


Rearrange an array so that arr becomes arr] and only use O(1) extra space, implemented in C++
We get an array of positive integer type, say, arr[] of any given size, such that the element value in the array should be greater than 0 but less than the size of the array. The task is to rearrange An array that only turns arr[i] into arr[arr[i]] in the given O(1) space and prints the final result.
Let’s look at various input and output scenarios for this situation −
Input− int arr[] = {0 3 2 1 5 4 }
Output− Array before sorting: 0 3 2 1 5 4 Rearrange the array so that arr[i] becomes arr[arr[i]], with O(1) extra space: 0 1 2 3 4 5
Explanation− We give Define an integer array of size 6, and all elements in the array have values less than 6. Now, we will rearrange the array so that arr[arr[0] is 0, arr[arr[1]] is 1, arr[arr [2]] is 2, arr[arr[3]] is 3, arr[ arr[4]] is 4, arr[arr[5]] is 5. Therefore, the final array after rearrangement is 0 1 2 3 4 5.
input− int arr[] = {1, 0}
output − Array before sorting: 1 0 Rearrange the array so that arr[i] becomes arr[arr[i]], where O(1) extra space is: 0 1
Explanation - We get an integer of size 2 An array where all elements in the array have values less than 2. Now, we will rearrange the array so that arr[arr[0] is 1 and arr[arr[1]] is 0. Therefore, the final array after rearrangement is 0 1.
Input− int arr[] = {1, 0, 2, 3}
Output−Array before sorting: 1 0 2 3 Rearrange the array so that arr[i] becomes arr[arr[i]], with O(1) extra space: 0 1 2 3
Explanation - We give the size is an integer array of 4, and all elements in the array have values less than 4. Now, we will rearrange the array so that arr[arr[0] is 0, arr[arr[1]] is 1, arr[arr[2] ]] is 2, and arr[arr[3]] is 3. Therefore, the final array after rearrangement is 0 1 2 3.
The method used in the following program is as follows
Input an array of integer elements and calculate the array size
Before printing array, call the function Rearrangement (arr, size)
-
Function internal rearrangement (arr, size)
Start loop FOR from i to 0 until i is less than size. Inside the loop, set temp to arr[arr[i]] % size and arr[i] = temp * size.
Start looping FOR from i to 0 until i is less than size. Within the loop, set arr[i] = arr[i] / size
to print the result.
Example
#include <bits/stdc++.h> using namespace std; void Rearrangement(int arr[], int size){ for(int i=0; i < size; i++){ int temp = arr[arr[i]] % size; arr[i] += temp * size; } for(int i = 0; i < size; i++){ arr[i] = arr[i] / size; } } int main(){ //input an array int arr[] = {0, 3, 2, 1, 5, 4}; int size = sizeof(arr) / sizeof(arr[0]); //print the original Array cout<<"Array before Arrangement: "; for (int i = 0; i < size; i++){ cout << arr[i] << " "; } //calling the function to rearrange the array Rearrangement(arr, size); //print the array after rearranging the values cout<<"\nRearrangement of an array so that arr[i] becomes arr[arr[i]] with O(1) extra space is: "; for(int i = 0; i < size; i++){ cout<< arr[i] << " "; } return 0; }
Output
If we run the above code it will generate the following output
Array before Arrangement: 0 3 2 1 5 4 Rearrangement of an array so that arr[i] becomes arr[arr[i]] with O(1) extra space is: 0 1 2 3 4 5
The above is the detailed content of Rearrange an array so that arr becomes arr] and only use O(1) extra space, implemented in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Question: Use C program to explain the concepts of post-increment and pre-increment of arrays. Solution Increment Operator (++) - There are two types of increment operators used to increase the value of a variable by 1 - pre-increment and post-increment. In prepended increment, the increment operator is placed before the operand, and the value is incremented first and then the operation is performed. eg:z=++a;a=a+1z=a The increment operator is placed after the operand in the post-increment operation, and the value will increase after the operation is completed. eg:z=a++;z=aa=a+1 Let us consider an example of accessing a specific element in a memory location by using pre-increment and post-increment. Declare an array of size 5 and perform compile-time initialization. Afterwards try assigning the pre-increment value to variable 'a'. a=++arr[1]
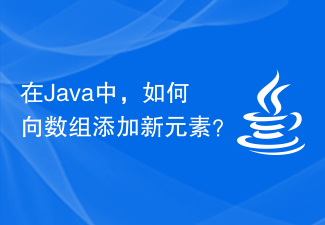
Adding new elements to an array is a common operation in Java and can be accomplished using a variety of methods. This article will introduce several common methods of adding elements to an array and provide corresponding code examples. 1. A common way to use a new array is to create a new array, copy the elements of the original array to the new array, and add new elements at the end of the new array. The specific steps are as follows: Create a new array whose size is 1 larger than the original array. This is because a new element is being added. Copy the elements of the original array to the new array. Add to the end of the new array
