What are decorators and how are they used in JavaScript?
In this tutorial, you will learn about JavaScript decorators and understand their inner workings and uses.
What are decorators in JavaScript?
The word decorator means to combine one set of code or program with another set of code or program, or it can be said to wrap a function with another function to extend the function or work of that function. Decorators can also be called decorator functions.
Developers have been using this decorator term in other languages like Python, C#, and now JavaScript has also introduced decorators.
Function Decorator
In JavaScript, functions behave like objects, so they are also called first class objects, this happens because we can assign functions to variables or return functions from functions, or we can pass functions as parameters Give function.
Example
const func_variable= function(){ console.log("Hey there"); } func_variable()
Example
Passing a function to another function
// MainFunction function takes a function as a parameter function MainFunction(func) { func() console.log("Main function") } // Assigning function to a variable var argumentFunction = function() { console.log("passed function") } //passing argumentFunction function to to MainFunction MainFunction(argumentFunction)
Example
Returning a function through another function
function SumElement(par1, par2) { var addNumbers = function () { result = par1+par2; console.log("Sum is:", result); } // Returns the addNumbers function return addNumbers } var PrintElement = SumElement(3, 4) console.log(PrintElement); PrintElement()
Higher-order functions
Higher-order functions refer to functions that take a function as a parameter and return that function after performing certain operations. The function discussed above is a higher-order function, namely printAdditionFunc.
Example
// higher order function function PrintName(name) { return function () { console.log("My name is ", name); } } // output1 is a function, PrintName returns a function var output1 = PrintName("Kalyan") output1() var output2= PrintName("Ashish") output2()
You may be thinking, we already have decorators as higher-order functions, so why do we need separate decorators?
So, we have the function decorator, which is a higher-order JavaScript function, but when the class appears in JavaScript, we also have functions within the class, where the higher-order function becomes failed, just like the decorator.
Example
Let’s look at the problem of higher-order functions of classes -
// higher is a decorator function function higher(arg) { return function() { console.log("First "+ arg.name) arg() // Decorator function call console.log("Last " + arg.name) } } class Website { constructor(fname, lname) { this.fname = fname this.lname = lname } websiteName() { return this.fname + " " + this.lname } } var ob1 = new Website("Tutorials", "Point") // Passing the class method websiteName to the higher order function var PrintName = higher(ob1.websiteName) PrintName()
What happens here is that when the higher function is called, it calls its argument, which is a member function of the class, as the websiteName function here. Since the function websiteName is called from an external class function, the value of the function is undefined inside the websiteName function. So this is the reason behind this log error.
So, to solve this problem, we will also pass an object of class Website which will eventually hold the value of this.
//higher is a decorator function function higher(ob1, arg) { return function() { console.log("Execution of " + arg.name + " begin") arg.call(ob1) //// Decorator function call console.log("Execution of " + arg.name + " end") } } class Website { constructor(fname, lname) { this.fname = fname this.lname = lname } websiteName() { return this.fname + " " + this.lname } } var ob1 = new Website("Tutorials", "Point") //Passing the class method websiteName to the higher order function var PrintName = higher(ob1, ob1.websiteName) PrintName()
In the PrintName function, we call the arg (which is a websiteName function) through the call function, which calls the websiteName function with the help of the Website class object, so the value of the pointer will be the object of the Website class and is the object with fname and lname variables so it will work without any error.
I hope you will understand decorators and their uses with the help of this article.
The above is the detailed content of What are decorators and how are they used in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
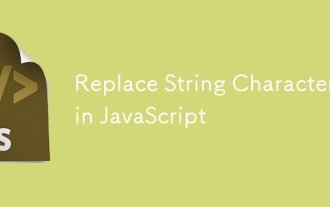
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
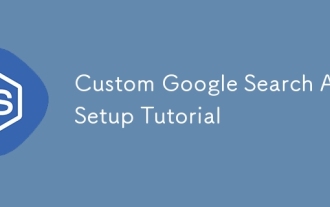
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
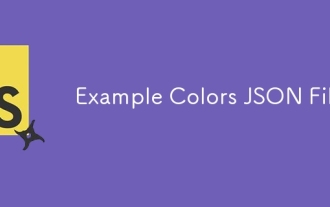
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
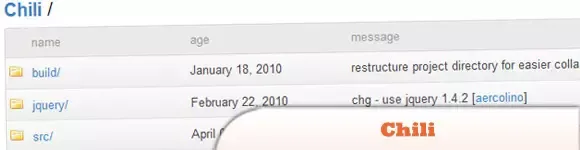
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
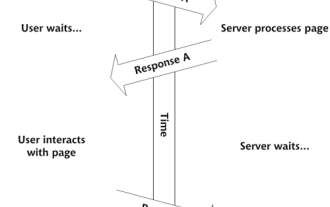
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
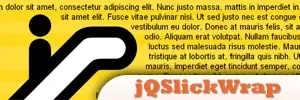
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
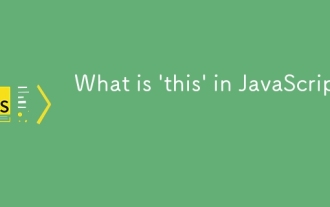
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
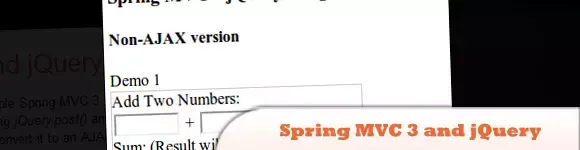
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
