Maximum number of distinct straight lines through a point in C
We get the number N for each line and the coordinates of the two points (x1,y1) and (x2,y2). The goal is to find the maximum number of straight lines from the given straight lines that can pass through a single point such that no two straight lines cover each other and no rotation is performed.
We will represent the straight line as (pair) m,c) where y=mx c,m is the slope m=y2-y1/x2-x1
Given c1!=c2, Lines with the same m are parallel. We will calculate different slopes in meters. For a vertical line, if x1=x2, then slope = INT_MAX, otherwise m.
Let us understand through examples.
Input
Line 1 (x1,y1)=(4,10) (x2,y2)=(2,2) Line 2 (x1,y1)=(2,2) (x2,y2)=(1,1)
Output
Maximum lines: 2
Explanation - The number of bus lines is 2. The slopes of the two lines are different.
Input
Line 1 (x1,y1)=(1,5) (x2,y2)=(3,2) Line 2 (x1,y1)=(2,7) (x2,y2)=(2,8)
Output
Maximum lines: 2
Explanation - The number of bus lines is 2. Both have different slopes.
The methods used in the following program are as follows
The integer arrays x1[] and x2[] are used to store the coordinates of points on the line.
Function numLines(int x1[],int y1[], int x2[], int y2[]) is counting the number of lines passing through a single point.
Apply the formula to each point in x1[],y1[],x2[],y2[] to calculate the slope and use k to increment the slope count.
Array s[] stores slope values.
>Return k as the number of rows in the result.
Example
Live Demonstration
#include <stdio.h> int numLines(int n, int x1[], int y1[], int x2[], int y2[]){ double s[10]; int k=0; double slope; for (int i = 0; i < n; ++i) { if (x1[i] == x2[i]) slope = 999; else slope = (y2[i] - y1[i]) * 1.0 / (x2[i] - x1[i]) * 1.0; s[k++]=slope; } return k; } int main(){ int n = 2; int x1[] = { 1, 5 }, y1[] = { 3, 2 }; int x2[] = { 2,7 }, y2[] = { 2, 8 }; printf("Maximum lines: %d", numLines(n, x1, y1, x2, y2)); return 0; }
Output
If we run the above code, it will generate the following output-
Maximum distinct lines passing through a single point : 2
The above is the detailed content of Maximum number of distinct straight lines through a point in C. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


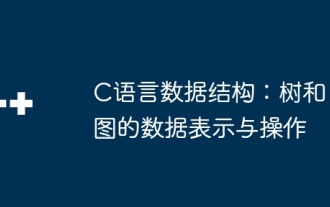
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
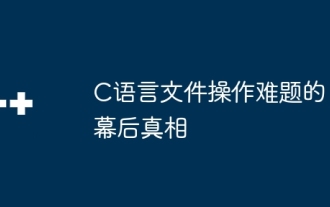
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
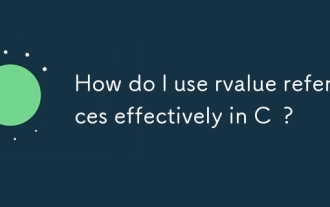
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
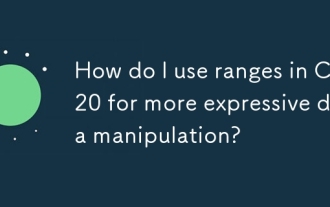
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
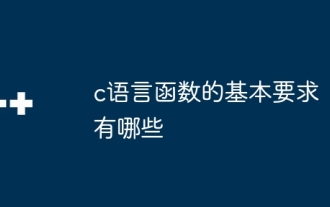
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
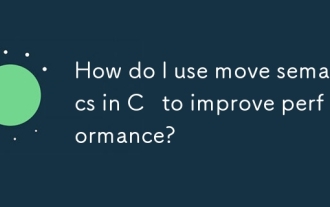
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
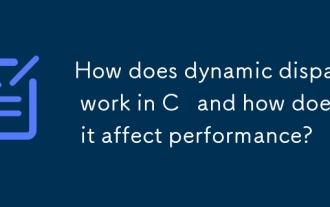
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
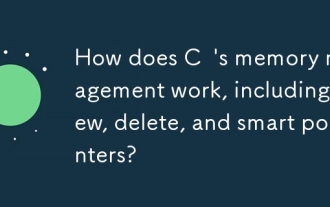
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
