How to search for a pattern in a string in JavaScript?
In this article, we will search for a specific pattern and pass only the strings that match the given pattern. We will use the following method to implement this functionality -
Method 1
In this method we will search for the string matching the given pattern and get it from the string. string.search() is a built-in method provided by JavaScript for searching strings. We can also pass a regular expression or a normal string in this method.
Syntax
str.search( expression )
Parameters
str - Defines the string to be compared.
Expression - Defines a string expression that will be compared to a string
This will return a string The index where the string has started to match, or "-1" will be returned if the string does not match.
Example 1
In the following example, we compare a string with a regular expression and return its index if the string matches. If not, -1 will be returned.
#index.html
<!DOCTYPE html> <html> <head> <title> Creating Objects from Prototype </title> </head> <body> <h2 style="color:green"> Welcome To Tutorials Point </h2> </body> <script> const paragraph = 'Start your learning journey with tutorials point today!'; // any character that is not a word character or whitespace const regex = /(lear)\w+/g; console.log("Index: " + paragraph.search(regex)); console.log("Alphabet start: " + paragraph[paragraph.search(regex)]); // expected output: "." </script> </html>
Output
Example 2
below In the example, we create multiple regular expressions and check whether they meet the requirements on the string.
# index.html
<!DOCTYPE html> <html> <head> <title> Creating Objects from Prototype </title> </head> <body> <h2 style="color:green"> Welcome To Tutorials Point </h2> </body> <script> const paragraph = 'Start your learning journey with tutorials point today!'; // any character that is not a word character or whitespace const regex = /(lear)\w+/g; const regex1 = /(!)\w+/g; const regex2 = /(!)/g; console.log("Index: " + paragraph.search(regex)); console.log("Index: " + paragraph.search(regex1)); console.log("Index: " + paragraph.search(regex2)); console.log("Alphabet start: " + paragraph[paragraph.search(regex)]); // expected output: "." </script> </html>
output
The above is the detailed content of How to search for a pattern in a string in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
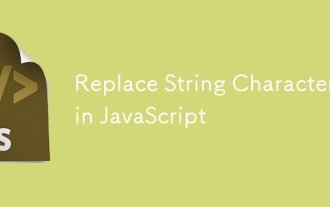
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
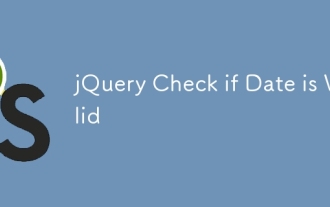
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
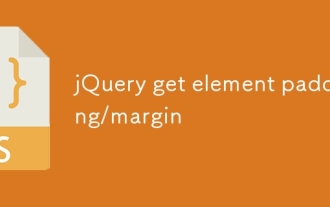
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
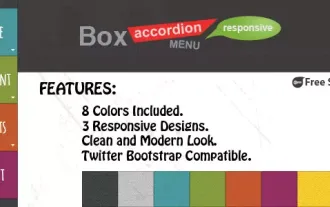
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
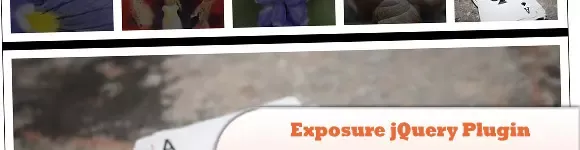
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
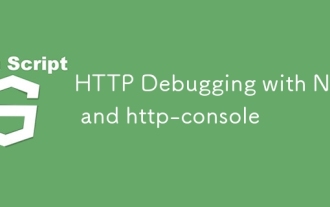
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
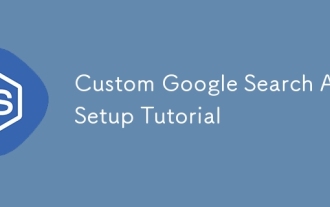
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
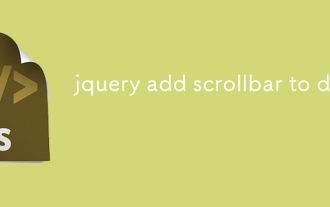
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c
