


PHP development of real-time chat functionality with voice messaging and video calling support
PHP development of real-time chat functionality for voice messaging and video calling support
Introduction:
Live chat functionality has become a common requirement in modern applications, and with the With the continuous advancement of technology, voice messages and video calls have become the main ways for users to communicate. This article will introduce how to use PHP to develop a real-time chat function and add support for voice messages and video calls.
1. The basis of real-time chat function
- Client preparation
Before we start, we need to prepare basic client code and use HTML, CSS and JavaScript to build chat interface. Data is exchanged with the server through the WebSocket protocol. - Server-side preparation
For the server-side, we need to use PHP to handle WebSocket requests. Usually, we need to use the PHP WebSocket library to implement WebSocket server functionality. In this article, we will use the Ratchet library as a WebSocket server.
2. Implement the voice message function
In order to realize the voice message function, we need to use WebRTC technology. WebRTC is an open, cross-platform API that provides real-time communication capabilities through the browser without the need for plug-ins or additional installations.
The specific steps are as follows:
- Get the user's recording data
Use HTML5's getUserMedia API to obtain the user's microphone input and transmit the recorded audio data to the server.
navigator.mediaDevices.getUserMedia({ audio: true }) .then(function(stream) { var audioContext = new AudioContext(); var mediaStreamSource = audioContext.createMediaStreamSource(stream); // ... }) .catch(function(error) { console.log('getUserMedia error: ' + error); });
- Sending and receiving voice data
Encode the obtained audio data, and then transmit it to the server through WebSocket, and the server then sends the data to the receiver.
// 发送语音数据 function sendVoiceData(data) { connection.send(data); } // 接收语音数据 connection.onmessage = function(message) { var data = message.data; // 处理接收到的音频数据 };
- Play voice data
The receiver can decode the received voice data and play it.
3. Implement the video call function
To implement the video call function, you also need to use WebRTC technology. WebRTC can obtain and process audio and video streams and transmit them over the network.
The specific steps are as follows:
- Get the user's camera and microphone data
Use the getUserMedia API to obtain the user's camera and microphone data and transmit the data to the server.
navigator.mediaDevices.getUserMedia({ video: true, audio: true }) .then(function(stream) { var videoElement = document.getElementById('local-video'); videoElement.srcObject = stream; // ... }) .catch(function(error) { console.log('getUserMedia error: ' + error); });
- Sending and receiving video data
Encode the obtained video data, and then transmit it to the server through WebSocket, and the server then sends the data to the receiver.
// 发送视频数据 function sendVideoData(data) { connection.send(data); } // 接收视频数据 connection.onmessage = function(message) { var data = message.data; // 处理接收到的视频数据 };
- Play video data
The receiver can decode the received video data and play it.
Conclusion:
The above are the basic steps to implement voice messaging and video call support for PHP development of real-time chat function. By combining WebSocket and WebRTC technologies, we can easily implement these functions. Hope this article helps you!
The above is the detailed content of PHP development of real-time chat functionality with voice messaging and video calling support. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


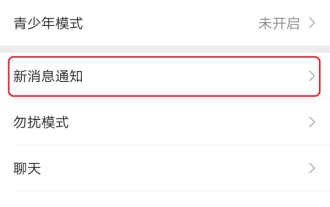
In our daily life and work, using WeChat for simple and important communication has become something that everyone will encounter. At the same time, WeChat has also become an indispensable communication tool in our lives. Recently, some friends using the Android version of WeChat encountered a problem. When you make a WeChat call to a friend, not only can you hear the friend's incoming call ringtone, but the friend's WeChat call ringtone is different from other people's, and is no longer a monotonous and boring unified ringtone. So, how to set the ringtone for voice and video calls on the Android version of WeChat? Download The editor of this website will introduce the specific method to you. I hope it will be helpful to friends who have this need. How to set the ringtone for incoming calls in the WeChat Android version? Open the WeChat interface, find the [Me] option and click to enter, then find the [Settings] option

