


Translate the following into Chinese: C++ Divide a number into two divisible parts
In this question we are given a string that can be interpreted as a number. Now we have to divide this string into two parts such that the first part is divisible by A and the second part is divisible by B (given our two integers). For example -
Input : str = "123", a = 12, b = 3 Output : YES 12 3 "12" is divisible by a and "3" is divisible by b. Input : str = "1200", a = 4, b = 3 Output : YES 12 00 Input : str = "125", a = 12, b = 3 Output : NO
Now, in this problem, we will do some pre-computation to make our program faster and then it will be able to work under higher constraints.
Method to find solution
In this method we will run two loops in the string, first loop from start to end and second loop from end to start. Now, at each point, we take modulo the integer formed by an in the first loop and b in the second loop, and then we can find the answer.
Example
#include <bits/stdc++.h> using namespace std; void divisionOfString(string &str, int a, int b){ int n = str.length(); vector<int> mod_a(n+1, 0); // mod_a[0] = (str[0] - '0')%a; for (int i=1; i<n; i++) // front loop for calculating the mod of integer with a mod_a[i] = ((mod_a[i-1]*10)%a + (str[i]-'0'))%a; vector<int> mod_b(n+1, 0); mod_b[n-1] = (str[n-1] - '0')%b; int power10 = 10; // as we have assigned answer to last index for (int i= n-2; i>=0; i--){ // end loop for calculating the mod of integer with b mod_b[i] = (mod_b[i+1] + (str[i]-'0')*power10)%b; power10 = (power10 * 10) % b; } for (int i=0; i<n-1; i++){ // finding the division point if (mod_a[i] != 0) // we can skip through all the positions where mod_a is not zero continue; if (mod_b[i+1] == 0){ // now if the next index of mod_b is also zero so that is our division point cout << "YES\n"; /*******Printing the partitions formed**********/ for (int k=0; k<=i; k++) cout << str[k]; cout << " "; for (int k=i+1; k < n; k++) cout << str[k]; return; } } cout << "NO\n"; // else we print NO } // Driver code int main(){ string str = "123"; // given string int a = 12, b = 3; divisionOfString(str, a, b); return 0; }
Output
YES 12 3
Explanation of the above code
In this method, we now calculate the remainder of the number formed by each division. Our first number should be divisible by a, so we run a forward loop and store the mod of that number with a. For b, we run a backward loop and now store the mod because we know that if the mod of an at any position is zero, and the mod of b at the next index is zero, this will be our answer, so we print it .
Conclusion
In this tutorial we solved a problem of dividing a number into two divisible parts. We also learned the C program for this problem and the complete method (general) to solve it. We can write the same program in other languages such as C, java, python and other languages. We hope you found this tutorial helpful.
The above is the detailed content of Translate the following into Chinese: C++ Divide a number into two divisible parts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
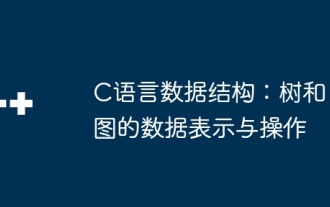
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
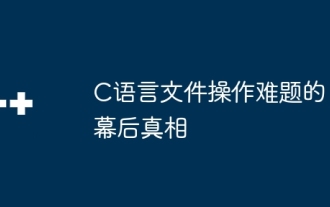
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
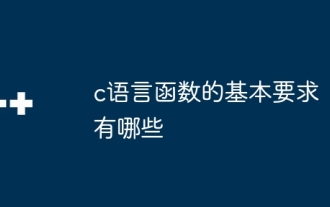
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
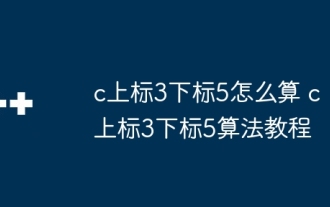
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
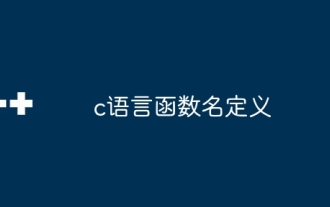
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
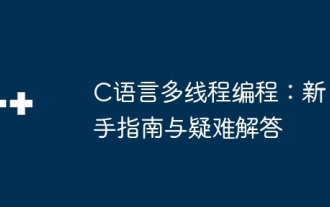
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
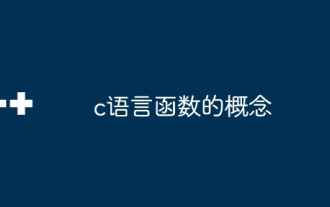
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
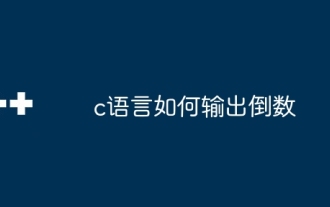
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
