How to solve C++ runtime error: 'out of memory exception'?
How to solve C runtime error: 'out of memory exception'?
Introduction:
In C programming, we often encounter insufficient memory, especially when processing large data sets or complex algorithms. An 'out of memory exception' is thrown when the program cannot allocate additional memory to meet its needs. This article will describe how to solve this type of problem and give corresponding code examples.
- Check for memory leaks:
A memory leak means that the memory allocated in the program is not released, resulting in excessive memory usage and eventually insufficient memory. Therefore, we should first check whether there is a memory leak problem in the code. You can ensure the correct allocation and release of memory by using memory allocation debugging tools and rational use of new/delete or malloc/free.
Sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
- Reduce memory usage:
If memory leaks are not the problem, then you can avoid 'out of by reducing memory usage memory exception'. You can try the following methods: - Optimization algorithm: Optimize the complexity of the algorithm and reduce the memory requirements.
- Use data structures: Appropriate data structures can reduce memory usage and improve code execution efficiency.
- Reduce redundant data: remove unnecessary data and release unused memory space in a timely manner.
Sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
- Use appropriate data structures:
Choosing appropriate data structures is very important to reduce memory usage. For example, using a linked list instead of an array can avoid occupying contiguous blocks of memory. Using a hash table instead of an array reduces the storage of redundant data. Choosing the appropriate data structure according to actual needs can improve memory utilization efficiency.
Sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
- Increase available memory:
Sometimes, even if your program has no memory leaks and the memory usage is reasonable, you will still encounter an 'out of memory exception'. At this time, the problem can be solved by increasing the available memory. You can try the following methods: - Increase physical memory: Provide more available memory for programs to use by increasing the physical memory capacity of the computer.
- Reduce the memory usage of other programs: close some unnecessary programs or processes and release more memory for the current program to use.
- Error handling and exception catching:
Finally, in order to better handle the 'out of memory exception' exception, we need to use appropriate error handling and exception catching mechanisms. You can check the size of available memory before trying to allocate it. If the available memory is insufficient, appropriate error handling or exception catching can be performed to avoid program crashes.
Sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
Summary:
Handling 'out of memory exception' errors in C can start from checking memory leaks, reducing memory usage, and using appropriate Data structures, increasing available memory, and using error handling and exception catching are all addressed. Through the above methods, we can better manage memory, avoid the problem of insufficient memory, and improve the stability and performance of the program.
The above is the detailed content of How to solve C++ runtime error: 'out of memory exception'?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


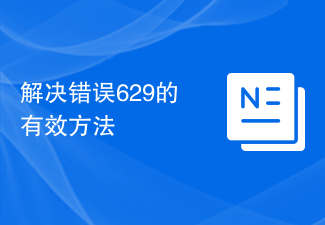
Error 629 refers to a common problem encountered when using a computer or network, indicating that a malfunction or error has occurred in the currently connected network or server. When error 629 occurs, users cannot access the Internet normally, which is very troublesome for many people. However, we don’t need to worry too much as error 629 usually has some simple solutions. First, we can try to restart the computer and network devices, such as routers or modems. Sometimes, this error may just be a temporary problem and a reboot can help restart the
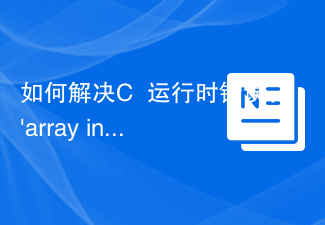
How to solve C++ runtime error: 'arrayindexoutofbounds' In C++ programming, array is one of the commonly used data structures. However, when we accidentally exceed the array index range in our code, a runtime error occurs: 'arrayindexoutofbounds'. This error is common, but relatively easy to fix. This article will introduce you to some workarounds to help you better understand and deal with this type of error. Common reasons for this error
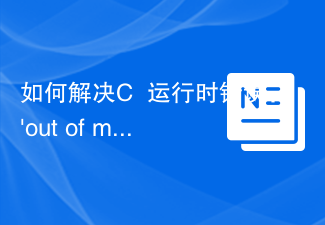
How to solve C++ runtime error: 'outofmemoryexception'? Introduction: In C++ programming, out of memory situations are often encountered, especially when processing large data sets or complex algorithms. 'outofmemoryexception' (out of memory exception) is thrown when the program cannot allocate additional memory to meet its needs. This article will describe how to solve this type of problem and give corresponding code examples. Check for memory leak issues: Memory Leak
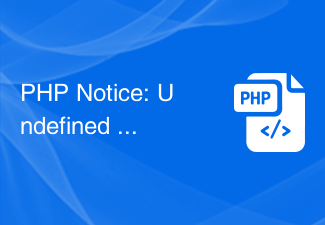
Solution to PHPNotice:Undefinedindex error When using PHP to develop applications, we often encounter the error message "PHPNotice:Undefinedindex". This error is usually caused by accessing an undefined array index. This article will introduce several methods to solve the Undefinedindex error and give corresponding code examples. Use isset() function to check if array index exists first
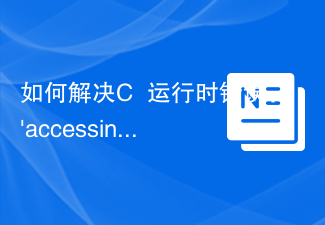
How to solve C++ runtime error: 'accessingnullpointer'? Introduction: C++ is a powerful and widely used programming language, but when writing code, we often encounter various errors. One of them is the "accessingnullpointer" runtime error, also known as NUll pointer access error. This article will describe how to resolve this error and provide some code examples to help readers understand better. What is a NUll pointer access error? NUL
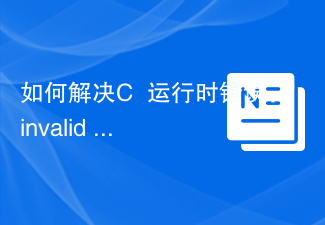
How to solve C++ runtime error: 'invalidformatspecifier'? When developing in C++, we often encounter various runtime errors. One of the more common errors is the 'invalidformatspecifier' error. This error usually occurs when using the printf or scanf function and indicates that we have used an invalid or mismatched format specifier in the format string.
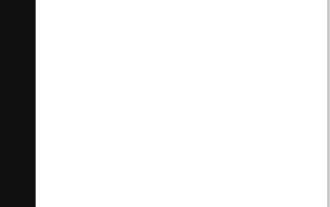
How to solve the script error on the current page is a very annoying thing, because although this prompt says that an error has occurred, it does not affect normal use. So what is the solution? Come and take a look at the detailed tutorial. ~How to solve the script error on the current page: 1. First, search and open "Control Panel" in the menu box. 2. Then click "System and Security". 3. Select "Change User Account Control Settings" in the first item. 4. Adjust notifications to Never notify, and then click OK.
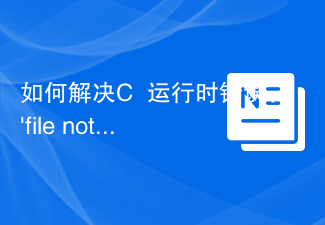
How to solve C++ runtime error: 'filenotfound'? In C++ programming, 'filenotfound' (file not found) is a common runtime error. This usually occurs when a program tries to open a file but discovers that the file does not exist. Such errors may cause the program to crash or produce unexpected behavior. Fortunately, we have several ways to solve this problem. 1. Check the file path. When receiving the 'filenotfound' error, first
