


Practice of data collection and processing functions of C++ in embedded system development
C Practice of data collection and processing functions in embedded system development
Abstract: Embedded system development has relatively high requirements for data collection and processing functions in practical applications. high. Through an example, this article shows how to use C language to implement data acquisition and processing functions in embedded systems. The specific implementation plan and code examples will be introduced in detail below.
- Introduction
With the widespread application of embedded systems in various industries, the demand for data collection and processing is increasing day by day. As an efficient, flexible and object-oriented programming language, C language is widely used in the development of embedded systems. This article will illustrate the advantages and applications of C in data acquisition and processing functions through an example. - Instance Background
Suppose we want to design a temperature and humidity acquisition system to monitor indoor environmental conditions. The system needs to collect temperature and humidity data in real time and process the data. We will implement the data collection and processing functions of this system through C language. - Data Collection
3.1 Sensor Driver
First, we need to write a sensor driver to read the temperature and humidity sensor. The following is a simplified pseudocode example:
#include <sensor.h> class SensorDriver { public: SensorDriver(); ~SensorDriver(); float readTemperature(); float readHumidity(); private: Sensor* sensor; }; SensorDriver::SensorDriver() { sensor = new Sensor(); } SensorDriver::~SensorDriver() { delete sensor; } float SensorDriver::readTemperature() { return sensor->readTemperature(); } float SensorDriver::readHumidity() { return sensor->readHumidity(); }
In the above code, we created a class named SensorDriver, which encapsulates the reading function of the sensor. Real-time temperature and humidity data can be obtained through the readTemperature() and readHumidity() functions.
3.2 Data Storage
Next, we need to store the collected data for subsequent processing. The following is a simplified pseudocode example:
#include <iostream> #include <fstream> class DataStorage { public: DataStorage(); ~DataStorage(); void storeData(float temperature, float humidity); private: std::ofstream file; }; DataStorage::DataStorage() { file.open("data.txt", std::ofstream::app); } DataStorage::~DataStorage() { file.close(); } void DataStorage::storeData(float temperature, float humidity) { file << "Temperature: " << temperature << ", Humidity: " << humidity << std::endl; }
In the above code, we created a class named DataStorage, which is responsible for storing the collected data into files. Use the storeData() function to write real-time temperature and humidity data to the data.txt file.
- Data processing
4.1 Data analysis
Before data processing, we need to analyze the collected data. The following is a simplified pseudocode example:
#include <vector> class DataAnalyzer { public: DataAnalyzer(); ~DataAnalyzer(); void analyzeData(std::vector<float> temperatures, std::vector<float> humidities); private: // 数据分析相关的成员变量和函数 }; DataAnalyzer::DataAnalyzer() { // 初始化成员变量 } DataAnalyzer::~DataAnalyzer() { // 释放资源 } void DataAnalyzer::analyzeData(std::vector<float> temperatures, std::vector<float> humidities) { // 数据分析逻辑 }
In the above code, we created a class called DataAnalyzer, which is responsible for analyzing the collected temperature and humidity data. Through the analyzeData() function, you can get the corresponding analysis results.
- System integration
Finally, we need to integrate the data collection and processing functions. The following is a simplified pseudocode example:
int main() { SensorDriver sensorDriver; DataStorage dataStorage; DataAnalyzer dataAnalyzer; while (true) { // 读取温湿度数据 float temperature = sensorDriver.readTemperature(); float humidity = sensorDriver.readHumidity(); // 存储温湿度数据 dataStorage.storeData(temperature, humidity); // 在一定时间间隔后进行数据分析 // ... // 数据分析 dataAnalyzer.analyzeData(temperatures, humidities); } return 0; }
In the above code, we created instances of SensorDriver, DataStorage and DataAnalyzer in the main() function, and then continuously read the temperature and humidity data through a loop, and perform storage and analysis.
- Conclusion
Through the above examples, we have demonstrated the method of using C language to implement data acquisition and processing functions in embedded systems. The efficiency and flexibility of C language allow us to easily implement functions such as sensor driving, data storage and processing. In practical applications, we can expand and optimize these functions as needed to meet specific embedded system development needs.
Reference:
[1] C Reference. (n.d.). C Reference Home. Retrieved from http://www.cplusplus.com/
The above is the detailed content of Practice of data collection and processing functions of C++ in embedded system development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
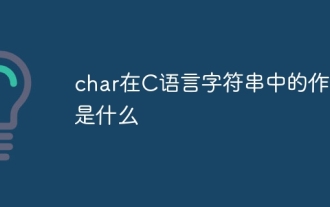
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
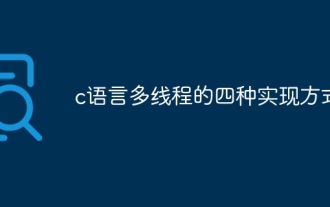
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
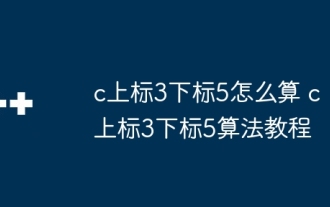
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
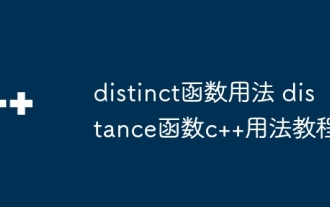
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
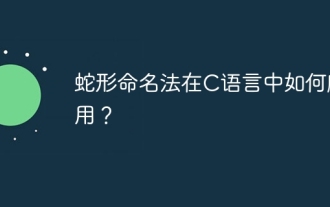
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
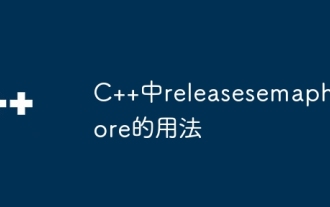
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
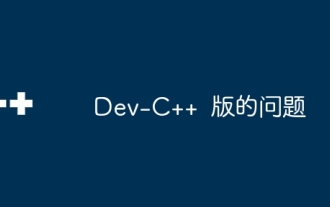
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
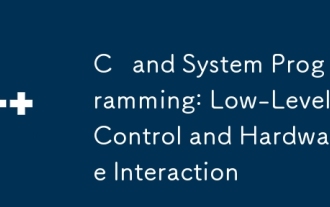
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
