


How to call a function from a function name stored in a string using JavaScript?
We will use the eval() function to call a function from its name stored in a string. Evaluate() Function evaluates a string into JavaScript code. This allows us to call the function via Pass a string containing its name as an argument to the eval() function.
Example
This is a complete working example of how to use JavaScript to call a function from its name stored in a string -
// Define the function that we want to call function sayHello() { console.log("Hello, world!"); } // Store the function name as a string let functionName = "sayHello"; // Use the `eval()` function to convert the string to a function call eval(functionName + "()");
In the above example, we first define a simple function called sayHello(), which only logs "Hello, world!" to the console. Next, we define a variable called functionName that stores the string "sayHello", which is the name of the function we want to call.
Finally, we use the eval() function to convert the string functionName into a function call by concatenating it with the brackets () required to actually call the function.
The eval() function is a powerful but potentially dangerous feature of JavaScript that allows you to evaluate strings just like JavaScript code. Using eval() in production code is generally not recommended because it can be used to execute arbitrary code, which can be a security risk.
alternative method
It's worth mentioning that there are safer ways to call functions from function names stored in strings without using eval, such as -
let functionName = "sayHello"; let functionObj = window[functionName]; functionObj();
or
let functionName = "sayHello"; let functionObj = window['sayHello']; functionObj();
These alternatives are safer than using eval because they do not execute arbitrary code but refer to the function directly by name.
The above is the detailed content of How to call a function from a function name stored in a string using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
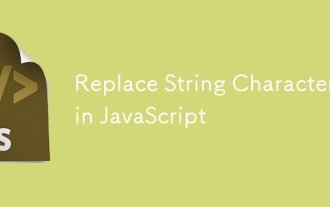
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
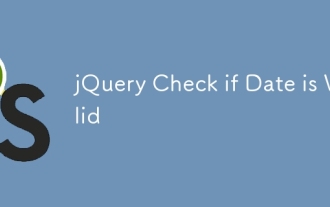
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
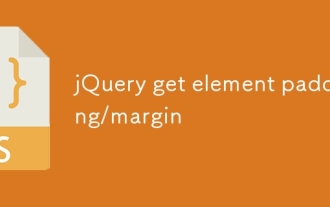
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
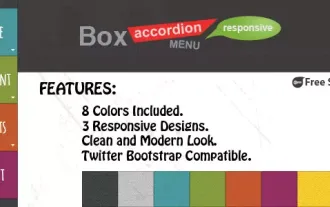
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
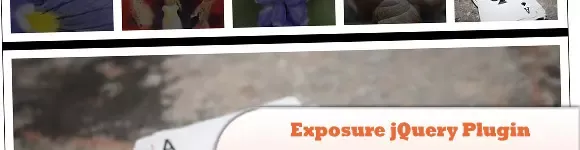
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
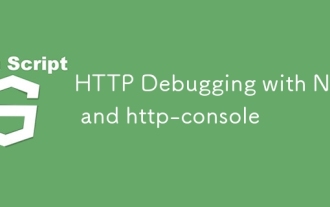
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
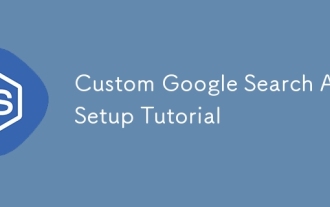
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
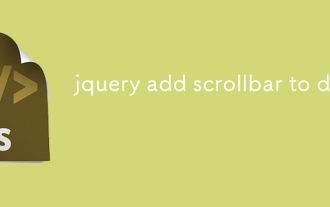
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c
