


How to implement statistical charts with real-time data updates through PHP and Vue.js
How to implement statistical charts with real-time data updates through PHP and Vue.js
Introduction:
In modern web applications, data visualization is a very important part. Through statistical charts, we can understand the trends and patterns of data more clearly. Moreover, if these statistical charts can be updated in real time, real-time data analysis and decision support can be provided. This article will introduce how to use PHP and Vue.js to implement statistical charts with real-time data updates.
Technical preparation:
- PHP 5.4 or higher
- Vue.js 2.0 or higher
- Chart.js 2.0 or higher High version
Step 1: Set the basic HTML structure
First, we need to set the basic HTML structure. In this example, we will create a simple bar chart.
<!DOCTYPE html> <html> <head> <title>实时数据统计图表</title> <!-- 引入Chart.js库 --> <script src="https://cdn.jsdelivr.net/npm/chart.js"></script> </head> <body> <div id="app"> <canvas id="chart"></canvas> </div> <!-- 引入Vue.js库 --> <script src="https://cdn.jsdelivr.net/npm/vue"></script> <!-- 引入自定义的脚本 --> <script src="app.js"></script> </body> </html>
Step 2: Write PHP back-end code
Next, we need to write PHP back-end code to obtain real-time data. In this example, we will use a simple random number to simulate real-time data.
<?php // 随机生成一个实时数据 $data = rand(1, 10); // 将数据返回给前端 echo $data;
Step 3: Write Vue.js code
Then, we need to write Vue.js code to handle the acquisition and update of data. In this example, we will use Vue.js components and lifecycle hook functions to achieve this.
First, create a Vue instance in the app.js file and define the required data and methods.
// 创建Vue实例 new Vue({ el: '#app', data: { chart: null, // 图表对象 data: [], // 数据数组 labels: [], // 标签数组 }, methods: { // 初始化图表 initChart() { const ctx = document.getElementById('chart').getContext('2d'); this.chart = new Chart(ctx, { type: 'bar', data: { labels: this.labels, datasets: [{ label: '实时数据', data: this.data, backgroundColor: '#36a2eb', }] }, options: { scales: { y: { beginAtZero: true } } } }); }, // 获取实时数据 fetchData() { axios.get('data.php').then(response => { const data = response.data; this.data.push(data); // 将数据加入到数组中 this.labels.push(new Date().toLocaleTimeString()); // 将时间标签加入到数组中 this.chart.update(); // 更新图表 }).catch(error => { console.error(error); }); }, }, mounted() { this.initChart(); // 初始化图表 this.fetchData(); // 获取实时数据 setInterval(this.fetchData, 2000); // 每两秒钟获取一次实时数据 } });
Step 4: Run the code
Finally, we need to save the above code as an app.js file and run the PHP built-in web server.
Execute the following command in the terminal:
php -S localhost:8000
Then, open http://localhost:8000 in the browser to see the real-time updated histogram.
Conclusion:
Through the combination of PHP and Vue.js, we can easily implement statistical charts with real-time data updates. By constantly getting the latest data and updating charts, we can monitor and analyze data changes in real time. This is very helpful for data-driven decision-making and business process optimization.
I hope this article will help you understand how to implement statistical charts with real-time data updates through PHP and Vue.js!
The above is the detailed content of How to implement statistical charts with real-time data updates through PHP and Vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


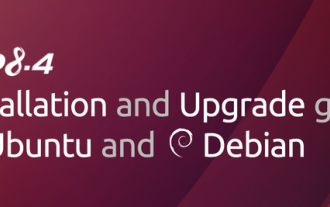
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
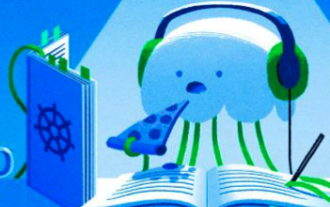
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
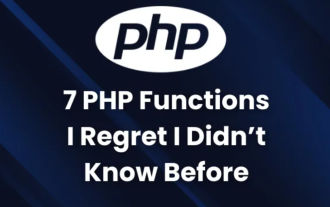
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
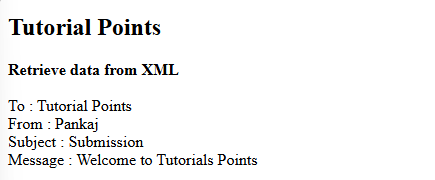
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
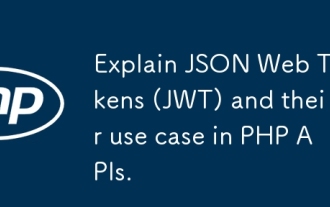
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
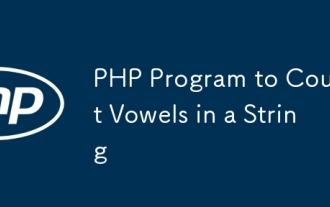
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
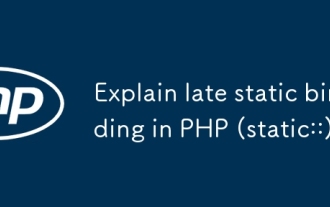
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
