


Master C++ programming skills for the development of various functions of embedded systems
Master C programming skills for the development of various functions of embedded systems
In the field of embedded systems, C is a widely used programming language. Its powerful object-oriented features and efficient performance make C one of the preferred languages for developing various functions of embedded systems. This article will introduce some C programming techniques commonly used in embedded system development, and illustrate them with code examples.
1. Use classes and objects for modular design
Object-oriented programming is one of the biggest features of C. In embedded system development, modular design using classes and objects can make the code structure clearer and easier to maintain and reuse. For example, to develop a module that controls LED lights, you can define a class named LED, which contains methods and member variables for operating LED lights. The following is a simple sample code:
class LED { public: void turnOn(); void turnOff(); private: int pin; }; void LED::turnOn() { // 控制LED灯点亮的代码 } void LED::turnOff() { // 控制LED灯关闭的代码 }
Using the LED class can easily operate multiple LED lights, improving the readability and maintainability of the code.
2. Use virtual functions to achieve polymorphism
Polymorphism is one of the very important concepts in object-oriented programming. By using virtual functions, unified calls between different derived classes can be achieved. In embedded system development, virtual functions can be used to achieve a unified interface for different hardware devices. The following is a simple sample code:
class Device { public: virtual void init() = 0; virtual void sendData(const char* data) = 0; }; class UART : public Device { public: void init() override { // UART设备初始化的代码 } void sendData(const char* data) override { // 发送数据的代码 } }; class SPI : public Device { public: void init() override { // SPI设备初始化的代码 } void sendData(const char* data) override { // 发送数据的代码 } }; void initialize(Device* device) { device->init(); } void sendData(Device* device, const char* data) { device->sendData(data); }
By defining an abstract Device class and implementing virtual functions in derived classes, the operations of initialization and data sending can be uniformly called on different devices.
3. Use smart pointers to manage dynamic memory
In embedded system development, dynamic memory management is a very important issue. To avoid problems like memory leaks and dangling pointers, you can use smart pointers for memory management. The following is a sample code:
class Example { public: Example() { // 创建资源的代码 } ~Example() { // 释放资源的代码 } }; void exampleFunc() { std::shared_ptr<Example> example = std::make_shared<Example>(); // 使用example指向的资源 // ... // 不需要再手动释放资源,智能指针会自动管理内存 }
By using std::shared_ptr, memory can be automatically released during dynamic memory allocation, avoiding the problem of memory leaks.
This article introduces some C programming techniques commonly used in embedded system development, and illustrates them with code examples. Mastering these skills can make embedded system development more efficient and easier to maintain. Of course, this is just the tip of the iceberg of C programming skills. I hope readers can further learn and apply them to improve their abilities in the field of embedded system development.
The above is the detailed content of Master C++ programming skills for the development of various functions of embedded systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Utilizing C++ to implement real-time audio and video processing functions of embedded systems The application range of embedded systems is becoming more and more extensive, especially in the field of audio and video processing, where the demand is growing. Faced with such demand, using C++ language to implement real-time audio and video processing functions of embedded systems has become a common choice. This article will introduce how to use C++ language to develop real-time audio and video processing functions of embedded systems, and give corresponding code examples. In order to realize the real-time audio and video processing function, you first need to understand the basic process of audio and video processing. Generally speaking, audio and video
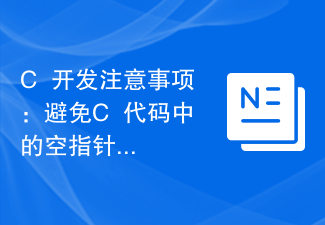
