Which array element has the smallest sum of absolute differences?
Here, we will see an interesting question. We have an array 'a' containing N elements. We need to find an element x that minimizes the value of |a[0] - x| |a[1] - x| ... |a[n-1] - x|. Then we need to find the minimized sum.
Suppose the array is: {1, 3, 9, 6, 3}, and now x is 3. So the sum is |1 - 3| |3 - 3| |9 - 3| |6 - 3| |3 - 3| = 11.
To solve this problem, we need to choose the median of the array as x. If the size of the array is even, there will be two median values. They are all the best choices for x.
Algorithm
minSum(arr, n)
begin sort array arr sum := 0 med := median of arr for each element e in arr, do sum := sum + |e - med| done return sum end
Example
#include <iostream> #include <algorithm> #include <cmath> using namespace std; int minSum(int arr[], int n){ sort(arr, arr + n); int sum = 0; int med = arr[n/2]; for(int i = 0; i<n; i++){ sum += abs(arr[i] - med); } return sum; } int main() { int arr[5] = {1, 3, 9, 6, 3}; int n = 5; cout << "Sum : " << minSum(arr, n); }
Output
Sum : 11
The above is the detailed content of Which array element has the smallest sum of absolute differences?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
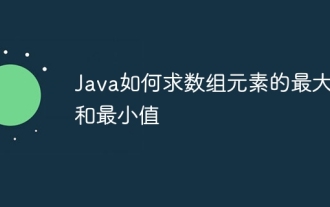
Use the `Arrays.stream()` function in Java to convert an array into a stream, and then use the `min()` and `max()` functions to calculate the minimum and maximum values.
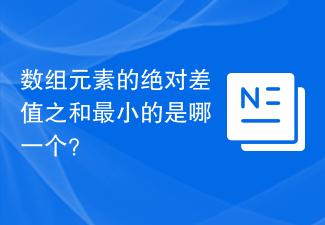
Here we will see an interesting problem. We have an array 'a' containing N elements. We need to find an element x that minimizes the value of |a[0]-x|+|a[1]-x|+...+|a[n-1]-x|. Then we need to find the minimized sum. Suppose the array is: {1,3,9,6,3}, and now x is 3. So the sum is |1-3|+|3-3|+|9-3|+|6-3|+|3-3|=11. To solve this problem, we need to choose the median of the array as x. If the size of the array is even, there will be two median values. They are all the best choices for x. Algorithm minSum(arr,n)begin &
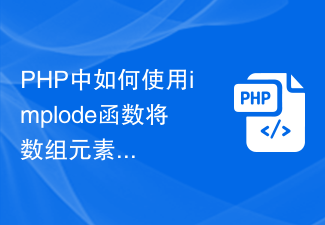
In PHP programming, the implode function is a very commonly used function that can concatenate elements in an array into a string. Using this function can save developers from writing a lot of code to connect strings, making it more efficient. The basic syntax of implode is: stringimplode(string$glue,array$pieces). This function receives two parameters: $glue represents the separator to connect the array elements, and $pieces represents
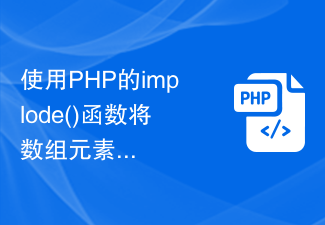
Use PHP's implode() function to connect array elements into a delimited string. The code example is as follows: <?php//Define an array $array=array('apple','banana','orange'); //Use the implode() function to connect the array elements into a delimited string $delimiter=',';//Define the delimiter $result=im
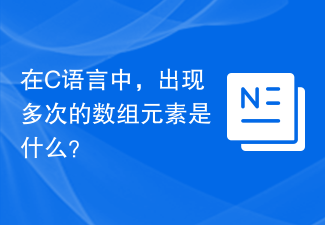
Array is a container that contains elements of the same data type, and the length needs to be defined in advance. Elements in an array can appear in any order and any number of times. So, in this program, we will find the elements that appear multiple times in an array. Problem description - We have been given an array arr[], we need to find the recurring elements in the array and print them. Let us take an example to understand better. Example: Input:arr[]={5,11,11,2,1,4,2}Output:112 Explanation We have an array arr containing some elements, first we will compare the next element in the repeat function. Repeat function is used to find duplicate elements in an array. In the repeat function we use
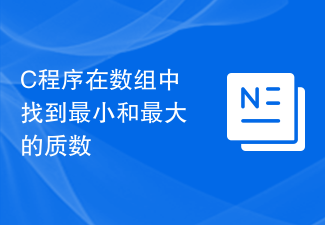
Problem StatementGiven an array containing n positive integers. We have to find the number where the prime numbers have minimum and maximum values. If the given array is -arr[]={10,4,1,12,13,7,6,2,27,33}thenminimumprimenumberis2andmaximumprimenumberis13 Algorithm 1.Findmaximumnumberfromgivennumber.LetuscallitmaxNumber2.Generateprimenumbersfrom1tomaxNumberandstoretheminadynamicar
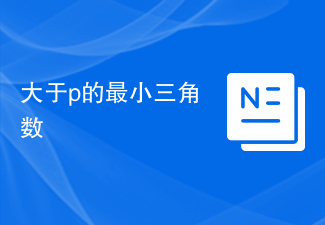
We will discuss triangle numbers and how to find the smallest triangle number that is only greater than the given number "num". We will first discuss what a trigonometric number is and then find the smallest trigonometric number that is greater than "num" We will see two different approaches to the same problem. In the first method we will run a simple loop to generate the output, while in the second method we will first generate a general formula for calculating the required number and then directly apply that formula to get the minimum Triangle number. Problem Statement We have to find the smallest number of triangles that is only greater than "num". We have multiple boxes with balls in them. The number of balls the box contains is a different triangular number for all boxes. The boxes are numbered from 1 to n. we have to find out from the box
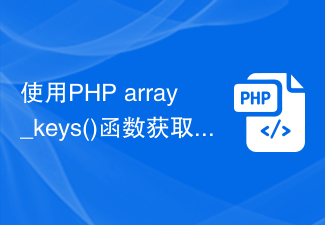
In the process of using PHP to develop, it is often necessary to operate arrays. In an array, we usually need to get the key value of the element to facilitate subsequent operations. For this purpose, PHP provides a very convenient function array_keys(), which can quickly obtain the keys of elements from an array. The usage of the array_keys() function is very simple. Its basic syntax is as follows: arrayarray_keys(array$array[,mixed$search_valu
