C++ program: Find the second smallest element in a linked list
An ordered collection of data elements. Each data element has a link to its next element (sometimes its previous element). Assuming there is a linked list, then We need to find the second smallest element. Below is the following scenario.
Let’s assume some simple input and output scenarios
Assume this scenario, we have a linked list, the elements contained in it are "8->4->6->2->9,". Then after iterating the entire linked list, the second smallest element is 8.
Input = 8->4->6->2->9 Output = 8
Programming implementation of linked list
Node* head = new Node(8); head->next = new Node(4); head->next->next = new Node(6); head->next->next->next = new Node(2); head->next->next->next->next = new Node(9); result = solve(head);
Consider another situation where every element in the linked list has the same value. After iterating through all elements, the second smallest element will not be found in the linked list. Since each element in the linked list contains the same value.
Input = 1->1->1->1->1->1 Output = There is no second smallest element in Linked list
algorithm
Here are the steps to follow when performing the task
Assign two variables (S1, S2)
S1 will save the smallest element in the linked list
S2 will hold the second smallest element in the linked list.
Each iteration, the smallest element will be moved to S1, and when it is encountered, it will be moved to S2
When the minimum (S1) is smaller than the new smaller value, the new smaller will become the minimum (S1).
The new smaller one will become smaller (S1) and the smaller one (S1) will move to the second smaller one (S2).
After each possible traversal, the second smallest element in the final output will be the output.
Example
In the C implementation, we can keep 2 variables. 1 is the smallest, other is the second smallest, and then the linked list is traversed. Every time a smaller element is encountered, the smallest variable is updated to the next smallest, and the new smaller one becomes the smallest. So whenever an element is smaller than the smallest element, the second smallest element becomes the smallest and the smallest element becomes the new element. If not, we compare the second smallest element and determine if the current element is smaller than the second smallest element, and update accordingly.
#include <iostream> using namespace std; class Node { public: int val; Node *next; Node(int val) { this->val = val; next = NULL; } }; int solve(Node* root) { int s1=root->val, s2=root->val; while(root) { if(root->val <= s1) { s2 = s1; s1 = root->val; } else if(root->val < s2) { s2 = root->val; } root = root->next; } return s2; } int main() { Node* head = new Node(5); head->next = new Node(8); head->next->next = new Node(9); head->next->next->next = new Node(2); head->next->next->next->next = new Node(4); cout << "Second smallest element in the linked list is : " << solve(head); return 0; }
Output
Second smallest element in the linked list is: 4
in conclusion
Traverse the linked list once, the time complexity is O(n). If you found the above information useful, then do visit our official website to know more related topics about programming.
The above is the detailed content of C++ program: Find the second smallest element in a linked list. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


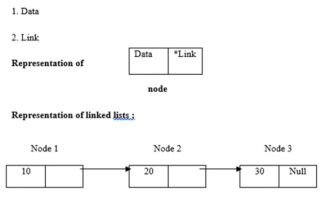
Linked lists use dynamic memory allocation, i.e. they grow and shrink accordingly. They are defined as collections of nodes. Here, a node has two parts, data and links. The representation of data, links and linked lists is as follows - Types of linked lists There are four types of linked lists, as follows: - Single linked list/Singly linked list Double/Doubly linked list Circular single linked list Circular double linked list We use the recursive method to find the length of the linked list The logic is -intlength(node *temp){ if(temp==NULL) returnl; else{&n
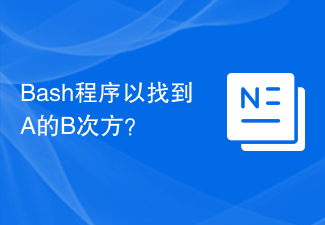
