Centralized and client-server architecture of DBMS
Introduction
A database management system (DBMS) is a software system designed to manage and organize data in a structured manner. To achieve this goal, DBMS uses a specific architecture to specify how data is stored, retrieved, and updated. In DBMS, the two most commonly used architectures are centralized architecture and client-server architecture.
Centralized Architecture
The architecture of a centralized database management system (DBMS) means that all data is stored on a single server, and all clients connect to the server to access and manipulate the data. This architecture is also called a monolithic architecture. One of the main advantages of a centralized architecture is its simplicity - there is only one server to manage, and all clients work with the same data.
However, this type of architecture also has some disadvantages. One of the main disadvantages is that since all data is stored on a single server, that server can become a bottleneck as the number of clients and/or data volume increases. Additionally, if the server goes down for any reason, all clients lose access to the data.
An example of a DBMS that uses a centralized architecture is SQLite, which is an open source, independent, high-reliability, embedded, full-featured public domain SQL database engine. SQLite's architecture is based on a client-server model, but the entire database is contained in a single file, making it ideal for small to medium-sized applications.
Example
import sqlite3 #connect to the database conn = sqlite3.connect('example.db') #create a cursor object cursor = conn.cursor() #create a table cursor.execute('''CREATE TABLE employees (id INT PRIMARY KEY NOT NULL, name TEXT NOT NULL, salary REAL);''') #commit the changes conn.commit() #close the connection conn.close()
Explanation
is:Explanation
In the above example, we import the sqlite3 module, connect to the database named "example.db", create a cursor object, and then use the cursor to create a table named "employees" with three columns: "id", "name" and "salary". The table is defined with an INT data type for the "id" column (also set as primary key and NOT NULL), a TEXT data type for the "name" column, and a REAL data type for the "salary" column. After creating the table, we use the "commit" method to save the changes and the "close" method to close the connection.
Client-Server Architecture
The client-server architecture of DBMS is an architecture in which data is stored on a central server, but clients connect to the server to access and manipulate the data. This architecture is more complex than a centralized architecture, but it offers several advantages over the latter.
One of the main advantages of client-server architecture is that it is more scalable than centralized architecture. As the number of clients and/or data volume increases, servers can be upgraded or additional servers added to handle the load. This allows the system to continue to run smoothly even as it scales up.
Another advantage of client-server architecture is that it is more fault tolerant than centralized architecture. If one server goes down, other servers can take over its duties and clients can still access data. This makes the system less likely to experience downtime, a critical factor in many business environments.
An example of a database management system that uses a client-server architecture is MySQL, which is an open source relational database management system. MySQL uses a multi-threaded architecture, and multiple clients can connect to the server and make requests at the same time. The server processes these requests and returns the results to the appropriate client.
Example
import mysql.connector #connect to the database cnx = mysql.connector.connect(user='username', password='password', host='hostname', database='database_name') #create a cursor object cursor = cnx.cursor() #create a table cursor.execute('''CREATE TABLE employees (id INT PRIMARY KEY NOT NULL, name VARCHAR(255) NOT NULL, salary DECIMAL(10,2));''') #commit the changes cnx.commit() #close the connection cnx.close()
Explanation
is:Explanation
In the above example, we import the mysql.connector module, use the "connect" method to connect to the database, and pass in the necessary parameters, such as username, password, hostname, and database name. We create a cursor object and use the cursor to create a table called "employees" with three columns: "id", "name", and "salary".
The "id" column of this table is defined as the INT data type, which is also set as the primary key and NOT NULL, the "name" column is defined as the VARCHAR data type, and the "salary" column is defined as the DECIMAL data type. After creating the table, we use the "commit" method to save the changes and the "close" method to close the connection.
Fragmentation
Sharding is a method of distributing large databases across multiple servers. This approach is often used in client-server architectures to improve performance and scalability. Data is split into smaller chunks called shards and then distributed across multiple servers.
Each shard is an independent subset of data, and clients can connect to any server to access the data they need. This approach allows for horizontal scalability, meaning that as the amount of data or the number of clients increases, more servers can be added to the system to handle the load.
copy
Replication is a method of maintaining multiple copies of a database on different servers. This approach is often used in client-server architectures to improve fault tolerance and performance. There are several types of replication, including master-slave replication, where one server acts as the master and the other servers act as slaves, and all changes made on the master are replicated to the slaves.
