


How to create a simple socket communication program using PHP?
How to create a simple socket communication program using PHP?
With the popularity and development of the Internet, network communication has become an indispensable part of people's daily lives. In the process of network communication, sockets are widely used to implement inter-process communication and network communication. As a popular server-side programming language, PHP can also use the socket function extensions it provides to quickly create simple socket communication programs. This article will introduce how to use PHP to create a simple socket communication program, with corresponding code examples.
In PHP, the basic steps to create a socket communication program are as follows:
Step 1: Create socket
Use the socket_create function to create a socket object, specify the communication protocol, communication type and communication The version of the protocol. For example, creating a socket object based on the TCP protocol can be written as follows:
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
Step 2: Bind the socket to the specified IP address and port
Use the socket_bind function to bind the socket to the specified IP address and port . For example, to bind the socket to the local machine's 127.0.0.1 address and port 8080, you can write:
$address = '127.0.0.1'; $port = 8080; if (!socket_bind($socket, $address, $port)) { die('绑定socket失败'); }
Step 3: Monitor the socket connection request
Use the socket_listen function to monitor the socket connection request and specify the maximum Number of connections. For example, listening for up to 5 connection requests can be written like this:
if (!socket_listen($socket, 5)) { die('监听socket失败'); }
Step 4: Accept the client connection request
Use the socket_accept function to accept the client's connection request and return a new socket object, which uses for communicating with the client. For example, accepting a connection request from a client can be written like this:
$clientSocket = socket_accept($socket); if ($clientSocket === false) { die('接受客户端连接失败'); }
Step 5: Communicate with the client
Use the socket_read and socket_write functions to read and write data with the client. For example, to receive the data sent by the client, you can write like this:
$data = socket_read($clientSocket, 1024); if ($data === false) { die('读取数据失败'); } echo '接收到的数据:' . $data;
Step 6: Close the socket connection
Use the socket_close function to close the socket connection. For example, closing the socket connection with the client can be written as follows:
socket_close($clientSocket);
Using the above steps, we can create a simple socket communication program. The following is a complete sample code:
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP); $address = '127.0.0.1'; $port = 8080; if (!socket_bind($socket, $address, $port)) { die('绑定socket失败'); } if (!socket_listen($socket, 5)) { die('监听socket失败'); } echo '开始监听连接...'; while (true) { $clientSocket = socket_accept($socket); if ($clientSocket === false) { die('接受客户端连接失败'); } $data = socket_read($clientSocket, 1024); if ($data === false) { die('读取数据失败'); } echo '接收到的数据:' . $data; $response = 'Hello, Client!'; socket_write($clientSocket, $response, strlen($response)); socket_close($clientSocket); } socket_close($socket);
The above code implements a simple socket communication program. It listens to the local port 8080 and accepts client connection requests. After receiving the data sent by the client, a reply message is sent to the client. It should be noted that this example does not handle the situation of multiple clients connecting at the same time. It is only for learning and understanding the basic principles of socket communication.
To summarize, using PHP to create a simple socket communication program requires completing the following steps: creating a socket, binding the socket to the specified IP address and port, listening for socket connection requests, accepting client connection requests, and communicating with the client. Communicate and close the socket connection. By understanding and mastering the principles and methods of socket programming, we can better apply socket technology in actual development and provide more possibilities for network communication.
The above is the detailed content of How to create a simple socket communication program using PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


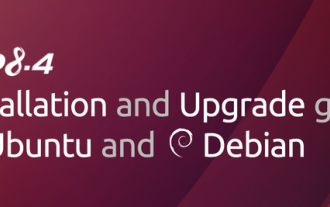
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
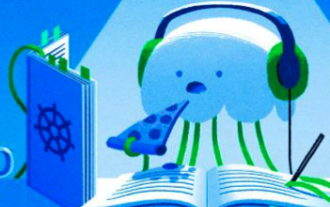
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
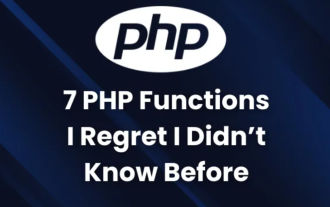
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
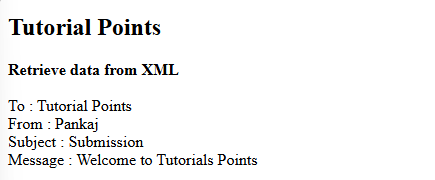
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
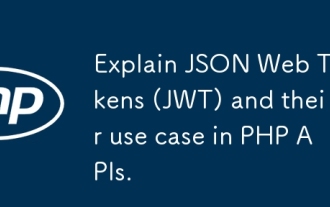
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
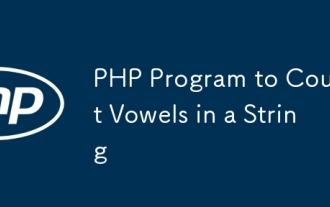
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
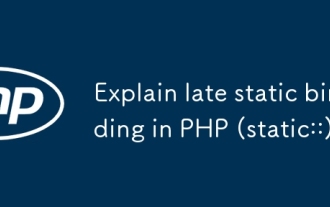
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
