Pattern classes in Java
The Pattern class represents a compiled version of a regular expression pattern. The Pattern class is located in the java.util.regex package. This class has various methods for various operations such as matching, splitting, searching, etc. In order to create a schema object, use the compile method.
grammar
public static Pattern compile(String regex)
The regex here represents a regular expression, which is a string. To compile it we use this method. Additionally, this compiled object can be used to match patterns using the Matcher method.
algorithm
To compile and match patterns, follow these steps -
Step 1 - Initialize the regular expression pattern with a string.
Step 2 - Now, compile the schema using the compile method.
Step 3 - Define the input string to match the pattern.
Step 4 - Create a Matcher object and apply the pattern to the input string.
Step 5 − Use the Matcher method to perform various operations
grammar
public class Regex { public static void main(String[] args) { String pattern = "String1"; Pattern compiledPattern = Pattern.compile(pattern); String input = "Strin2"; Matcher matcher = compiledPattern.matcher(input); if (matcher.find()) { System.out.println("Match found: " + matcher.group(0)); System.out.println("Captured group: " + matcher.group(1)); } else { System.out.println("No match found."); } } }
Method 1: Use matches() method
This method involves using the matches() method.
Example
import java.util.regex.Pattern; public class MatchThePattern { public static void main(String[] args) { String pattern = "Hello (\w+)"; String input = "Hello World"; // Add the input string to be matched boolean letsMatch = Pattern.matches(pattern, input); if (letsMatch) { System.out.println("Pattern matched."); } else { System.out.println("Pattern not matched."); } } }
Output
Pattern matched.
illustrate
By passing two string inputs as parameters to the matches method, we can successfully match the two string patterns in the above code.
Method 2: Use find() method
The find() method returns a Boolean type and finds expressions that match the pattern. The following is a code example -
Example
In this example, we will use the find() method to demonstrate the second method.
import java.util.regex.Matcher; import java.util.regex.Pattern; public class LetsFindPattern { public static void main(String[] args) { String pattern = "\b\d+\b"; String input = "The price is 100 dollars for 3 items."; Pattern compiledPattern = Pattern.compile(pattern); Matcher matcher = compiledPattern.matcher(input); while (matcher.find()) { System.out.println("Match found: " + matcher.group()); } } }
Output
Match found: 100 Match found: 3
Comparison between Method 1 and Method 2
standard |
method 1 |
Method Two |
---|---|---|
type |
String |
String |
method |
Boolean matching (string regular expression) |
Boolean search() |
Method logic |
If the match is successful, return the pattern |
Return matching pattern |
in conclusion
Regular expressions are used to match patterns. The above methods are examples of actions you should take when matching patterns. We also demonstrate these methods with two worked examples, demonstrating their power and versatility. By understanding these methods and their use cases, you can use regular expressions to efficiently find matching patterns
The above is the detailed content of Pattern classes in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


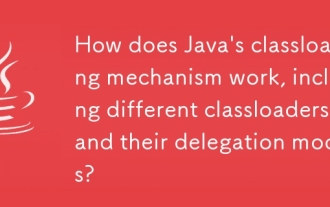
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
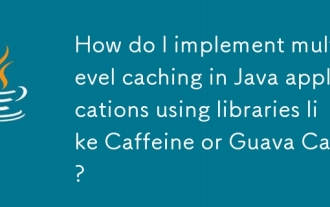
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
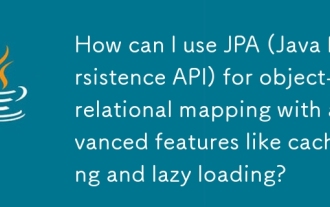
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
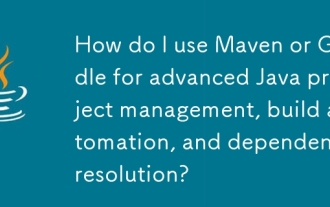
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
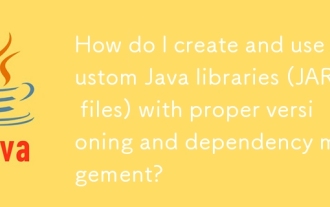
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
