C++ program creates a function with parameters and return value
Any programming language that uses functions has code that is simpler, more modular, and easier to change while debugging. Functions are very useful components in modular code. The ability of a function to accept parameters and output results. Functions don't necessarily need to accept input and always produce a result. In many cases, functions only accept some input and return nothing. Not always responsive and will not tolerate controversy. This article explains how to create a C program that uses functions that accept multiple parameters and produce a result after processing.
Function with parameters and return value
To define a function that takes several parameters and returns a value to the caller function (the caller function is the caller function that calls our function to perform some operation), the return type must be a specific type, not void , and the given parameter list must be present in the parameter list
grammar
<return type> function_name ( <type1> argument1, <type2> argument2, … ) { // function body }
In the following example, we pass a number as a parameter, then calculate the factorial of the given number and return the result. Let's look at the algorithm and implementation in C.
algorithm
- Define a function Factorial(), which will take n as a parameter
- Facts: = 1
- When n > 1; do
- fact = fact * n
- n = n - 1
- Finish
- Return facts
- End function body
- Call Factorial() and pass n to find the factorial of n
Example
#include <iostream> using namespace std; long factorial( int n ) { long fact = 1; while ( n > 1 ) { fact = fact * n; n = n - 1; } return fact; } int main() { cout << "Factorial of 6 is: "; long res = factorial( 6 ); cout << res << endl; cout << "Factorial of 8 is: "; res = factorial( 8 ); cout << res << endl; cout << "Factorial of 12 is: "; res = factorial( 12 ); cout << res << endl; }
Output
Factorial of 6 is: 720 Factorial of 8 is: 40320 Factorial of 12 is: 479001600
Another example of using a function to check if a number is a palindrome. We pass a number as parameter and the function will return true when it is a palindrome and false when it is not a palindrome.
algorithm
- Define a function solve(), which will require n
- Sum: = 0
- temp = n;
- When n > 0, execute
- rem := n mod 10
- Sum := (sum * 10) rem
- n := Lower limit of (n / 2)
- Finish
- If sum is the same as temp, then
- return true
- otherwise
- Return error
- If it ends
Example
#include <iostream> #include <sstream> using namespace std; string solve( int n ) { int sum = 0; int temp = n; int rem; while( n > 0) { rem = n % 10; sum = (sum * 10) + rem; n = n / 10; } if( temp == sum ) { return "true"; } else { return "false"; } } int main() { cout << "Is 153 a palindrome? " << solve( 153 ) << endl; cout << "Is 15451 a palindrome? " << solve( 15451 ) << endl; cout << "Is 979 a palindrome? " << solve( 979 ) << endl; }
Output
Is 153 a palindrome? false Is 15451 a palindrome? true Is 979 a palindrome? true
in conclusion
Using functions when writing code modularizes the code and has several advantages when debugging or working with other people's code. There are different function patterns, sometimes taking parameters from the caller function and returning the results to the caller function. Sometimes it takes no input but returns a value. In this article, we saw through a few examples how to write a function that takes parameters and returns a value to the caller function. Using functions is very simple and easy to implement. It's always good to use functions when writing code, as this can reduce unnecessary code duplication in many applications.
The above is the detailed content of C++ program creates a function with parameters and return value. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


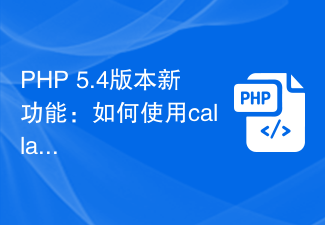
New feature of PHP5.4 version: How to use callable type hint parameters to accept callable functions or methods Introduction: PHP5.4 version introduces a very convenient new feature - you can use callable type hint parameters to accept callable functions or methods . This new feature allows functions and methods to directly specify the corresponding callable parameters without additional checks and conversions. In this article, we will introduce the use of callable type hints and provide some code examples,
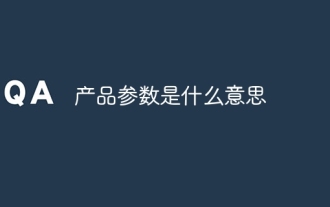
Product parameters refer to the meaning of product attributes. For example, clothing parameters include brand, material, model, size, style, fabric, applicable group, color, etc.; food parameters include brand, weight, material, health license number, applicable group, color, etc.; home appliance parameters include brand, size, color , place of origin, applicable voltage, signal, interface and power, etc.
