Write a C program to display the size and offset of structure members
Question
Write a C program to define a structure and display the size and offset of member variables
Structure Body - It is a collection of variables of different data types, grouped under one name.
General form of structure declaration
datatype member1; struct tagname{ datatype member2; datatype member n; };
Here, struct - keyword
tagname - specifies the name of the structure
member1, member2 - specifies the constituent structure data items.
Example
struct book{ int pages; char author [30]; float price; };
Structure variables
There are three ways to declare structure variables-
Method 1
struct book{ int pages; char author[30]; float price; }b;
Method 2
struct{ int pages; char author[30]; float price; }b;
Method 3
struct book{ int pages; char author[30]; float price; }; struct book b;
Initializing and accessing the structure
The link between members and structure variables is established through the member operator (or dot operator).
Can be initialized in the following ways:
Method 1
struct book{ int pages; char author[30]; float price; } b = {100, "balu", 325.75};
Method 2
struct book{ int pages; char author[30]; float price; }; struct book b = {100, "balu", 325.75};
Method 3 (using member operator)
struct book{ int pages; char author[30]; float price; } ; struct book b; b. pages = 100; strcpy (b.author, "balu"); b.price = 325.75;
Method 4 (using scanf function)
struct book{ int pages; char author[30]; float price; } ; struct book b; scanf ("%d", &b.pages); scanf ("%s", b.author); scanf ("%f", &b. price);
Declare the structure using data members and try to print their offset values and the size of the structure.
Program
Real-time demonstration
#include<stdio.h> #include<stddef.h> struct tutorial{ int a; int b; char c[4]; float d; double e; }; int main(){ struct tutorial t1; printf("the size 'a' is :%d</p><p>",sizeof(t1.a)); printf("the size 'b' is :%d</p><p>",sizeof(t1.b)); printf("the size 'c' is :%d</p><p>",sizeof(t1.c)); printf("the size 'd' is :%d</p><p>",sizeof(t1.d)); printf("the size 'e' is :%d</p><p>",sizeof(t1.e)); printf("the offset 'a' is :%d</p><p>",offsetof(struct tutorial,a)); printf("the offset 'b' is :%d</p><p>",offsetof(struct tutorial,b)); printf("the offset 'c' is :%d</p><p>",offsetof(struct tutorial,c)); printf("the offset 'd' is :%d</p><p>",offsetof(struct tutorial,d)); printf("the offset 'e' is :%d</p><p></p><p>",offsetof(struct tutorial,e)); printf("size of the structure tutorial is :%d",sizeof(t1)); return 0; }
Output
the size 'a' is :4 the size 'b' is :4 the size 'c' is :4 the size 'd' is :4 the size 'e' is :8 the offset 'a' is :0 the offset 'b' is :4 the offset 'c' is :8 the offset 'd' is :12 the offset 'e' is :16 size of the structure tutorial is :24
The above is the detailed content of Write a C program to display the size and offset of structure members. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


![How to increase disk size in VirtualBox [Guide]](https://img.php.cn/upload/article/000/887/227/171064142025068.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
We often encounter situations where the predefined disk size has no room for more data? If you need more virtual machine hard disk space at a later stage, you must expand the virtual hard disk and partitions. In this post, we will see how to increase disk size in VirtualBox. Increasing the disk size in VirtualBox It is important to note that you may want to back up your virtual hard disk files before performing these operations, as there is always the possibility of something going wrong. It is always a good practice to have backups. However, the process usually works fine, just make sure to shut down your machine before continuing. There are two ways to increase disk size in VirtualBox. Expand VirtualBox disk size using GUI using CL

In C, both structures and arrays are used as containers of data types, that is, in both structures and arrays we can store data and perform different operations on them. Based on the internal implementation, here are some basic differences between the two. Sr. Number Key Structure Array 1 Definition A structure can be defined as a data structure that is used as a container and can hold variables of different types. Array, on the other hand, is a data structure used as a container that can hold variables of the same type but does not support multiple data type variables. 2 Memory Allocation Memory allocation structures for input data do not have to be in contiguous memory locations. While in the case of arrays, the input data is stored in contiguous memory allocation, which means that arrays store data in a memory model that allocates contiguous memory blocks (i.e., has
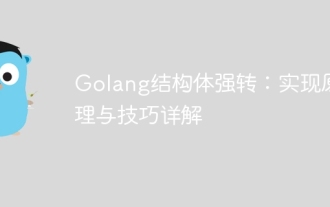
Struct coercion in Golang is to convert the value of one structure type to another type. This can be achieved through techniques such as assertion force transfer, reflection force transfer, and pointer indirect force transfer. Assertion coercion uses type assertions, reflective coercion uses the reflection mechanism, and pointer indirect coercion avoids value copying. The specific steps are: 1. Assertion force transfer: use typeassertion syntax; 2. Reflection force transfer: use reflect.Type.AssignableTo and reflect.Value.Convert functions; 3. Pointer indirect force transfer: use pointer dereference.
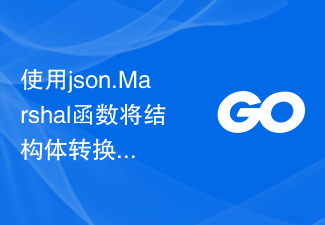
Use the json.Marshal function to convert a structure into a JSON string. In the Go language, you can use the json.Marshal function to convert a structure into a JSON string. A structure is a data type composed of multiple fields, and JSON is a commonly used lightweight data exchange format. Converting structures to JSON strings makes it easy to exchange data between different systems. Here is a sample code: packagemainimport(&q
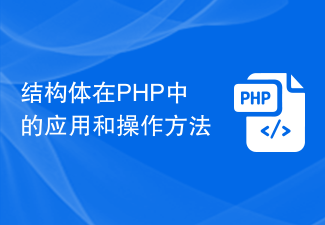
As the PHP language continues to develop and grow, the application and operation methods of structures in PHP are becoming increasingly complete. In addition to common variables and arrays, PHP also provides a more flexible data type, namely structure. A structure is a composite data type composed of multiple data members of different types. It can combine related data to form a more complete and structured data. In PHP, you can simulate the behavior and functionality of structures by using classes and objects. First, let's look at how
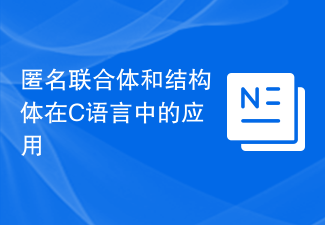
Here we take a look at what are anonymous unions and structures in C language. Anonymous unions and structures are unnamed unions and structures. Since they have no name, we cannot create a direct object of it. We use it as a nested structure or union. These are examples of anonymous unions and structures. struct{ datatypevariable; ...};union{ datatypevariable; ...};In this example, we are creating

How to return struct in Golang? Specify the structure type in the function signature, such as: funcgetPerson()Person{}. Use the return{} statement in the function body to return a structure containing the required fields. Struct fields can be base types or other structures.
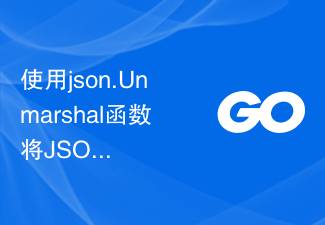
Use the json.Unmarshal function to parse a JSON string into a structure. In the Go language, you can use the json.Unmarshal function to parse a JSON string into a structure. This is a very useful feature, especially when processing API responses or reading configuration files. First, we need to define a structure type to represent the structure of the JSON object we want to parse. Suppose we have the following JSON string: {"name"