Here we will see how to get the number A raised to the power B using a bash script. The logic is simple. We have to use the "**" operator or the power operator to do this. Let us see the following program to understand this concept clearly. Example#!/bin/bash#GNUbashScripta=5b=6echo "$(($a**$b))" output 15625
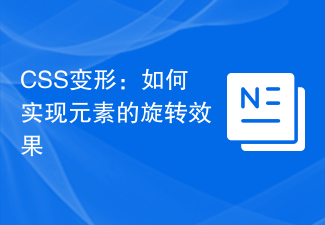
CSS transformation: How to achieve the rotation effect of elements requires specific code examples. In web design, animation effects are one of the important ways to improve user experience and attract user attention, and rotation animation is one of the more classic ones. In CSS, you can use the "transform" attribute to achieve various deformation effects of elements, including rotation. This article will introduce in detail how to use CSS "transform" to achieve the rotation effect of elements, and provide specific code examples. 1. How to use CSS’s “transf
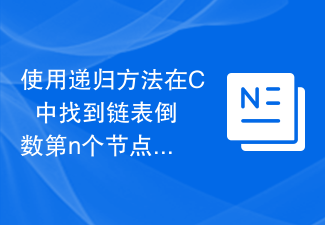
Given a singly linked list and a positive integer N as input. The goal is to find the Nth node from the end of the given list using recursion. If the input list has nodes a→b→c→d→e→f and N is 4, then the 4th node from the last will be c. We will first traverse until the last node in the list and when returning from the recursive (backtracking) increment count. When count equals N, a pointer to the current node is returned as the result. Let's look at various input and output scenarios for this - Input - List: -1→5→7→12→2→96→33N=3 Output − The Nth node from the last is: 2 Explanation − The third node is 2 . Input − List: -12→53→8→19→20→96→33N=8 Output – Node does not exist
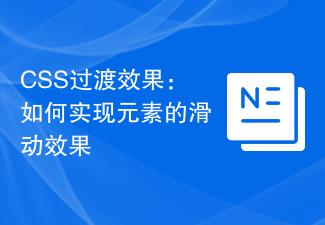
CSS transition effect: How to achieve the sliding effect of elements Introduction: In web design, the dynamic effect of elements can improve the user experience, among which the sliding effect is a common and popular transition effect. Through the transition property of CSS, we can easily achieve the sliding animation effect of elements. This article will introduce how to use CSS transition properties to achieve the sliding effect of elements, and provide specific code examples to help readers better understand and apply. 1. Introduction to CSS transition attribute transition CSS transition attribute tra
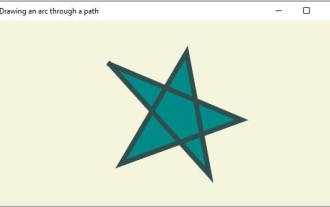
The javafx.scene.shape package provides some classes with which you can draw various 2D shapes, but these are just primitive shapes like lines, circles, polygons and ellipses etc... So if you want to draw complex For custom shapes, you need to use the Path class. Path class Path class You can draw custom paths using this geometric outline that represents a shape. To draw custom paths, JavaFX provides various path elements, all of which are available as classes in the javafx.scene.shape package. LineTo - This class represents the path element line. It helps you draw a straight line from the current coordinates to the specified (new) coordinates. HlineTo - This is the table
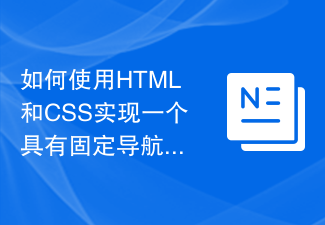
How to use HTML and CSS to implement a layout with a fixed navigation menu. In modern web design, fixed navigation menus are one of the common layouts. It can keep the navigation menu always at the top or side of the page, allowing users to browse web content conveniently. This article will introduce how to use HTML and CSS to implement a layout with a fixed navigation menu, and provide specific code examples. First, you need to create an HTML structure to present the content of the web page and the navigation menu. Here is a simple example
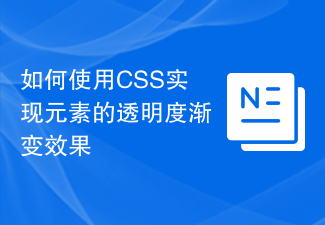
How to use CSS to achieve the transparency gradient effect of elements In web development, adding transition effects to web page elements is one of the important means to improve user experience. The gradient effect of transparency can not only make the page smoother, but also highlight the key content of the element. This article will introduce how to use CSS to achieve the transparency gradient effect of elements and provide specific code examples. Using the CSS transition attribute To achieve the transparency gradient effect of an element, we need to use the CSS transition attribute. t
