How to create a constant in Python?
Constants and variables are used to store data values in programming. A variable usually refers to a value that can change over time. A constant is a type of variable whose value cannot be changed during program execution.
There are only six built-in constants available in Python, they are False, True, None, Not Implemented, Ellipsis(...) and __debug__. Apart from these constants, Python does not have any built-in data types to store constant values.
Example
The following demonstrates an example of constants -
1 |
|
Output
1 |
|
False is a built-in constant in Python, used to store the Boolean value false. Assigning any value to it is illegal and will raise a SyntaxError.
But in the PEP 8 standard, constants are in uppercase. This helps the user know that it is a constant value. If we encounter any all-caps variables, by convention rather than rule, we should not change their values. Let's look at an example.
Example
π is a mathematical constant, approximately equal to 3.14159. Let us declare the value of the constant π in Python.
1 2 3 |
|
Output
1 |
|
In the example above, the mathematical constant pi is declared using all uppercase letters.
Example
As mentioned in the Constants section of PEP 8, we should use uppercase letters and underscores to separate words.
1 2 3 4 5 6 7 8 |
|
Output
1 2 3 |
|
As we can see, constants are also created exactly like variables. Both variables and constants follow similar naming rules, the only difference is that constants only use uppercase letters.
Example
Normally, in Python, constants are declared in modules. Let's take an example and create constants.
Declare constants in a separate file and name the file with a .py extension.
Constants.py file
1 2 3 4 5 6 |
|
Example.py file
1 2 3 4 5 |
|
Output
1 2 3 |
|
In the above example, we created the Constants.py file, called the Constants module. Then, we declared some constant values. After that, we create another python file which is an Example.py file and in this file we import the Constant module using the import keyword. Finally, access the constant value.
The purpose of using uppercase letters is to indicate that the current name is considered a constant. But it doesn't actually prevent the reallocation of constant values.
The above is the detailed content of How to create a constant in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
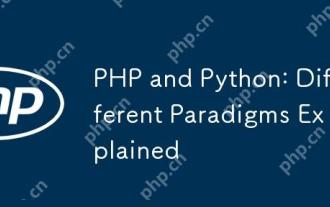
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
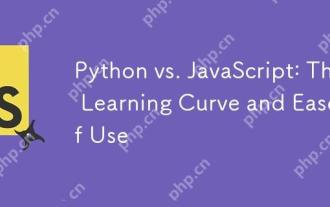
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
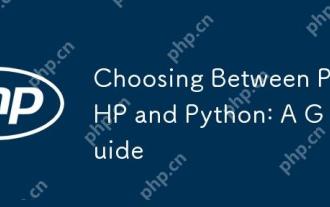
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
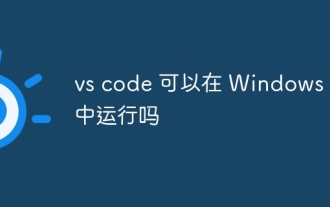
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
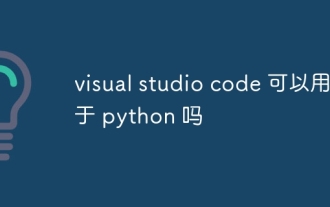
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
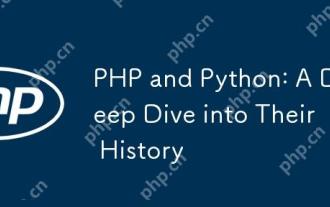
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
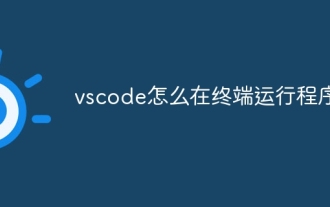
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
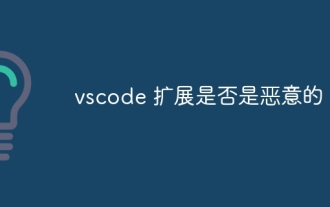
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
