


What are the fastest ways to read a text file line by line using C#?
There are multiple ways to read a text file line by line. These include StreamReader.ReadLine, File.ReadLines, etc. Let's consider the text file that exists within our text file. The local computer has lines like below.
Using StreamReader.ReadLine -
C# StreamReader is used to read characters into the specified stream encoding. StreamReader.Read method reads the next character or next group of characters input stream. StreamReader inherits from TextReader and provides the following methods: Read a character, block, line or everything.
Example
using System; using System.IO; using System.Text; namespace DemoApplication{ public class Program{ static void Main(string[] args){ using (var fileStream = File.OpenRead(@"D:\Demo\Demo.txt")) using (var streamReader = new StreamReader(fileStream, Encoding.UTF8)){ String line; while ((line = streamReader.ReadLine()) != null){ Console.WriteLine(line); } } Console.ReadLine(); } } }
Output
Hi All!! Hello Everyone!! How are you?
Use File.ReadLines
File.ReadAllLines() method to open a text file and read all the lines of the file one
IEnumerable
Example
using System; using System.IO; namespace DemoApplication{ public class Program{ static void Main(string[] args){ var lines = File.ReadLines(@"D:\Demo\Demo.txt"); foreach (var line in lines){ Console.WriteLine(line); } Console.ReadLine(); } } }
Output
Hi All!! Hello Everyone!! How are you?
Using File.ReadAllLines
This is very similar to ReadLines. However, it returns String[] instead of
IEnumerable
Example
using System; using System.IO; namespace DemoApplication{ public class Program{ static void Main(string[] args){ var lines = File.ReadAllLines(@"D:\Demo\Demo.txt"); for (var i = 0; i < lines.Length; i += 1){ var line = lines[i]; Console.WriteLine(line); } Console.ReadLine(); } } }
Output
Hi All!! Hello Everyone!! How are you?
The above is the detailed content of What are the fastest ways to read a text file line by line using C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


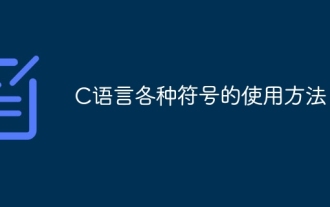
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
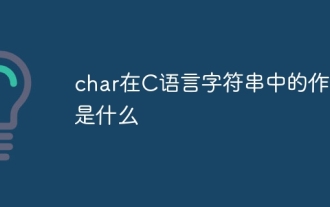
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
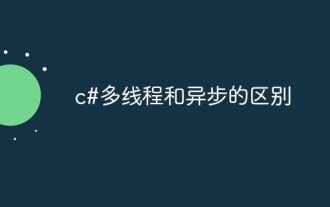
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
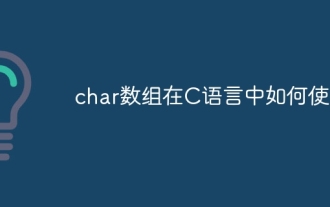
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
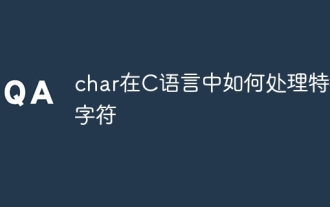
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
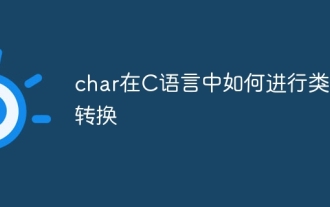
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
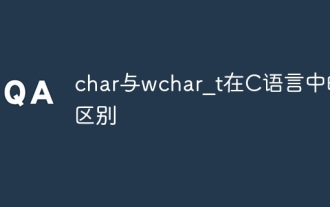
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
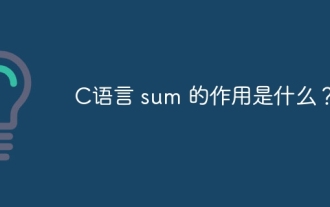
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
