


How to use PHP and Vue.js to implement data filtering and sorting functions on charts
How to use PHP and Vue.js to implement data filtering and sorting functions on charts
In web development, charts are a very common way of displaying data. Using PHP and Vue.js, you can easily implement data filtering and sorting functions on charts, allowing users to customize the viewing of data on charts, improving data visualization and user experience.
First, we need to prepare a set of data for chart use. Suppose we have a data table that contains three columns: name, age and grades. The data is as follows:
Name | Age | grade |
---|---|---|
张三 | 18 | 90 |
李四 | 20 | 80 |
王五 | 22 | 85 |
赵六 | 19 | 95 |
Next, we use PHP to pass the data to Vue.js and implement it on the front end Data filtering and sorting capabilities. First, in the back-end PHP file, we can use the following code to query the data and return it to the front-end:
<?php // 连接数据库 $conn = new mysqli("localhost", "root", "password", "database"); // 查询数据 $sql = "SELECT * FROM students"; $result = $conn->query($sql); // 将查询结果转化为JSON格式返回给前端 $data = []; while ($row = $result->fetch_assoc()) { $data[] = $row; } echo json_encode($data); // 关闭数据库连接 $conn->close(); ?>
Using Vue.js in the front-end, we can get and bind the data to the chart through an ajax request On the required data variables:
new Vue({ el: "#app", data: { students: [], filteredStudents: [] }, mounted() { // 发送ajax请求获取数据 axios.get("data.php").then(response => { this.students = response.data; this.filteredStudents = response.data; }); }, methods: { filterData() { // 根据选择的筛选条件,过滤数据并更新到filteredStudents }, sortData(fieldName, direction) { // 根据指定的字段和排序方向,对数据进行排序并更新到filteredStudents } } });
In the front-end code, we created a Vue instance and defined two variables in the data attribute: students and filteredStudents, which store original data and filtered data respectively. Send an ajax request to obtain data in mounted(), and bind the data to the corresponding variable in the then() method.
Next, we can add interactive elements for filtering and sorting on the page. For example, in the drop-down list of filter conditions, we can add the following code:
<select v-model="ageFilter" @change="filterData"> <option value="">全部年龄</option> <option value="18">18岁</option> <option value="19">19岁</option> <option value="20">20岁</option> <option value="21">21岁</option> <option value="22">22岁</option> </select>
In the sortData() method, we can use JavaScript's array sorting method to sort the data. For example, the code for sorting by name in ascending order is as follows:
sortData(fieldName, direction) { this.filteredStudents.sort((a, b) => { return a[fieldName] > b[fieldName] ? 1 : -1; }); if (direction === "desc") { this.filteredStudents.reverse(); } }
Finally, use Vue data binding in HTML to display the data on the chart:
<table> <tr v-for="student in filteredStudents"> <td>{{ student.name }}</td> <td>{{ student.age }}</td> <td>{{ student.grade }}</td> </tr> </table>
Through the above code example, we can use PHP and Vue.js implement data filtering and sorting functions on charts. Users can select specific data through filter conditions, and then sort the data through the sorting function, thereby improving the visualization of chart data and user experience.
The above is the detailed content of How to use PHP and Vue.js to implement data filtering and sorting functions on charts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


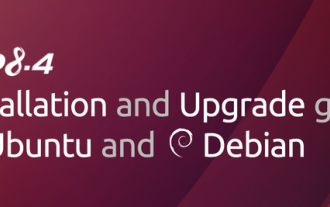
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
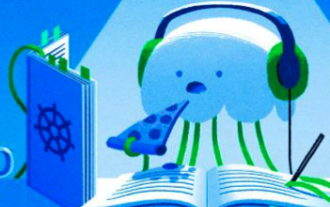
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
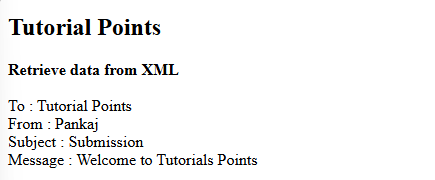
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
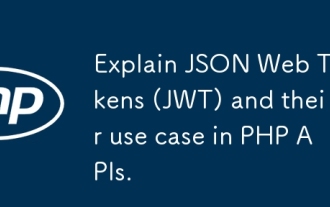
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
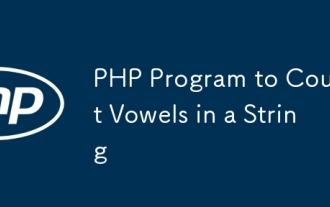
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
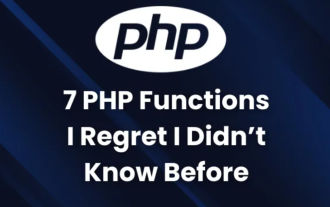
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
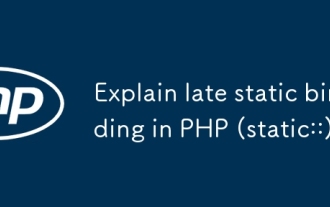
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
