How to draw a smooth curve through multiple points using JavaScript?
In this article, we will learn how to draw a smooth curve through multiple points using JavaScript with the help of the Canvas browser API and HTML elements.
When visualizing data on the web or creating interactive graphics, drawing smooth curves through multiple points can greatly enhance the beauty and readability of the information. Let's go through some examples to see how this can be achieved.
Example 1
In this example, we will utilize the concept of a Brazier curve defined by a set of control points to draw a smooth curve through them. We will use the canvas HTML element and its context API to predefine points through which to draw a smooth curve.
File name: index.html
<html lang="en"> <head> <title> How to Draw Smooth Curve Through Multiple Points using JavaScript? </title> <style> canvas { border: 1px solid #000; } </style> </head> <body> <canvas id="myCanvas" width="500" height="300"></canvas> <script> const canvas = document.getElementById("myCanvas"); const context = canvas.getContext("2d"); const points = [ { x: 50, y: 100 }, { x: 150, y: 200 }, { x: 250, y: 50 }, { x: 350, y: 150 }, { x: 450, y: 100 }, ]; function drawSmoothCurve(points) { context.beginPath(); context.moveTo(points[0].x, points[0].y); for (let i = 1; i < points.length - 1; i++) { const xc = (points[i].x + points[i + 1].x) / 2; const yc = (points[i].y + points[i + 1].y) / 2; context.quadraticCurveTo(points[i].x, points[i].y, xc, yc); } // Connect the last two points with a straight line context.lineTo(points[points.length - 1].x, points[points.length - 1].y); context.stroke(); } drawSmoothCurve(points); </script> </body> </html>
Example 2
In this example, we will follow the code structure above and draw a smooth curve through multiple points using the Bézier curve and Catmull-Rom spline methods.
File name: index.html
<html lang="en"> <head> <title>How to Draw Smooth Curve Through Multiple Points using JavaScript?</title> <style> canvas { border: 1px solid #000; } </style> </head> <body> <canvas id="myCanvas" width="500" height="300"></canvas> <script> const canvas = document.getElementById("myCanvas"); const context = canvas.getContext("2d"); const points = [ { x: 50, y: 100 }, { x: 150, y: 200 }, { x: 250, y: 50 }, { x: 350, y: 150 }, { x: 450, y: 100 }, ]; function drawSmoothCurve(points) { context.beginPath(); context.moveTo(points[0].x, points[0].y); // Example 1: Bézier Curves // context.quadraticCurveTo(cp1x, cp1y, x, y); // context.bezierCurveTo(cp1x, cp1y, cp2x, cp2y, x, y); for (let i = 1; i < points.length - 1; i++) { const xc = (points[i].x + points[i + 1].x) / 2; const yc = (points[i].y + points[i + 1].y) / 2; context.quadraticCurveTo(points[i].x, points[i].y, xc, yc); } // Connect the last two points with a straight line context.lineTo(points[points.length - 1].x, points[points.length - 1].y); context.stroke(); } drawSmoothCurve(points); // Example 2: Catmull-Rom Splines function catmullRomSpline(points, context) { context.beginPath(); context.moveTo(points[0].x, points[0].y); for (let i = 1; i < points.length - 2; i++) { const p0 = points[i - 1]; const p1 = points[i]; const p2 = points[i + 1]; const p3 = points[i + 2]; const t = 0.5; const x1 = (-t * p0.x + (2 - t) * p1.x + (t - 2) * p2.x + t * p3.x) / 2; const y1 = (-t * p0.y + (2 - t) * p1.y + (t - 2) * p2.y + t * p3.y) / 2; const x2 = ((2 * t - 3) * p0.x + (3 - 4 * t) * p1.x + (1 + 2 * t) * p2.x + (-t) * p3.x) / 2; const y2 = ((2 * t - 3) * p0.y + (3 - 4 * t) * p1.y + (1 + 2 * t) * p2.y + (-t) * p3.y) / 2; const x3 = (t * p1.x + (2 - t) * p2.x) / 2; const y3 = (t * p1.y + (2 - t) * p2.y) / 2; context.bezierCurveTo(x1, y1, x2, y2, x3, y3); } context.lineTo(points[points.length - 2].x, points[points.length - 2].y); context.lineTo(points[points.length - 1].x, points[points.length - 1].y); context.stroke(); } catmullRomSpline(points, context); </script> </body> </html>
in conclusion
In summary, using JavaScript to draw smooth curves through multiple points can greatly enhance the visual beauty and readability of web-based graphs and data visualizations. By leveraging the power of Bezier curves and Catmull-Rom splines, we learned how to draw a smooth curve through multiple points using JavaScript with the help of the canvas HTML element and its context API.
The above is the detailed content of How to draw a smooth curve through multiple points using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
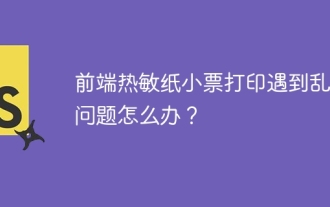
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
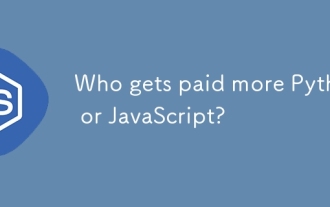
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
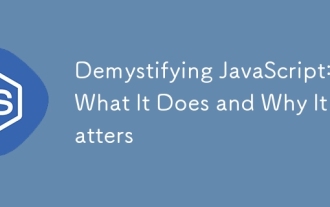
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
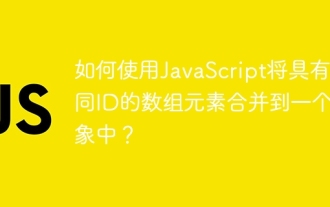
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
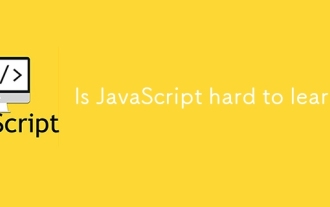
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
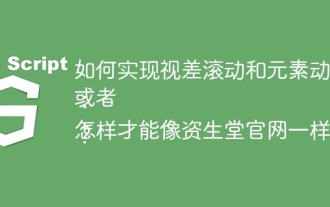
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
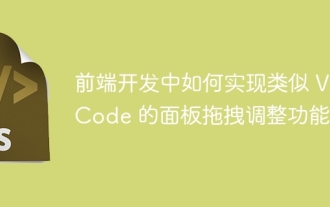
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
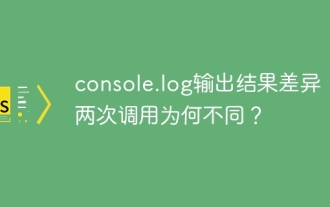
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
