


Translate the following into Chinese: C++ Query to answer the number of 1's and 0's to the left of a given index
Discuss a question to answer a query on a given array. For example, for each query index, we need to find the number of 1's and 0's to the left of the index.
Input: arr[ ] = { 0, 1, 1, 1, 0, 0, 0, 1, 0, 0}, queries[ ] = { 2, 4, 1, 0, 5 } Output: query 1: zeros = 1,ones = 1 query 2: zeros = 1,ones = 3 query 3: zeros = 1,ones = 0 query 4: zeros = 0,ones = 0 query 5: zeros = 2,ones = 3 Input: arr[ ] = { 0, 0, 1, 1, 1, 0, 1, 0, 0, 1 }, queries[ ] = { 3, 2, 6 } Output: query 1: zeros = 2,ones = 1 query 2: zeros = 2,ones = 0 query 3: zeros = 3,ones = 3
Ways to find a solution
Naive way
A simple way to solve this problem is to iterate through the array to the index of the query and check each element; if it is 0, Then increment the zero counter by 1, otherwise increment the zero counter by 1.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int nums[] = {1, 0, 0, 1, 1, 0, 0, 1, 0, 0}; int queries[] = { 2, 4, 1, 0, 5 }; int qsize = sizeof(queries) / sizeof(queries[0]); int zeros=0,ones=0; // loop for running each query. for(int i = 0;i<qsize;i++){ //counting zeros and ones for(int j = 0;j<queries[i];j++){ if(nums[j]==0) zeros++; else ones++; } cout << "\nquery " << i+1 << ": zeros = " << zeros << ",ones = " << ones; zeros=0; ones=0; } return 0; }
Output
query 1: zeros = 1,ones = 1 query 2: zeros = 2,ones = 2 query 3: zeros = 0,ones = 1 query 4: zeros = 0,ones = 0 query 5: zeros = 2,ones = 3
Efficient method
In the previous method, every time we start from the 0th index to calculate the new query 1 and 0.
Another way is to count 0 and 1 first. appears to the left of each index, stores them in an array, and returns the answer based on the index written in the query.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int nums[] = {1, 0, 0, 1, 1, 0, 0, 1, 0, 0}; int queries[] = { 2, 4, 1, 0, 5 }; int n = sizeof(nums) / sizeof(nums[0]); int arr[n][2]; int zeros = 0, ones = 0; // traverse through the nums array. for (int i = 0; i < n; i++) { // store the number of zeros and ones in arr. arr[i][0] = zeros; arr[i][1] = ones; // increment variable according to condition if (nums[i]==0) zeros++; else ones++; } int qsize = sizeof(queries) / sizeof(queries[0]); for (int i = 0; i < qsize; i++) cout << "\nquery " << i+1 << ": zeros = " << arr[queries[i]][0] << ",ones =" << arr[queries[i]][1]; return 0; }
Output
query 1: zeros = 1,ones =1 query 2: zeros = 2,ones =2 query 3: zeros = 0,ones =1 query 4: zeros = 0,ones =0 query 5: zeros = 2,ones =3
Conclusion
In this tutorial we discussed about returning the index left for every query in a given array The number of 1's and 0's. We discussed simple and effective ways to solve this problem. We also discussed a C program to solve this problem and we can implement it using programming languages like C, Java, Python etc. We hope you found this tutorial helpful.
The above is the detailed content of Translate the following into Chinese: C++ Query to answer the number of 1's and 0's to the left of a given index. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


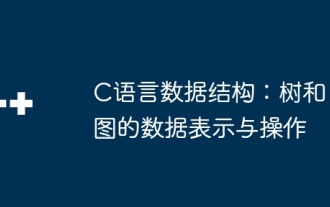
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
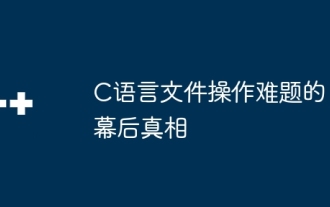
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
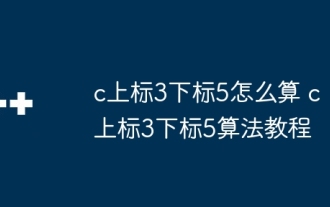
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
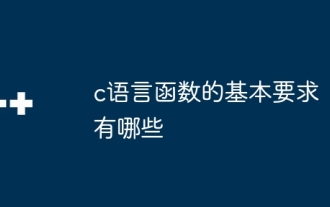
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
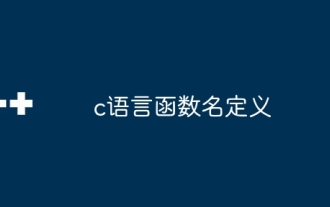
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
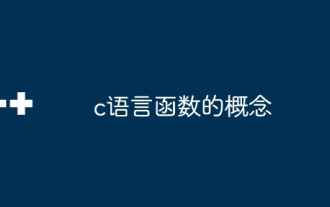
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
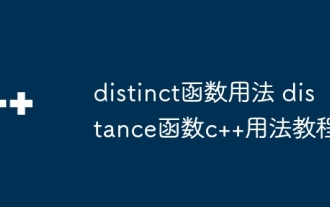
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
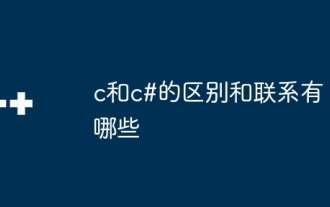
Although C and C# have similarities, they are completely different: C is a process-oriented, manual memory management, and platform-dependent language used for system programming; C# is an object-oriented, garbage collection, and platform-independent language used for desktop, web application and game development.