![Rearrange an array so that arr becomes arr] and only use O(1) extra space, implemented in C++](https://img.php.cn/upload/article/000/000/164/169319478769496.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
We get an array of positive integer type, say, arr[] of any given size, such that the element value in the array should be greater than 0 but less than the size of the array. The task is to rearrange an array only by changing arr[i] to arr[arr[i]] in the given O(1) space and print the final result. Let’s look at various input and output scenarios for this situation − Input − intarr[] = {032154} Output − Array before arrangement: 032154 Rearrange the array so that arr[i] becomes arr[arr[i]], And has O(1) extra space: 012345 Explanation − We are given an integer array of size 6, and all elements in the array have values less than 6. Now we will rearrange
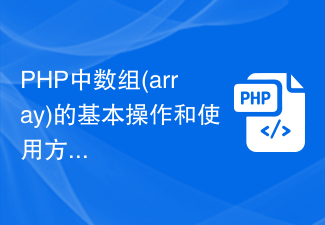
Basic operations and usage of arrays in PHP 1. Overview Array is a very important data type in PHP. It can be used to store multiple values, and these values can be accessed through indexes or keys. Arrays have rich operations and usage methods in PHP. This article will introduce in detail the basic operations and usage methods of arrays in PHP. 2. Create arrays In PHP, you can create arrays in two ways: countable arrays and associative arrays. Creating a Countable Array A countable array is an array that is arranged in order and indexed numerically
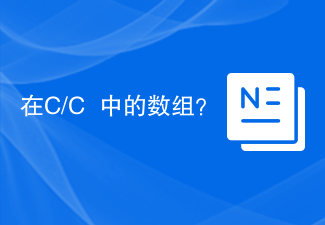
An array is a sequential collection of elements of the same type. Arrays are used to store collections of data, but it is often more useful to think of arrays as collections of variables of the same type. Instead of declaring a single variable such as number0, number1, ... and number99, you can declare an array variable (e.g. number) and represent it using numbers[0], numbers[1] and ..., numbers[99] each variable. Specific elements in the array are accessed through indexing. All arrays consist of contiguous memory locations. The lowest address corresponds to the first element, and the highest address corresponds to the last element. Declaring an ArrayDeclaring an array requires specifying the type of elements and the number of elements required. An array is as follows -ty
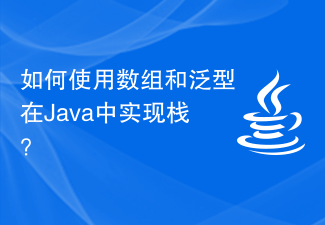
Java implements the stack by utilizing arrays and generics. This creates a versatile and reusable data structure that operates on the last-in-first-out (LIFO) principle. Following this principle, elements are added and removed from the top. By utilizing arrays as the basis, it ensures efficient memory allocation and access. Additionally, by incorporating generics, the stack is able to accommodate elements of different types, thereby enhancing its versatility. The implementation involves the definition of a Stack class containing generic type parameters. It includes basic methods such as push(), pop(), peek() and isEmpty(). Handling of edge cases, such as stack overflows and underflows, is also critical to ensure seamless functionality. This implementation enables developers to create programs that accommodate
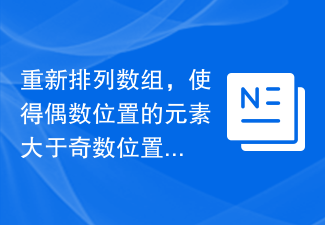
We get an array of integer type containing positive and negative numbers, say, arr[] of any given size. The task is to rearrange the array in such a way that all elements at even positions or indices should be larger than elements at odd positions or indices and print the result. Let’s look at various input and output scenarios for this - input −intarr[]={2,1,4,3,6,5,8,7} output − array before arrangement: 21436587 Rearrange the array so that the even positions are Greater than odd position: 12345678 Explanation − We get an integer array of size 8 containing positive and negative factors. Now, we rearrange the array so that all elements in even positions are larger than elements in odd positions,
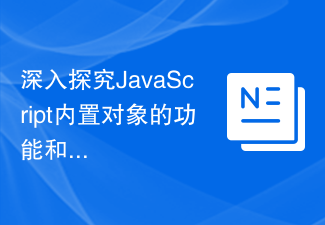
In-depth analysis of the functions and characteristics of JS built-in objects JavaScript is an object-based programming language. It provides many built-in objects with various rich functions and characteristics. In this article, we will provide an in-depth analysis of some commonly used built-in objects and give corresponding code examples. Math object The Math object provides some basic mathematical operation methods, such as exponentiation, square root, logarithm, etc. The following are some commonly used Math object method examples: //Find the absolute value Math.abs(-10
