How to clear cache using JavaScript?
Cache, often called cache, is a different memory system in a computer that stores frequently used data and instructions for a short period of time. When loading a website, the browsers we use automatically cache some resources, such as images, scripts, and stylesheets, so that they can be used again when the page reloads. Not only does this shorten the time it takes for your website to load, it also helps reduce the amount of data that has to be sent over the network. But this kind of cache stored by the browser also has some disadvantages. Problems can occur if cached resources are out of date or if the browser is unable to reload the page because it is using cached resources. To do this we sometimes have to clear these caches.
Users can use JavaScript to clear the browser's cache as needed. These are described below -
location.reload() method - One of the effective methods that can be used to reload the current page and disable caching. The user must give a boolean value as parameter and the value should be set to true. Instead of using cached versions, this technique forces the browser to reload all resources from the server.
navigator.serviceWorker.getRegistrations() method - This is another way to retrieve all Service Worker registrations using navigator.serviceWorker and then run the unregister method for each registration object's getRegistrations() method. The browser will then delete its HTTP cache.
caches.open() and cache.delete() methods - This method uses the Cache API to open a named cache and then deletes a specific resource from the cache using the delete() method
localStorage.clear() and sessionStorage.clear() methods - To remove all key-value pairs from a localStorage object, the user can use the localStorage.clear() method. The sessionStorage.clear() function can delete all key-value pairs from the sessionStorage object.
Use location.reload() method
When the boolean parameter is set to true, the location.reload() method will not cache the current page, but will reload it. If you specify true as a parameter, the browser will download all resources (including images and scripts) directly from the server instead of using cached copies.
grammar
location.reload(true);
In the above syntax, location is the global object of JavaScript and overloads the location method.
Example
In this example, we use the location.reload() method to clear cache memory through JavaScript. We used a "clear cache" button and associated it with a click event. The click event handler executes the location.reload() method with parameter true. Whenever the user clicks the button, the JavaScript forces the browser to reload the web page without the cached file.
<html> <body> <h2>Clearing <i> cache memory </i> using JavaScript</h2> <div> <img id = "myImage" style = "height: 200px" src ="https://www.tutorialspoint.com/javascript/images/javascript.jpg"/> </div> <button onclick = "clearCache()">Clear cache</button> <div id = "root" style="padding: 10px; background: #bdf0b8"></div> <script> let root = document.getElementById('root') function clearCache() { root.innerHTML += 'Cache cleared using location.reload(true)' windows.location.reload(true) } </script> </body> </html>
The web page displays this message and quickly reloads the latest files.
Use navigator.serviceWorker.getRegistrations() method
In JavaScript, the navigator.serviceWorker.getRegistrations() method is the second way that users can clear cache memory by unregistering all service worker thread registrations, navigator.serviceWorker.getRegistrations( ) method can be used to clear the browser's HTTP cache. One type of Web Worker is called a Service Worker and is used to perform a large number of processes in the background, such as caching resources. The browser must clear its HTTP cache by deactivating all service worker registrations.
grammar
if ('serviceWorker' in navigator) { navigator.serviceWorker.getRegistrations() .then(function(registrations) { for(let registration of registrations) { registration.unregister(); } }); }
In the above syntax, we check if "serviceWorker" is available in the navigator object. We then unregister the Service Worker using the navigator.serviceWorker.getRegistrations() and registration.unregister() methods.
Example
In this example, we clear the cache by using JavaScript to unregister the Service Worker. We used a "clear cache" button and associated it with a click event. The click event handler executes a function that unregisters the Service Worker. The navigator.serviceWorker.getRegistrations() and registration.unregister() methods are used to unregister a Service Worker. After logging out, we display a message on the web page.
<html> <body> <h2>Clearing <i>cache memory</i> using JavaScript</h2> <div> <img id = "myImage" style = "height: 100px" src ="https://www.tutorialspoint.com/images/logo.png" /> </div> <button onclick = "clearCache()">Clear cache</button> <div id = "root" style = "padding: 10px; background: #b8f0ea"></div> <script> let root = document.getElementById('root') function clearCache() { root.innerHTML = 'Cache cleared using navigator.serviceWorker.getRegistrations()' if ('serviceWorker' in navigator) { navigator.serviceWorker .getRegistrations() .then(function (registrations) { for (let registration of registrations) { registration.unregister() } }) } } </script> </body> </html>
Clearing the cache is a best practice, but sometimes it can affect the performance of your web pages because all files must be re-fetched. JavaScript has the ability to clear cache, which can be used as needed.
The above is the detailed content of How to clear cache using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


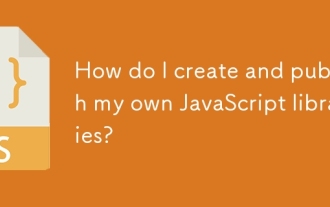
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
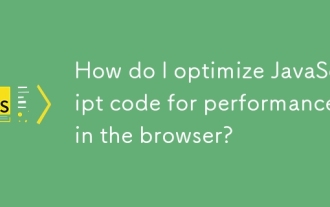
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
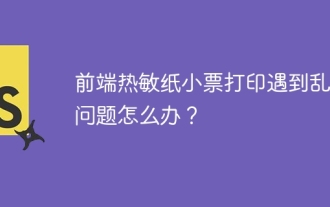
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
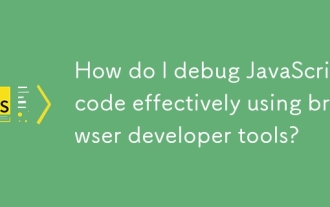
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
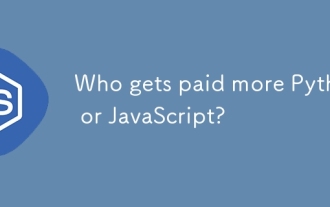
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
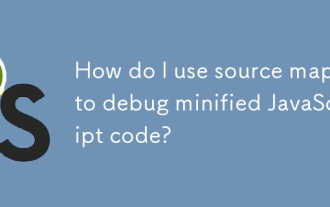
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
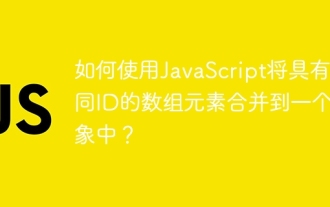
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
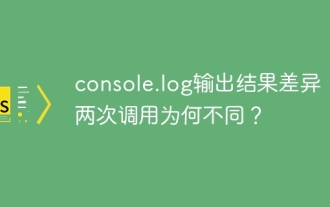
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