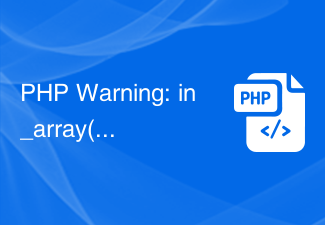
During the development process, we may encounter such an error message: PHPWarning: in_array()expectsparameter. This error message will appear when using the in_array() function. It may be caused by incorrect parameter passing of the function. Let’s take a look at the solution to this error message. First, you need to clarify the role of the in_array() function: check whether a value exists in the array. The prototype of this function is: in_a
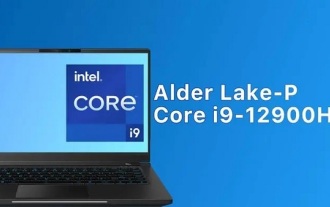
i9-12900H is a 14-core processor. The architecture and technology used are all new, and the threads are also very high. The overall work is excellent, and some parameters have been improved. It is particularly comprehensive and can bring users Excellent experience. i9-12900H parameter evaluation review: 1. i9-12900H is a 14-core processor, which adopts the q1 architecture and 24576kb process technology, and has been upgraded to 20 threads. 2. The maximum CPU frequency is 1.80! 5.00ghz, which mainly depends on the workload. 3. Compared with the price, it is very suitable. The price-performance ratio is very good, and it is very suitable for some partners who need normal use. i9-12900H parameter evaluation and performance running scores
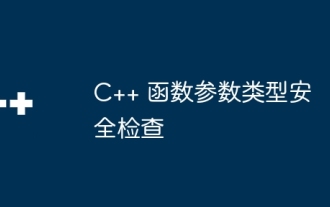
C++ parameter type safety checking ensures that functions only accept values of expected types through compile-time checks, run-time checks, and static assertions, preventing unexpected behavior and program crashes: Compile-time type checking: The compiler checks type compatibility. Runtime type checking: Use dynamic_cast to check type compatibility, and throw an exception if there is no match. Static assertion: Assert type conditions at compile time.
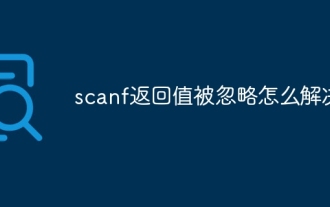
Solutions to the ignored return value of scanf include checking the return value of scanf, clearing the input buffer, and using fgets instead of scanf. Detailed introduction: 1. Check the return value of scanf. You should always check the return value of the scanf function. The return value of the scanf function is the number of successfully read parameters. If the return value is inconsistent with the expected one, it means that the input is incorrect; 2 , Clear the input buffer. When using the scanf function, if the input data does not match the expected format, the data in the input buffer will be lost, etc.
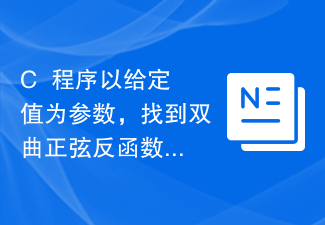
Hyperbolic functions are defined using hyperbolas instead of circles and are equivalent to ordinary trigonometric functions. It returns the ratio parameter in the hyperbolic sine function from the supplied angle in radians. But do the opposite, or in other words. If we want to calculate an angle from a hyperbolic sine, we need an inverse hyperbolic trigonometric operation like the hyperbolic inverse sine operation. This course will demonstrate how to use the hyperbolic inverse sine (asinh) function in C++ to calculate angles using the hyperbolic sine value in radians. The hyperbolic arcsine operation follows the following formula -$$\mathrm{sinh^{-1}x\:=\:In(x\:+\:\sqrt{x^2\:+\:1})}, Where\:In\:is\:natural logarithm\:(log_e\:k)
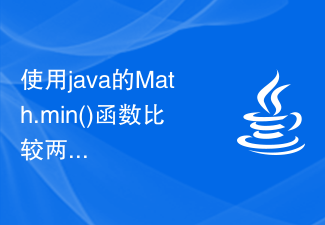
Use Java's Math.min() function to compare the sizes of two numerical values and return the smaller value. When developing Java applications, sometimes we need to compare the sizes of two numerical values and return the smaller number. Java provides the Math.min() function to implement this function. The Math.min() function is a static method of the JavaMath class. It is used to compare the size of two values and return the smaller number. Its syntax is as follows: publicstaticintmi
