How to get hashtable elements as sorted array?
A hash table is a non-universal collection of key-value pairs arranged according to the hash code of the key. Hash tables are used to create collections that are stored using hash tables. Hash tables optimize lookups by calculating the hash code for each key and storing it in an internal basket. When we access a specific value from the hash table, the hash code matches the specified key.
This hash table collection is defined in the System.Collections namespace of C#. The class that represents a collection of hash tables is the "Hashtable" class. This class provides constructors, methods, and properties to operate on collections of hash tables. By default, hash table collections are unsorted. If we want a sorted collection of hash tables, we need to represent it as an Array or ArrayList and sort the elements.
In this article, we will see how to get the hash table elements in the form of a sorted array. So let's get started.
Get hash table elements as sorted array
We know that by default, the hash table collection is unsorted. Sorting a hash table collection can be very difficult because we create the hash table collection based on the keys and then add values to each key.
If we want to sort a collection of hash tables, we must sort it based on keys or values. There is no direct method in the Hashtable class to sort a collection of hash tables. So we must turn to other methods.
One way is to get the hash table elements (keys or values) as a sorted array. To do this, we will follow the steps listed below.
Create a hash table object
Fill this object with key-value pairs
Create an array of string type, length = length of hash table
Traverse the hash table according to the key and fill the array with each key
Sort the generated array
Example
We wrote this method using C# as shown below.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable langCodes = new Hashtable(); // Add elements to the Hashtable langCodes.Add("C++", "CPlusPlus"); langCodes.Add("C#", "CSharp"); langCodes.Add("Java", "Java"); langCodes.Add("PL", "Perl"); langCodes.Add("PG", "Prolog"); int k = langCodes.Count; // create array of length = hashtable length string[] sortedArray = new string[k]; // Retrieve key values in Array int i = 0; Console.WriteLine("Hashtable langCodes Contents:"); foreach (DictionaryEntry de in langCodes) { Console.WriteLine("{0} ({1}) ", de.Key, de.Value); sortedArray[i] = de.Key.ToString(); i++; } Array.Sort(sortedArray); Console.WriteLine("Contents of sorted array based on Hashtable keys:"); foreach (var item in sortedArray) { Console.WriteLine(item); } } }
In this program, we define a Hashtable object langCodes and populate it with key-value pairs. We then retrieve the length of the hash table and use Declare an array "sortedArray" of this length. Next we traverse langCodes hash table and populate the sorted array object with keys langCodes Hash table values.
Then we use the filter Array.Sort(sortedArray) to sort the array and print it This sorted array.
Output
The output of the program is as follows.
Hashtable langCodes Contents: PG (Prolog) Java (Java) C# (CSharp) PL (Perl) C++ (CPlusPlus) Contents of sorted array based on Hashtable keys: C# C++ Java PG PL
From the output, we can see that the hash table key elements are retrieved as a sorted array of elements.
Now let’s give another example. We use the same method discussed above. The only difference is that in this example we will populate the array with values instead of keys from a hash table.
Example
Let’s look at the complete C# program.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable numberNames = new Hashtable(); // Add elements to the Hashtable numberNames.Add(12, "Twelve"); numberNames.Add(2, "Two"); numberNames.Add(65, "Sixty Five"); numberNames.Add(15, "Fifteen"); numberNames.Add(18, "Eighteen"); int k = numberNames.Count; //create array of length = hashtable length string[] sortedArray = new string[k]; // Retrieve hashtable values in array. int i = 0; Console.WriteLine("Hashtable langCodes Contents:"); foreach (DictionaryEntry de in numberNames) { Console.WriteLine("{0} ({1}) ", de.Key, de.Value); sortedArray[i] = de.Value.ToString(); i++; } Array.Sort(sortedArray); Console.WriteLine("Contents of sorted array based on Hashtable values:"); foreach (var item in sortedArray) { Console.WriteLine(item); } } }
This program has a hash table object, numberNames. We fill it with numbers and their corresponding number names. By traversing the hash table, we fill the sorted array with values. We then sort the array using the Array.Sort() filter and print the sorted array.
Output
The output of the program is shown below.
Hashtable langCodes Contents: 18 (Eighteen) 12 (Twelve) 65 (Sixty Five) 2 (Two) 15 (Fifteen) Contents of sorted array based on Hashtable values: Eighteen Fifteen Sixty Five Twelve Two
From the output, we can see that the contents of the array (the values in the hash table) are indeed sorted alphabetically. We can easily compare the output of the hash table and the sorted array. In a hash table, the output is unsorted. Key-value pairs are displayed randomly. When in an array, the output is sorted.
Although sorting hash table elements is difficult, we can do it by representing hash table elements as an array. But it is not possible to sort keys and values at the same time. We can retrieve all keys in an array or all values in an array. We can then sort the array using the Array.Sort() filter. We can also convert the hash table to an array or list of arrays and process it.
The above is the detailed content of How to get hashtable elements as sorted array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


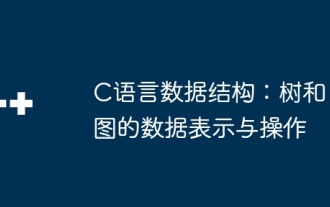
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
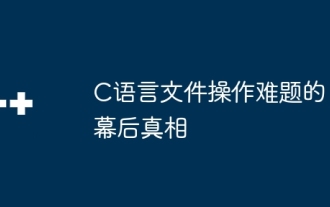
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
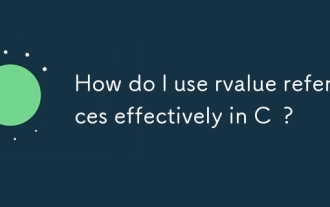
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
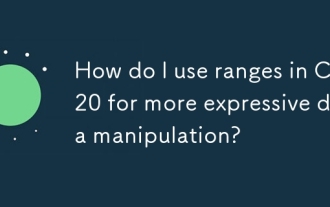
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
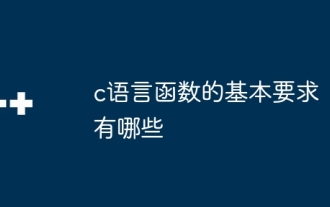
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
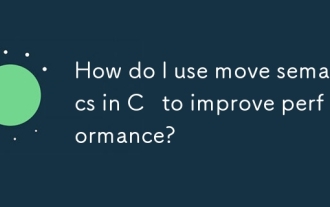
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
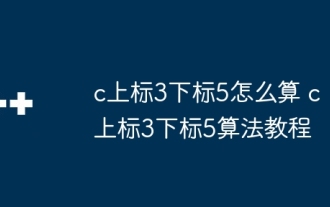
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
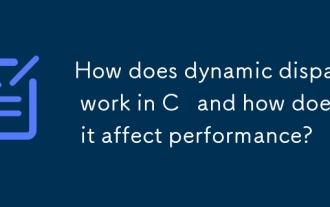
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
