How to use C++ for efficient recommendation system development?
How to use C for efficient recommendation system development?
Introduction:
The recommendation system has become an indispensable part of today's Internet industry. It can recommend personalized content to users by analyzing the user's historical behavior and preferences. As an efficient, flexible and cross-platform programming language, C is widely used in the development of recommendation systems. This article will introduce how to use C for efficient recommendation system development.
1. Data preprocessing
Before developing a recommendation system, data preprocessing needs to be performed first. This includes operations such as data cleaning, denoising, and deduplication. In C, these operations can be implemented using the data structures and algorithms provided by the standard library. The following is a simple data cleaning example code:
#include <iostream> #include <vector> #include <algorithm> // 数据清洗函数 void cleanData(std::vector<int>& data) { // 去重复 std::sort(data.begin(), data.end()); auto it = std::unique(data.begin(), data.end()); data.erase(it, data.end()); // 去零 data.erase(std::remove(data.begin(), data.end(), 0), data.end()); } int main() { std::vector<int> data = {1, 2, 2, 3, 4, 0, 5, 5, 6}; std::cout << "原始数据:"; for (int i : data) { std::cout << i << " "; } std::cout << std::endl; cleanData(data); std::cout << "清洗后数据:"; for (int i : data) { std::cout << i << " "; } std::cout << std::endl; return 0; }
2. Feature extraction and algorithm design
The recommendation system needs to extract useful features from the original data and design an appropriate algorithm for recommendation. In terms of feature extraction, data can be processed using various data structures and algorithms provided by C. For example, you can use a hash table (unordered_map) to count the preferences of different items. The following is a simple feature extraction sample code:
#include <iostream> #include <unordered_map> #include <vector> // 特征提取函数 std::unordered_map<int, int> extractFeatures(const std::vector<int>& data) { std::unordered_map<int, int> features; for (int i : data) { ++features[i]; } return features; } int main() { std::vector<int> data = {1, 2, 2, 3, 4, 2, 3, 5, 6}; std::unordered_map<int, int> features = extractFeatures(data); std::cout << "特征提取结果:" << std::endl; for (const auto& kv : features) { std::cout << "物品:" << kv.first << ",喜好程度:" << kv.second << std::endl; } return 0; }
In terms of algorithm design, the object-oriented features of C can be used to encapsulate the algorithm. For example, you can define a recommendation algorithm class based on collaborative filtering and then use this class to make recommendations. The following is a simple sample code for a recommendation algorithm:
#include <iostream> #include <unordered_map> #include <vector> // 推荐算法类 class CollaborativeFiltering { public: CollaborativeFiltering(const std::unordered_map<int, int>& features) : m_features(features) {} std::vector<int> recommendItems(int userId) { std::vector<int> items; for (const auto& kv : m_features) { if (kv.second >= m_threshold) { items.push_back(kv.first); } } return items; } private: std::unordered_map<int, int> m_features; int m_threshold = 2; }; int main() { std::unordered_map<int, int> features = {{1, 2}, {2, 3}, {3, 1}, {4, 2}, {5, 3}}; CollaborativeFiltering cf(features); std::vector<int> recommendedItems = cf.recommendItems(1); std::cout << "推荐结果:" << std::endl; for (int i : recommendedItems) { std::cout << i << " "; } std::cout << std::endl; return 0; }
3. Performance optimization and concurrency processing
In the development process of recommendation systems, performance optimization and concurrency processing are very important. As an efficient programming language, C provides a variety of optimization and concurrency processing mechanisms. For example, multithreading can be used to speed up large-scale data processing. The std::thread library introduced in C 11 facilitates multi-threaded programming. The following is a simple sample code for concurrent processing:
#include <iostream> #include <vector> #include <thread> // 并发处理函数 void process(std::vector<int>& data, int startIndex, int endIndex) { for (int i = startIndex; i < endIndex; ++i) { data[i] = data[i] * 2; } } int main() { std::vector<int> data(10000, 1); std::vector<std::thread> threads; int numThreads = 4; // 线程数 int chunkSize = data.size() / numThreads; for (int i = 0; i < numThreads; ++i) { int startIndex = i * chunkSize; int endIndex = i == numThreads - 1 ? data.size() : (i + 1) * chunkSize; threads.emplace_back(process, std::ref(data), startIndex, endIndex); } for (auto& thread : threads) { thread.join(); } std::cout << "处理结果:"; for (int i : data) { std::cout << i << " "; } std::cout << std::endl; return 0; }
Conclusion:
This article introduces how to use C for efficient recommendation system development. Through steps such as data preprocessing, feature extraction and algorithm design, performance optimization and concurrent processing, an efficient and accurate recommendation system can be effectively developed. I hope it will be helpful to readers in the development of recommendation systems.
The above is the detailed content of How to use C++ for efficient recommendation system development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
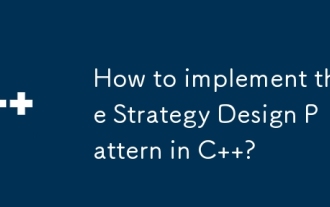
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
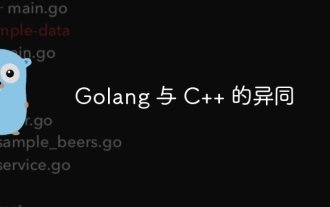
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
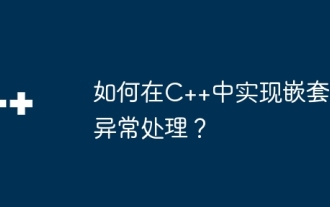
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
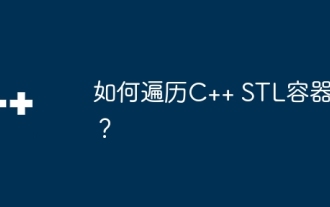
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
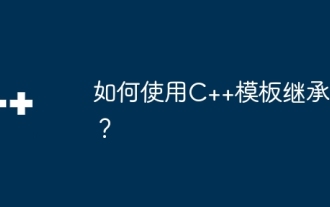
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
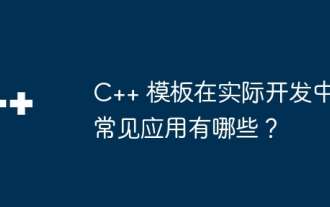
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
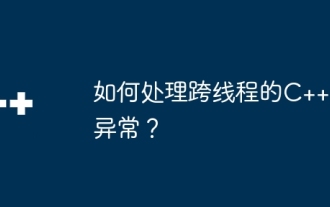
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
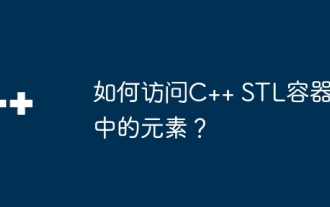
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
