


Brainstorming: Secrets to building efficient brain mapping applications with PHP and Vue
Brainstorming: Secrets to building efficient mind mapping applications with PHP and Vue
Overview:
In modern society, mind mapping applications play an important role in improving efficiency and organizing thinking. plays an important role. This article will introduce how to use PHP and Vue to build efficient mind mapping applications, and provide code examples for readers' reference.
Part One: Backend Development (PHP)
In building the backend part of the mind mapping application, we will choose PHP as the development language. PHP is a commonly used server-side scripting language that is easy to learn and use, and has extensive support and documentation.
Step 1: Create a database
First, we need to create a database to store the brain map data. We can use MySQL or other relational database management systems to create databases and tables.
Sample code:
CREATE DATABASE mindmap; USE mindmap; CREATE TABLE nodes ( id INT AUTO_INCREMENT PRIMARY KEY, parent_id INT, label VARCHAR(255) );
Step 2: Write API interface
Next, we need to write an API interface to handle the requests sent by the front end and interact with the database. We can use PHP frameworks (such as Laravel or Symfony) to simplify this process.
Sample code (using Laravel framework):
// 创建节点 public function createNode(Request $request) { $node = new Node; $node->parent_id = $request->parent_id; $node->label = $request->label; $node->save(); return response()->json(['node' => $node]); } // 获取节点 public function getNode($id) { $node = Node::find($id); return response()->json(['node' => $node]); } // 更新节点 public function updateNode(Request $request, $id) { $node = Node::find($id); $node->parent_id = $request->parent_id; $node->label = $request->label; $node->save(); return response()->json(['node' => $node]); } // 删除节点 public function deleteNode($id) { $node = Node::find($id); $node->delete(); return response()->json(['message' => 'Node deleted successfully']); }
Part 2: Front-end development (Vue)
The front-end part uses the Vue framework to build interactive interfaces and real-time updates.
Step 1: Install Vue
First, we need to install Vue and related dependencies. We can use npm (Node Package Manager) to install these dependencies.
Sample code:
$ npm install vue vue-router axios
Step 2: Build the brain map component
Next, we will use Vue to build the brain map component. This component is responsible for rendering the mind map, handling user operations, and communicating with the backend API.
Sample code:
<template> <div> <div class="mindmap"> <div v-for="node in nodes" :key="node.id" class="node"> {{ node.label }} </div> </div> <button @click="addNode">Add Node</button> </div> </template> <script> import axios from 'axios'; export default { data() { return { nodes: [], }; }, mounted() { this.getNodes(); }, methods: { getNodes() { axios.get('http://localhost/api/nodes') .then((response) => { this.nodes = response.data.nodes; }) .catch((error) => { console.error(error); }); }, addNode() { axios.post('http://localhost/api/nodes', { parent_id: null, label: 'New Node', }) .then((response) => { this.nodes.push(response.data.node); }) .catch((error) => { console.error(error); }); }, }, }; </script>
Part 3: Testing and Deployment
After completing the above steps, we can test and deploy the application to the production environment. We can use testing frameworks such as PHPUnit to test API interfaces, and use common web servers such as Nginx or Apache to deploy applications.
Conclusion:
By using PHP and Vue, we can easily build efficient mind mapping applications. In terms of back-end development, we used PHP to create the database and API interface; in terms of front-end development, we used Vue to build the interactive interface and implemented data interaction with the back-end through the API interface. I hope this article will be helpful to you in building a mind mapping application. I wish you can build an efficient mind mapping application and improve your work efficiency!
The above is the detailed content of Brainstorming: Secrets to building efficient brain mapping applications with PHP and Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


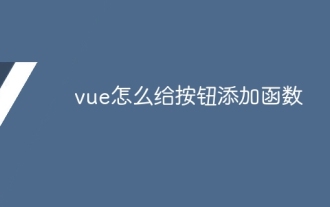
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
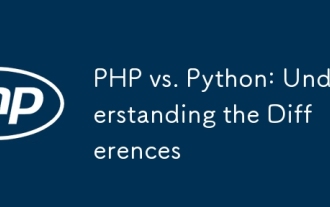
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
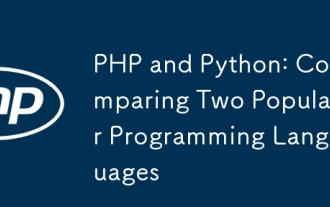
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
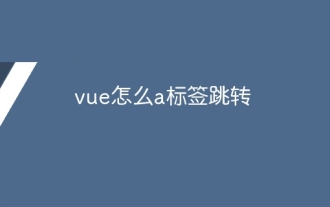
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
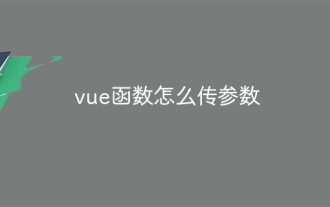
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
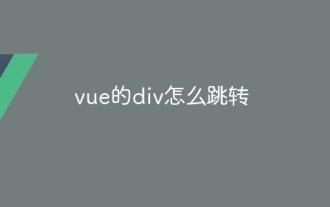
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
