How to develop autonomous driving and intelligent navigation in C++?
How to develop automatic driving and intelligent navigation in C?
Autonomous driving and intelligent navigation are one of the hot areas of technological development today. With the rapid development of computer hardware technology and the continuous improvement of algorithms, C language is increasingly used in the fields of autonomous driving and intelligent navigation. This article will introduce how to develop autonomous driving and intelligent navigation in C and provide code examples.
- Sensor data acquisition and processing
Autonomous driving and intelligent navigation systems require the use of various sensors to obtain environmental data, such as cameras, lidar, GPS, etc. The C language provides a wealth of libraries and tools to facilitate us to obtain and process these sensor data.
Taking the camera as an example, we can use the OpenCV library to obtain the image data of the camera and process it. The following is a simple code example:
#include <opencv2/opencv.hpp> int main() { cv::VideoCapture cap(0); // 打开摄像头 if (!cap.isOpened()) { std::cerr << "Unable to open camera!" << std::endl; return -1; } cv::Mat frame; while (cap.read(frame)) { // 读取每一帧图像 // 图像处理代码 cv::imshow("Camera", frame); if (cv::waitKey(1) == 27) { // 按下ESC键退出 break; } } cap.release(); // 释放摄像头资源 cv::destroyAllWindows(); return 0; }
- Data fusion and perception
In autonomous driving and intelligent navigation systems, the fusion and perception of sensor data are crucial This step can be achieved by using filtering algorithms, machine learning and other methods.
A common method is to use a Kalman filter, which can fuse data from multiple sensors and provide a more accurate estimate. Here is a simple code example that demonstrates how to use a Kalman filter to fuse accelerometer and gyroscope data:
#include <iostream> #include <Eigen/Dense> int main() { Eigen::MatrixXd A(2, 2); // 状态转移矩阵 Eigen::MatrixXd B(2, 1); // 控制矩阵 Eigen::MatrixXd C(1, 2); // 观测矩阵 Eigen::MatrixXd Q(2, 2); // 过程噪声协方差矩阵 Eigen::MatrixXd R(1, 1); // 观测噪声协方差矩阵 // 初始化参数 A << 1, 1, 0, 1; B << 0.5, 1; C << 1, 0; Q << 0.1, 0, 0, 0.1; R << 1; Eigen::Vector2d x_hat; // 状态估计向量 Eigen::MatrixXd P_hat(2, 2); // 状态协方差矩阵 // 初始化状态估计向量和状态协方差矩阵 x_hat << 0, 0; P_hat << 1, 0, 0, 1; double u, z; for (int i = 0; i < 100; ++i) { // 获取传感器数据 u = 1; z = 2; // 预测步骤 x_hat = A * x_hat + B * u; P_hat = A * P_hat * A.transpose() + Q; // 更新步骤 Eigen::MatrixXd K = P_hat * C.transpose() * (C * P_hat * C.transpose() + R).inverse(); Eigen::Vector2d y = z - C * x_hat; x_hat = x_hat + K * y; P_hat = (Eigen::MatrixXd::Identity(2, 2) - K * C) * P_hat; std::cout << "x_hat: " << x_hat << std::endl; } return 0; }
- Path Planning and Control
Automatic Driving and intelligent navigation systems require path planning and control based on environmental data to achieve autonomous navigation. C language provides a powerful numerical calculation library and control library to facilitate the development of path planning and control algorithms.
Taking a simple PID control algorithm as an example, the following is a sample code:
#include <iostream> class PIDController { public: PIDController(double kp, double ki, double kd) : kp_(kp), ki_(ki), kd_(kd), error_sum_(0), prev_error_(0) {} double calculate(double setpoint, double input) { double error = setpoint - input; error_sum_ += error; double d_error = error - prev_error_; prev_error_ = error; double output = kp_ * error + ki_ * error_sum_ + kd_ * d_error; return output; } private: double kp_; double ki_; double kd_; double error_sum_; double prev_error_; }; int main() { PIDController pid_controller(0.1, 0.01, 0.01); double setpoint = 10; double input = 0; for (int i = 0; i < 100; ++i) { double output = pid_controller.calculate(setpoint, input); input += output; std::cout << "Output: " << output << std::endl; } return 0; }
Summary:
This article introduces how to perform automatic driving and intelligent navigation in C development. We first learned about the acquisition and processing of sensor data, then introduced the methods of data fusion and perception, and finally explained the algorithms for path planning and control. Through these code examples, I believe readers can better understand the basic principles and methods of developing autonomous driving and intelligent navigation in C so that they can be applied in actual projects. I hope this article will be helpful to readers' study and work.
The above is the detailed content of How to develop autonomous driving and intelligent navigation in C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


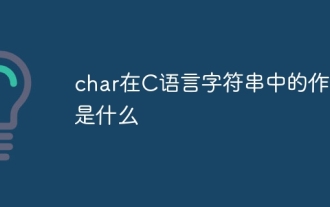
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
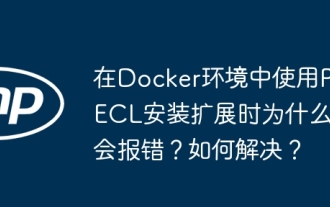
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
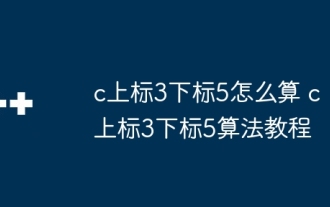
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
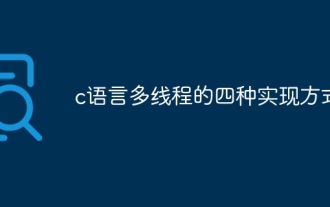
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
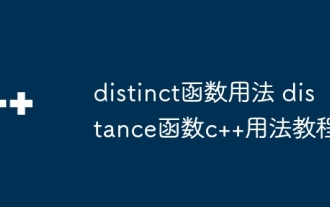
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
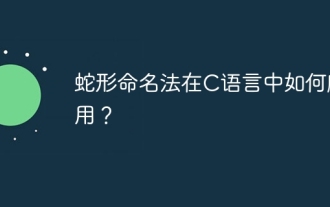
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
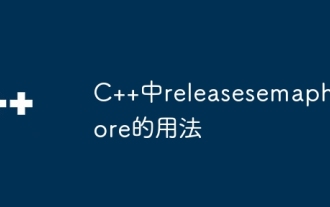
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
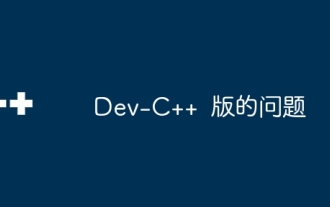
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