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
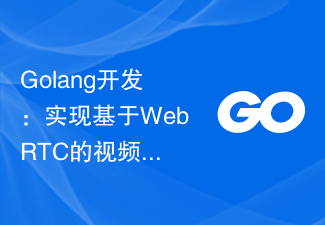
Golang development: Implementing video call applications based on WebRTC Summary: WebRTC (WebReal-Time Communication) is an open standard real-time audio and video communication technology that can be used to build audio and video calls, conferences, real-time live broadcasts and other applications. This article will introduce how to use Golang to develop a video call application based on WebRTC, and provide some specific code examples to help readers have a deeper understanding and mastery of related technologies. 1. Background WebRT
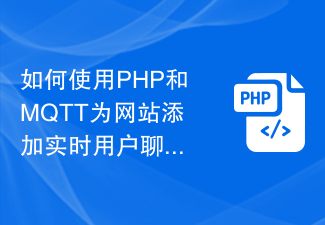
How to use PHP and MQTT to add real-time user chat function to the website. In today's Internet era, website users increasingly need real-time communication and communication. In order to meet this demand, we can use PHP and MQTT to add real-time user chat function to the website. This article will introduce how to use PHP and MQTT to implement the real-time user chat function of the website and provide code examples. Make sure the environment is ready Before starting, make sure you have installed and configured the PHP and MQTT runtime environments. You can use integrated development such as XAMPP
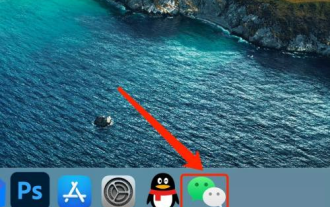
Recently, some friends have consulted the editor about how to set up WeChat Mac to automatically convert voice messages into text. The following is a method for setting up WeChat Mac to automatically convert voice messages into text. Friends in need can come and learn more. Step 1: First, open the Mac version of WeChat. As shown in the picture: Step 2: Next, click "Settings". As shown in the picture: Step 3: Then, click "General". As shown in the picture: Step 4: Then check the option "Automatically convert voice messages in chat to text". As shown in the picture: Step 5: Finally, close the window. As shown in the picture:
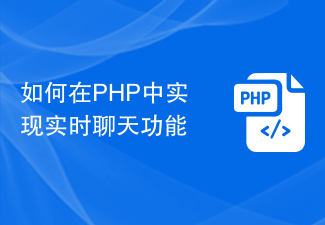
How to implement real-time chat function in PHP With the popularity of social media and instant messaging applications, real-time chat function has become a standard feature of many websites and applications. In this article, we will explore how to implement live chat functionality using PHP language, along with some code examples. Using WebSocket Protocol Live chat functionality typically requires the use of the WebSocket protocol, which allows two-way communication between the server and the client. In PHP, we can use the Ratchet library to implement WebSocket services
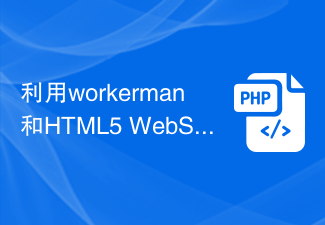
Real-time online chat using Workerman and HTML5 WebSocket technology Introduction: With the rapid development of the Internet and the popularity of smartphones, real-time online chat has become an indispensable part of people's daily lives. In order to meet the needs of users, web developers are constantly looking for more efficient and real-time chat solutions. This article will introduce how to combine the PHP framework Workerman and HTML5 WebSocket technology to implement a simple real-time online chat system.
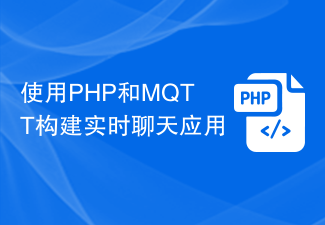
Building a real-time chat application using PHP and MQTT Introduction: With the rapid development of the Internet and the popularity of smart devices, real-time communication has become one of the essential functions in modern society. In order to meet people's communication needs, developing a real-time chat application has become the goal pursued by many developers. In this article, we will introduce how to use PHP and MQTT (MessageQueuingTelemetryTransport) protocol to build a real-time chat application. what is
