Java program to calculate the area of a triangle using determinants
Introduction
The Java program for calculating the area of a triangle using determinants is a simple and efficient program that can calculate the area of a triangle based on the coordinates of the given three vertices.
This program is useful for anyone learning or working with geometry, as it demonstrates how to use basic arithmetic and algebraic calculations in Java, as well as how to read user input using the Scanner class. The program prompts the user for the coordinates of three points of the triangle, which are then read in and used to calculate the determinant of the coordinate matrix. Use the absolute value of the determinant to ensure the area is always positive, then use a formula to calculate the area of the triangle and display it to the user. The program can be easily modified to accept input in different formats or to perform additional calculations, making it a versatile tool for geometric calculations.
determining factors
The determinant is a mathematical concept used to determine certain properties of a matrix. In linear algebra, a determinant is a scalar value that can be calculated from the elements of a square matrix. Determinants can be used to determine whether a matrix has an inverse, whether a system of linear equations has a unique solution, and the area or volume of a parallelogram or parallelepiped.
grammar
1 |
|
algorithm
Import Scanner class.
Define a public class named TriangleArea.
Define a main method in the class.
Create a Scanner object to read user input.
Prompts the user to enter the coordinates of three points separated by spaces.
Read the coordinates entered by the user and store them in six double variables (x1, y1, x2, y2, x3, y3).
Use the formula to calculate the determinant of the coordinate matrix -
Then we calculate the area of the triangle using the formula -
1 2 3 |
|
1 |
|
Example 1
method
First, we prompt the user to enter the coordinates of the three points of the triangle.
We use the Scanner class to read the coordinates entered by the user and store them in six double variables (x1, y1, x2, y2, x3, y3).
Next, we use the formula to calculate the determinant of the coordinate matrix -
1 2 3 |
|
Then we calculate the area of the triangle using the formula -
1 |
|
This is a Java program that uses determinants to calculate the area of a triangle -
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
illustrate
Please note that the Math.abs() function is used to ensure that the area is always positive, since the determinant may be negative if the vertices are listed in counterclockwise order.
Output
1 2 3 4 5 |
|
Example 2
This method works with any triangle, regardless of its orientation or size. The program assumes that the user entered valid numeric coordinates for the three points, otherwise an exception may be thrown if the input is invalid.
This is a Java program that uses determinants to calculate the area of a triangle -
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
|
illustrate
The program prompts the user to enter the coordinates of the three points that form the triangle, and then uses the calculateTriangleArea() method to calculate the area of the triangle through the determinant. Finally, it prints the calculated area to the console.
Output
1 2 3 4 |
|
in conclusion
A Java program to calculate the area of a triangle using determinants is a simple and efficient way to calculate the area of a triangle given coordinates. The program uses basic arithmetic and algebraic calculations to determine the determinant of a coordinate matrix and then uses that determinant to calculate the area of a triangle using a simple formula. This program demonstrates how to use the Scanner class for user input, the Math class for mathematical operations, and how to use code organization and modularity.
The time complexity of the program is constant time, which means that it performs a fixed number of operations regardless of the size of the input. This makes it a fast and efficient program for calculating the area of a triangle. The space complexity of the program is also constant because it only uses a fixed amount of memory to store variables and does not require any additional memory allocation.
The above is the detailed content of Java program to calculate the area of a triangle using determinants. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
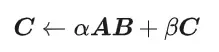
General Matrix Multiplication (GEMM) is a vital part of many applications and algorithms, and is also one of the important indicators for evaluating computer hardware performance. In-depth research and optimization of the implementation of GEMM can help us better understand high-performance computing and the relationship between software and hardware systems. In computer science, effective optimization of GEMM can increase computing speed and save resources, which is crucial to improving the overall performance of a computer system. An in-depth understanding of the working principle and optimization method of GEMM will help us better utilize the potential of modern computing hardware and provide more efficient solutions for various complex computing tasks. By optimizing the performance of GEMM
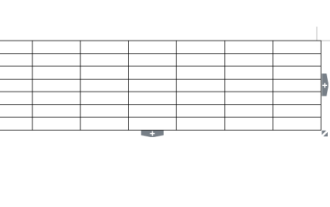
WORD is a powerful word processor. We can use word to edit various texts. In Excel tables, we have mastered the calculation methods of addition, subtraction and multipliers. So if we need to calculate the addition of numerical values in Word tables, How to subtract the multiplier? Can I only use a calculator to calculate it? The answer is of course no, WORD can also do it. Today I will teach you how to use formulas to calculate basic operations such as addition, subtraction, multiplication and division in tables in Word documents. Let's learn together. So, today let me demonstrate in detail how to calculate addition, subtraction, multiplication and division in a WORD document? Step 1: Open a WORD, click [Table] under [Insert] on the toolbar, and insert a table in the drop-down menu.

