C program to calculate distance between three points in 3D space
Given a three-dimensional plane and therefore three coordinates, the task is to find the distance between the given points and display the result.
On the three-dimensional plane, there are three coordinate axes. The coordinates of the x-axis are (x1, y1, z1), the coordinates of the y-axis are (x2, y2, z2), and the coordinates of the z-axis are (x3, y3,z). There is a direct formula for calculating the distance between them as follows
$$\sqrt{\lgroup x2-x1\rgroup^{2} \lgroup y2-y1\rgroup^{2} \lgroup z2 -z1\rgroup^{2}}$$
The following is a diagram showing three different axes and their coordinates
The method used below is as follows −
- Input coordinates (x1, y1, z1), (x2, y2, z2) and (x3, y3, z3)
- Apply the formula to calculate the difference between these points
- Print distance
Algorithm
Start Step 1-> declare function to calculate distance between three point void three_dis(float x1, float y1, float z1, float x2, float y2, float z2) set float dis = sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2) + pow(z2 - z1, 2) * 1.0) print dis step 2-> In main() Set float x1 = 4 Set float y1 = 9 Set float z1 = -3 Set float x2 = 5 Set float y2 = 10 Set float z2 = 9 Call three_dis(x1, y1, z1, x2, y2, z2) Stop
Example
's translation is:Example
#include <stdio.h> #include<math.h> //function to find distance bewteen 3 point void three_dis(float x1, float y1, float z1, float x2, float y2, float z2) { float dis = sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2) + pow(z2 - z1, 2) * 1.0); printf("Distance between 3 points are : %f", dis); return; } int main() { float x1 = 4; float y1 = 9; float z1 = -3; float x2 = 5; float y2 = 10; float z2 = 9; three_dis(x1, y1, z1, x2, y2, z2); return 0; }
Output
If we run the above code it will generate the following output
Distance between 3 points are : 12.083046
The above is the detailed content of C program to calculate distance between three points in 3D space. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


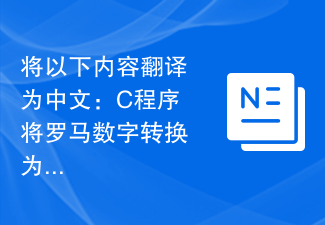
Given below is a C language algorithm to convert Roman numerals to decimal numbers: Algorithm Step 1 - Start Step 2 - Read Roman numerals at runtime Step 3 - Length: = strlen(roman) Step 4 - For i=0 to Length-1 Step 4.1-switch(roman[i]) Step 4.1.1-case'm': &nbs
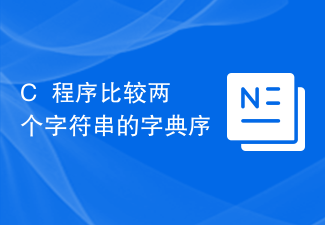
Lexicographic string comparison means that strings are compared in dictionary order. For example, if there are two strings 'apple' and 'appeal', the first string will come last because the first three characters of 'app' are the same. Then for the first string the character is 'l' and in the second string the fourth character is 'e'. Since 'e' is shorter than 'l', it will come first if we sort lexicographically. Strings are compared lexicographically before being arranged. In this article, we will see different techniques for lexicographically comparing two strings using C++. Using the compare() function in C++ strings The C++string object has a compare()
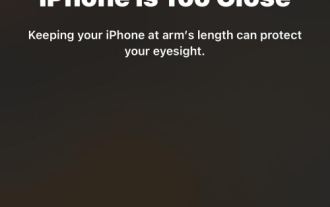
What is the screen distance feature in iOS17 update? Apple provides a screen distance function on the iPhone to help users prevent eye fatigue and the risk of myopia. The feature (available on iOS 17 or later) will use the iPhone's TrueDepth camera (the same camera that also helps FaceID) to measure the distance between the face and the phone. If Screen Distance detects that you are holding your iPhone closer than 12 inches or 30 centimeters for an extended period of time, it will prompt you to maintain distance from the iPhone screen. When your device detects that it is less than 12 inches from your face, you will see the "iPhone too close" message on the screen and advise that you should keep your distance to protect your vision. Only if you place the device further
