How to update the _id of a MongoDB document?
You can't update it, but you can save the new ID and delete the old one. Follow some steps to update MongoDB's _id. The steps are as follows:
Step 1: In the first step, the ObjectId needs to be stored in a variable.
anyVariableName=db.yourCollectionName.findOne({_id:yourObjectIdValue)});
Step 2: In the second step, you need to set a new id.
yourDeclaredVariableName._id=yourNewObjectIdValue;
Step 3: In the third step, you need to insert the new ID in the document.
db.yourCollectionName.insert(yourDeclaredVariableName);
Step 4: In the fourth step, you need to delete the old ID.
db.yourCollectionName.remove({_id:yourOldObjectIdValue)});
To understand the above steps, let us create a collection with documents. The query to create a collection using documents is as follows:
> db.updateIdDemo.insertOne({"StudentName":"Robert"}); { "acknowledged" : true, "insertedId" : ObjectId("5c6ebfec6fd07954a4890683") } > db.updateIdDemo.insertOne({"StudentName":"Chris"}); { "acknowledged" : true, "insertedId" : ObjectId("5c6ebff66fd07954a4890684") } > db.updateIdDemo.insertOne({"StudentName":"Maxwell"}); { "acknowledged" : true, "insertedId" : ObjectId("5c6ebfff6fd07954a4890685") }
Display all documents in the collection with the help of find() method. The query is as follows:
> db.updateIdDemo.find().pretty();
The following is the output:
{ "_id" : ObjectId("5c6ebfec6fd07954a4890683"), "StudentName" : "Robert" } { "_id" : ObjectId("5c6ebff66fd07954a4890684"), "StudentName" : "Chris" } { "_id" : ObjectId("5c6ebfff6fd07954a4890685"), "StudentName" : "Maxwell" }
The following is the query to update the _id of a MongoDB document:
Step1: > myId=db.updateIdDemo.findOne({_id:ObjectId("5c6ebfec6fd07954a4890683")}); { "_id" : ObjectId("5c6ebfec6fd07954a4890683"), "StudentName" : "Robert" } Step 2: > myId._id=ObjectId("5c6ebfec6fd07954a4890689"); ObjectId("5c6ebfec6fd07954a4890689") Step 3: > db.updateIdDemo.insert(myId); WriteResult({ "nInserted" : 1 }) Step 4: > db.updateIdDemo.remove({_id:ObjectId("5c6ebfec6fd07954a4890683")}); WriteResult({ "nRemoved" : 1 })
Let us check if the _id has been updated. Display all documents in the collection with the help of find() method:
> db.updateIdDemo.find().pretty();
The following is the output:
{ "_id" : ObjectId("5c6ebff66fd07954a4890684"), "StudentName" : "Chris" } { "_id" : ObjectId("5c6ebfff6fd07954a4890685"), "StudentName" : "Maxwell" } { "_id" : ObjectId("5c6ebfec6fd07954a4890689"), "StudentName" : "Robert" }
View the sample output, "StudentName":_id of "Robert" corrected.
The above is the detailed content of How to update the _id of a MongoDB document?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


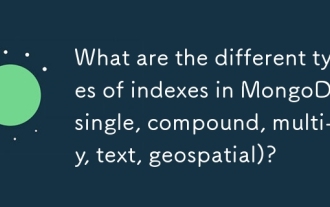
The article discusses various MongoDB index types (single, compound, multi-key, text, geospatial) and their impact on query performance. It also covers considerations for choosing the right index based on data structure and query needs.
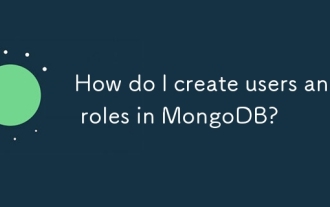
The article discusses creating users and roles in MongoDB, managing permissions, ensuring security, and automating these processes. It emphasizes best practices like least privilege and role-based access control.
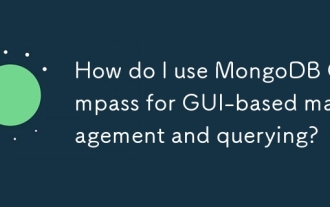
MongoDB Compass is a GUI tool for managing and querying MongoDB databases. It offers features for data exploration, complex query execution, and data visualization.
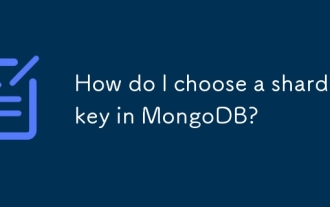
The article discusses selecting a shard key in MongoDB, emphasizing its impact on performance and scalability. Key considerations include high cardinality, query patterns, and avoiding monotonic growth.

The article discusses configuring MongoDB auditing for security compliance, detailing steps to enable auditing, set up audit filters, and ensure logs meet regulatory standards. Main issue: proper configuration and analysis of audit logs for security
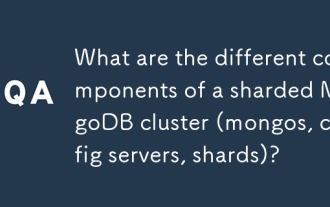
The article discusses components of a sharded MongoDB cluster: mongos, config servers, and shards. It focuses on how these components enable efficient data management and scalability.
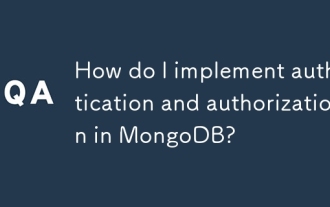
The article guides on implementing and securing MongoDB with authentication and authorization, discussing best practices, role-based access control, and troubleshooting common issues.
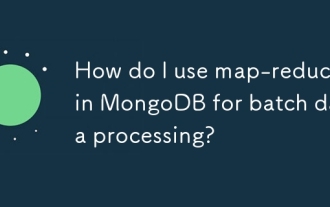
The article explains how to use map-reduce in MongoDB for batch data processing, its performance benefits for large datasets, optimization strategies, and clarifies its suitability for batch rather than real-time operations.
