


C++ program written using recursion to calculate the product of two numbers
Recursion is a technique of calling a function from the same function itself. There must be some base or terminating condition to end the recursive call. Recursive procedures are very helpful for performing complex iterative solutions with less code and finding easier solutions through sub-operations.
In this article, we will discuss the recursive method of performing the product (multiplication) between two numbers in C. First we understand the basic principles, recursive function calling syntax, algorithm and source code.
Use recursive multiplication
In high-level languages, there are multiplication operators that can perform multiplication directly. However, we know that multiplication is actually repeated addition. So the result of A*B is the number of repeated additions of A and B, or it can be said that the number of repeated additions of B and A. Whenever there are repetitions, we can do this using recursion. Let's look at the recursive function definition syntax first.
grammar
<return type> function_name ( parameter list ) { if ( base condition ) { terminate recursive call } recursive function call: function_name ( updated parameter list ) }
Algorithm
Let’s look at an algorithm that performs multiplication using recursion.
- Define a function multiply(), which accepts two numbers A and B
- If A < B, then
< B,则
- Return multiplication (B, A)
- Otherwise when B is not 0, then
- Return A multiplication (A, B - 1)
- otherwise
- Return 0
- If it ends
- If A < B, then
< B,则
- End of function definition
- Read two inputs A and B
- res = multiplication (A, B)
- Show nothing
Example
#include <iostream> #include <sstream> using namespace std; int multiply( int A, int B) { if( A < B ) { return multiply( B, A ); } else if( B != 0 ) { return A + multiply( A, B - 1 ); } else { return 0; } } int main() { cout << "Multiplication of 5, 7 is: " << multiply( 5, 7 ) << endl; cout << "Multiplication of 8, 0 is: " << multiply( 8, 0 ) << endl; cout << "Multiplication of 25, 3 is: " << multiply( 25, 3 ) << endl; cout << "Multiplication of 9, 1 is: " << multiply( 9, 1 ) << endl; }
Output
Multiplication of 5, 7 is: 35 Multiplication of 8, 0 is: 0 Multiplication of 25, 3 is: 75 Multiplication of 9, 1 is: 9
Look, in this program, the function parameters A and B are both integers. Now, after each step, it decrements the second parameter B by 1 and adds A to A itself. Like this, the function is performing the multiplication process.
in conclusion
Recursion is the process of calling the same function from the function itself. When calling a function recursively, we update or change the parameter set slightly so that the same effect does not occur again and again, and then divide the problem into smaller sub-problems and solve the problem by solving these smaller problems in a bottom-up approach . Almost anything that can be implemented using a loop can also be implemented using recursion. In this article, we saw the simple process of multiplying two integers using recursion. Add integers multiple times to get the final multiplication result.
The above is the detailed content of C++ program written using recursion to calculate the product of two numbers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The recursion depth of C++ functions is limited, and exceeding this limit will result in a stack overflow error. The limit value varies between systems and compilers, but is usually between 1,000 and 10,000. Solutions include: 1. Tail recursion optimization; 2. Tail call; 3. Iterative implementation.
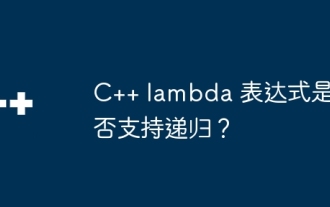
Yes, C++ Lambda expressions can support recursion by using std::function: Use std::function to capture a reference to a Lambda expression. With a captured reference, a Lambda expression can call itself recursively.

The recursive algorithm solves structured problems through function self-calling. The advantage is that it is simple and easy to understand, but the disadvantage is that it is less efficient and may cause stack overflow. The non-recursive algorithm avoids recursion by explicitly managing the stack data structure. The advantage is that it is more efficient and avoids the stack. Overflow, the disadvantage is that the code may be more complex. The choice of recursive or non-recursive depends on the problem and the specific constraints of the implementation.
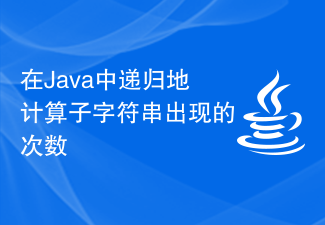
Given two strings str_1 and str_2. The goal is to count the number of occurrences of substring str2 in string str1 using a recursive procedure. A recursive function is a function that calls itself within its definition. If str1 is "Iknowthatyouknowthatiknow" and str2 is "know" the number of occurrences is -3. Let us understand through examples. For example, input str1="TPisTPareTPamTP", str2="TP"; output Countofoccurrencesofasubstringrecursi
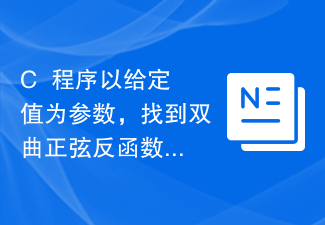
Hyperbolic functions are defined using hyperbolas instead of circles and are equivalent to ordinary trigonometric functions. It returns the ratio parameter in the hyperbolic sine function from the supplied angle in radians. But do the opposite, or in other words. If we want to calculate an angle from a hyperbolic sine, we need an inverse hyperbolic trigonometric operation like the hyperbolic inverse sine operation. This course will demonstrate how to use the hyperbolic inverse sine (asinh) function in C++ to calculate angles using the hyperbolic sine value in radians. The hyperbolic arcsine operation follows the following formula -$$\mathrm{sinh^{-1}x\:=\:In(x\:+\:\sqrt{x^2\:+\:1})}, Where\:In\:is\:natural logarithm\:(log_e\:k)
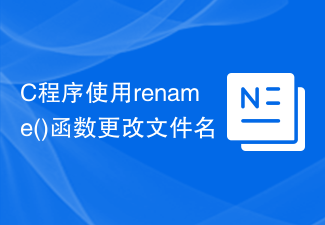
The rename function changes a file or directory from its old name to its new name. This operation is similar to the move operation. So we can also use this rename function to move files. This function exists in the stdio.h library header file. The syntax of the rename function is as follows: intrename(constchar*oldname,constchar*newname); The function of the rename() function accepts two parameters. One is oldname and the other is newname. Both parameters are pointers to constant characters that define the old and new names of the file. Returns zero if the file was renamed successfully; otherwise, returns a nonzero integer. During a rename operation
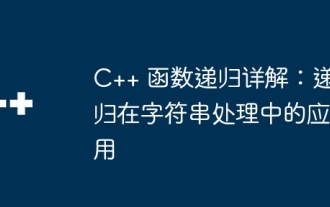
A recursive function is a technique that calls itself repeatedly to solve a problem in string processing. It requires a termination condition to prevent infinite recursion. Recursion is widely used in operations such as string reversal and palindrome checking.
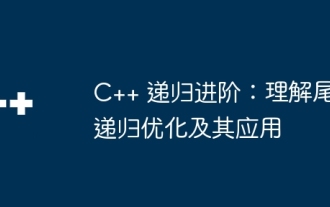
Tail recursion optimization (TRO) improves the efficiency of certain recursive calls. It converts tail-recursive calls into jump instructions and saves the context state in registers instead of on the stack, thereby eliminating extra calls and return operations to the stack and improving algorithm efficiency. Using TRO, we can optimize tail recursive functions (such as factorial calculations). By replacing the tail recursive call with a goto statement, the compiler will convert the goto jump into TRO and optimize the execution of the recursive algorithm.