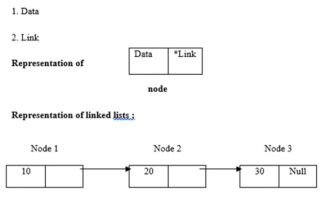
Linked lists use dynamic memory allocation, i.e. they grow and shrink accordingly. They are defined as collections of nodes. Here, a node has two parts, data and links. The representation of data, links and linked lists is as follows - Types of linked lists There are four types of linked lists, as follows: - Single linked list/Singly linked list Double/Doubly linked list Circular single linked list Circular double linked list We use the recursive method to find the length of the linked list The logic is -intlength(node *temp){ if(temp==NULL) returnl; else{&n
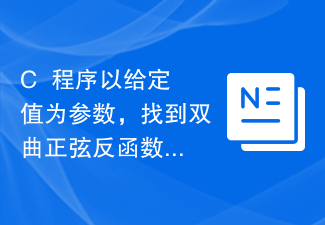
Hyperbolic functions are defined using hyperbolas instead of circles and are equivalent to ordinary trigonometric functions. It returns the ratio parameter in the hyperbolic sine function from the supplied angle in radians. But do the opposite, or in other words. If we want to calculate an angle from a hyperbolic sine, we need an inverse hyperbolic trigonometric operation like the hyperbolic inverse sine operation. This course will demonstrate how to use the hyperbolic inverse sine (asinh) function in C++ to calculate angles using the hyperbolic sine value in radians. The hyperbolic arcsine operation follows the following formula -$$\mathrm{sinh^{-1}x\:=\:In(x\:+\:\sqrt{x^2\:+\:1})}, Where\:In\:is\:natural logarithm\:(log_e\:k)
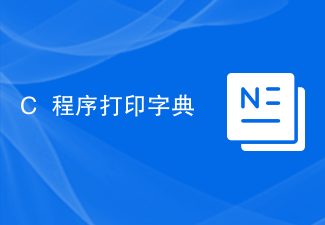
A map is a special type of container in C++ in which each element is a pair of two values, namely a key value and a map value. The key value is used to index each item, and the mapped value is the value associated with the key. Regardless of whether the mapped value is unique, the key is always unique. To print map elements in C++ we have to use iterator. An element in a set of items is indicated by an iterator object. Iterators are primarily used with arrays and other types of containers (such as vectors), and they have a specific set of operations that can be used to identify specific elements within a specific range. Iterators can be incremented or decremented to reference different elements present in a range or container. The iterator points to the memory location of a specific element in the range. Printing a map in C++ using iterators First, let's look at how to define
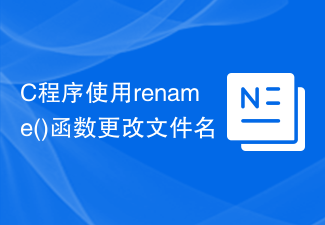
The rename function changes a file or directory from its old name to its new name. This operation is similar to the move operation. So we can also use this rename function to move files. This function exists in the stdio.h library header file. The syntax of the rename function is as follows: intrename(constchar*oldname,constchar*newname); The function of the rename() function accepts two parameters. One is oldname and the other is newname. Both parameters are pointers to constant characters that define the old and new names of the file. Returns zero if the file was renamed successfully; otherwise, returns a nonzero integer. During a rename operation
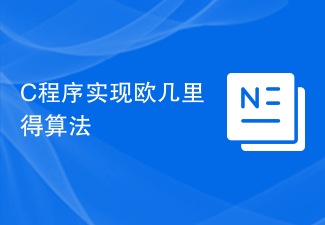
The problem implements Euclidean's algorithm to find the greatest common divisor (GCD) and least common multiple (LCM) of two integers and outputs the results with a given integer. Solution The solution to implement Euclidean algorithm to find the greatest common divisor (GCD) and least common multiple (LCM) of two integers is as follows - the logic of finding GCD and LCM is as follows - if (firstno*secondno!=0){ gcd= gcd_rec(firstno,secondno); printf("TheGCDof%dand%dis%d",