Another type of replication is called master-master replication, where multiple servers can act as masters and slaves, allowing data to be written to any server and changes replicated to all other servers.
cache
Caching is a method of storing frequently accessed data in memory to improve access speed. This approach is often used in centralized and client-server architectures to improve performance. When a client requests data from the server, the server first checks whether the data is already in the cache.
If so, the server returns the data from the cache, which is faster than retrieving the data from the main data store. The cache can also be used to temporarily store data about to be written to the main data store, which helps reduce the load on the server and improves write performance.
Load Balancing
Load balancing is a method of distributing load among multiple servers. This approach is often used in client-server architectures to improve performance and scalability. A load balancer is usually placed in front of a group of servers and is responsible for distributing incoming requests to different servers.
This can be achieved in a variety of ways, such as polling or a minimum number of connections, and the goal is to ensure that all servers are used as efficiently as possible. Load balancing also helps improve fault tolerance because if one server goes down, the load balancer can redirect traffic to other servers to keep the system running smoothly.
These are just some examples of how different techniques and methods can be used to improve the performance, scalability, and availability of database systems. It is important to remember that the architecture of a database system is critical to ensuring that it meets the performance and scalability requirements of the system. Identifying the right architecture and implementing it according to best practices will be critical to the success of your DBMS.
in conclusion
Both centralized and client-server architectures of DBMS have their own advantages and disadvantages, and the choice of architecture will depend on the specific needs of the application. Centralized architectures are simpler and easier to manage, but they can become bottlenecks as systems grow in size. Client-server architectures are more complex, but they are more scalable and fault-tolerant, making them a better choice for larger, more critical systems.
Speaking of code examples, specific DBMSs also have their own syntax and structure, which are not exactly the same, but it can give you a general understanding of how to connect and create tables in a DBMS. Be sure to consult the documentation for the specific DBMS you are using and test your code before deploying it to a production environment.
The above is the detailed content of Centralized and client-server architecture of DBMS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


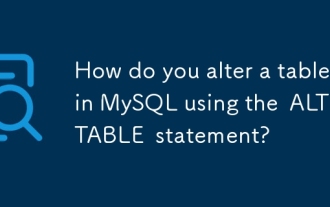
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
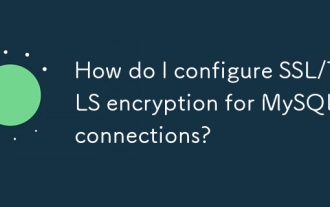
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
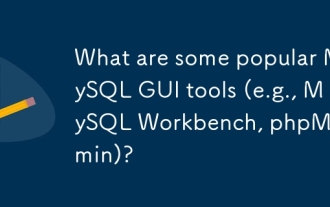
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
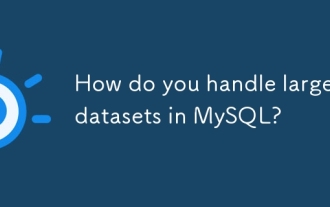
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
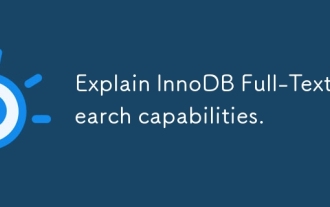
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
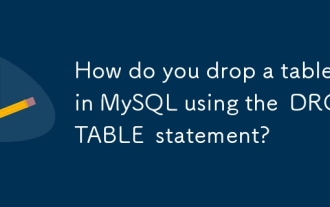
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
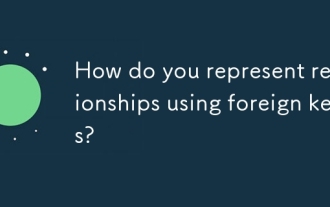
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
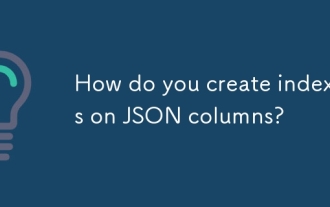
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