In C++ development, null pointer exception is a common error, which often occurs when the pointer is not initialized or is continued to be used after being released. Null pointer exceptions not only cause program crashes, but may also cause security vulnerabilities, so special attention is required. This article will explain how to avoid null pointer exceptions in C++ code. Initializing pointer variables Pointers in C++ must be initialized before use. If not initialized, the pointer will point to a random memory address, which may cause a Null Pointer Exception. To initialize a pointer, point it to an
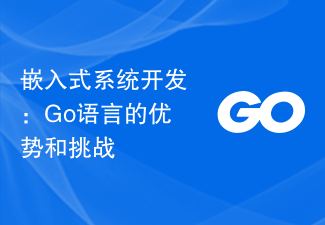
Embedded system development has always been a challenging task in the field of information technology, which requires developers to have deep technical knowledge and rich experience. As embedded devices become more complex and functional requirements become more diverse, choosing a programming language suitable for development has become critical. In this article, we will delve into the advantages and challenges of Go language in embedded system development and provide specific code examples to help readers better understand. As a modern programming language, Go language is known for its simplicity, efficiency, reliability and
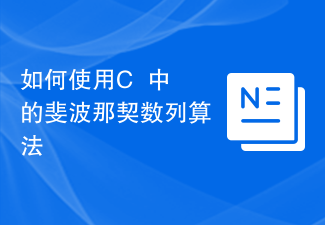
How to use the Fibonacci sequence algorithm in C++ The Fibonacci sequence is a very classic sequence, and its definition is that each number is the sum of the previous two numbers. In computer science, using the C++ programming language to implement the Fibonacci sequence algorithm is a basic and important skill. This article will introduce how to use C++ to write the Fibonacci sequence algorithm and provide specific code examples. 1. Recursive method Recursion is a common method of Fibonacci sequence algorithm. In C++, the Fibonacci sequence algorithm can be implemented concisely using recursion. under
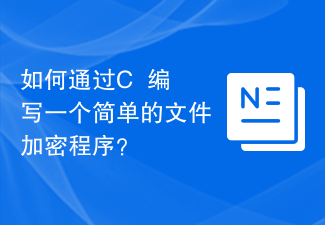
How to write a simple file encryption program in C++? Introduction: With the development of the Internet and the popularity of smart devices, the importance of protecting personal data and sensitive information has become increasingly important. In order to ensure the security of files, it is often necessary to encrypt them. This article will introduce how to use C++ to write a simple file encryption program to protect your files from unauthorized access. Requirements analysis: Before starting to write a file encryption program, we need to clarify the basic functions and requirements of the program. In this simple program we will use symmetry
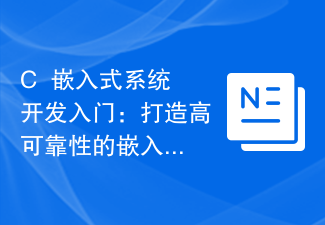
Embedded systems refer to applications that run on specific hardware platforms and are typically used to control, monitor, and process various devices and systems. As a powerful programming language, C++ is widely used in embedded system development. This article will introduce the basic concepts and techniques of C++ embedded system development, and how to create high-reliability embedded applications. 1. Overview of Embedded System Development Embedded system development requires a certain understanding of the hardware platform, because embedded applications need to interact directly with the hardware. In addition to hardware platforms, embedded systems
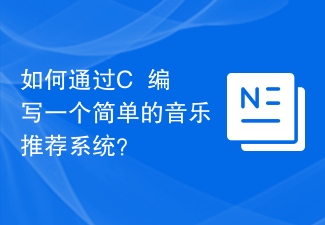
How to write a simple music recommendation system in C++? Introduction: Music recommendation system is a research hotspot in modern information technology. It can recommend songs to users based on their music preferences and behavioral habits. This article will introduce how to use C++ to write a simple music recommendation system. 1. Collect user data First, we need to collect user music preference data. Users' preferences for different types of music can be obtained through online surveys, questionnaires, etc. Save data in a text file or database
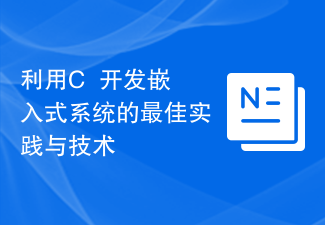
Best practices and technologies for developing embedded systems using C++ Summary: With the wide application of embedded systems in various fields, using C++ to develop efficient and reliable embedded systems has become an important task. This article will introduce the best practices and technologies for developing embedded systems using C++, including system architecture, code optimization and debugging techniques, and demonstrate specific implementation methods through code examples. Introduction With the continuous development of hardware technology, embedded systems have been widely used in various fields such as automobiles, home appliances, and medical equipment. For embedded systems, the
