elements. Background image animation effects can increase the visual appeal and user experience of web pages. This article will introduce how to use CSS to achieve the rotating background animation effect of elements, and provide specific code examples. First, we need to prepare a background image, which can be any picture you like, such as a picture of the sun or an electric fan. Save the image and name it "bg.png". Next, create an HTML file and add a div element in the file, setting it to
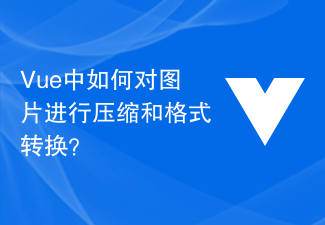
How to compress and format images in Vue? In front-end development, we often encounter the need to compress and format images. Especially in mobile development, in order to improve page loading speed and save user traffic, it is critical to compress and format images. In the Vue framework, we can use some tool libraries to compress and format images. Compression using the compressor.js library compressor.js is a JavaS for compressing images
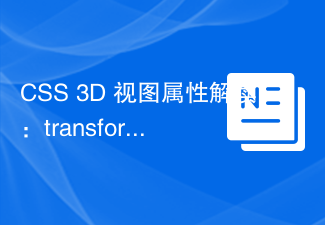
Interpretation of CSS3D view properties: transform and perspective, specific code examples are required Introduction: In modern web design, 3D effects have become a very popular element. Through the transform and perspective properties of CSS, we can easily add 3D visual effects to web pages to make them more vivid and attractive. This article will explain these two properties and provide specific code examples. 1. transform attribute: transf
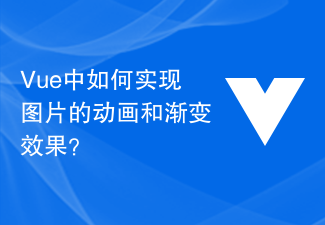
How to implement image animation and gradient effects in Vue? Vue is a progressive framework for building user interfaces that makes it easy to implement animations and gradient effects. In this article, we will introduce how to use Vue to implement image animation and gradient effects, and provide some code examples. 1. Use Vue’s transition effects to implement image animation. Vue provides built-in instructions for transition effects, making it easy to add animation effects to HTML elements. When using transition effects, you can wrap picture elements and add transition instructions on the elements. Example
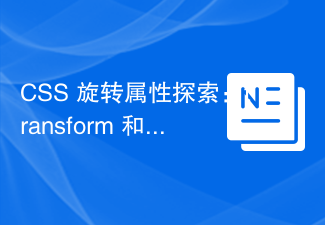
Exploration of CSS rotation properties: transform and rotate Introduction: In modern web design, we often need to add some special effects to elements to increase the attractiveness and user experience of the page. Among them, the rotation of elements is a common effect that can help us create unique visual effects. In CSS, we can use the transform attribute and its rotation attribute rotate to achieve the rotation of the element. This article explores the use of these two properties and provides code
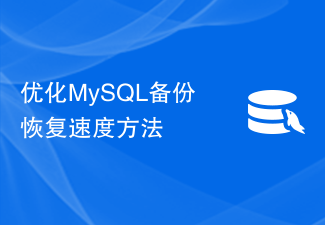
MySQL connection problem: How to optimize the backup and recovery speed of the database? In the process of using MySQL database, backup and recovery are very important operations. They can ensure the security of data and support the stable operation of the system. However, in large or highly loaded database systems, the speed of backup and recovery may become a challenge because they involve a large number of data interactions and network connections. This article will discuss how to optimize the backup and recovery speed of MySQL database. First, choosing an appropriate backup and recovery strategy is
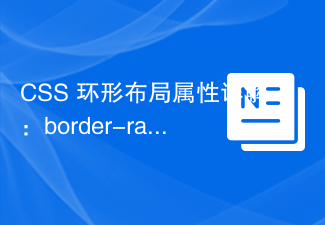
Detailed explanation of CSS circular layout properties: border-radius and transform 1. Introduction In web design, circular layout is often used to create circular elements, such as buttons, avatars, etc. The two key CSS properties for implementing a circular layout are border-radius and transform. This article will introduce in detail how to use the border-radius and transform properties to create a ring layout, and provide specific code examples. 2. border-ra

Resize, crop, rotate, and flip images. First, our original images are 10 images of different sizes downloaded from the Internet, as follows: Operation 1: resize Resize the image to the same size (320,240) fromPILimportImageimporttorchvision.transformsastransforms# Use the PIL library to read in Image and resizeefResizeImage():ifnotos.path.exists(rdir):os.makedirs(rdir)foriinrange(10):im=Image.open(d