How to use Python's count() function to calculate the number of an element in a list requires specific code examples. As a powerful and easy-to-learn programming language, Python provides many built-in functions to handle different data structures. One of them is the count() function, which can be used to count the number of elements in a list. In this article, we will explain how to use the count() function in detail and provide specific code examples. The count() function is a built-in function of Python, used to calculate a certain

Introduction The Java program for calculating the area of a triangle using determinants is a concise and efficient program that can calculate the area of a triangle given the coordinates of three vertices. This program is useful for anyone learning or working with geometry, as it demonstrates how to use basic arithmetic and algebraic calculations in Java, as well as how to use the Scanner class to read user input. The program prompts the user for the coordinates of three points of the triangle, which are then read in and used to calculate the determinant of the coordinate matrix. Use the absolute value of the determinant to ensure the area is always positive, then use a formula to calculate the area of the triangle and display it to the user. The program can be easily modified to accept input in different formats or to perform additional calculations, making it a versatile tool for geometric calculations. ranks of determinants
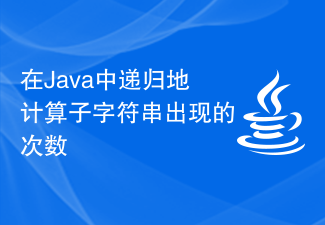
Given two strings str_1 and str_2. The goal is to count the number of occurrences of substring str2 in string str1 using a recursive procedure. A recursive function is a function that calls itself within its definition. If str1 is "Iknowthatyouknowthatiknow" and str2 is "know" the number of occurrences is -3. Let us understand through examples. For example, input str1="TPisTPareTPamTP", str2="TP"; output Countofoccurrencesofasubstringrecursi
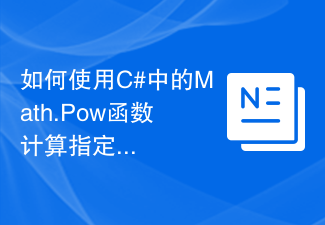
In C#, there is a Math class library, which contains many mathematical functions. These include the function Math.Pow, which calculates powers, which can help us calculate the power of a specified number. The usage of the Math.Pow function is very simple, you only need to specify the base and exponent. The syntax is as follows: Math.Pow(base,exponent); where base represents the base and exponent represents the exponent. This function returns a double type result, that is, the power calculation result. Let's
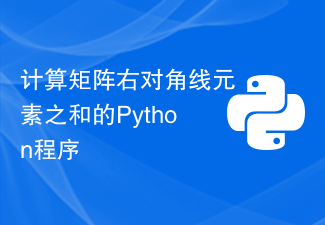
A popular general-purpose programming language is Python. It is used in a variety of industries, including desktop applications, web development, and machine learning. Fortunately, Python has a simple and easy-to-understand syntax that is suitable for beginners. In this article, we will use Python to calculate the sum of the right diagonal of a matrix. What is a matrix? In mathematics, we use a rectangular array or matrix to describe a mathematical object or its properties. It is a rectangular array or table containing numbers, symbols, or expressions arranged in rows and columns. . For example -234512367574 Therefore, this is a matrix with 3 rows and 4 columns, expressed as a 3*4 matrix. Now, there are two diagonals in the matrix, the primary diagonal and the secondary diagonal
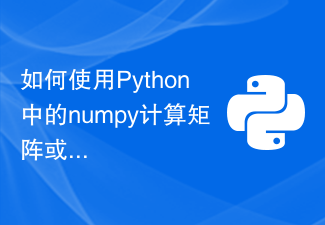
In this article, we will learn how to calculate the determinant of a matrix using the numpy library in Python. The determinant of a matrix is a scalar value that can represent the matrix in compact form. It is a useful quantity in linear algebra and has numerous applications in various fields including physics, engineering, and computer science. In this article, we will first discuss the definition and properties of determinants. We will then learn how to use numpy to calculate the determinant of a matrix and see how it is used in practice through some examples. Thedeterminantofamatrixisascalarvaluethatcanbeusedtodescribethepropertie
