


What is the purpose of the 'enumerate()' function in Python?
In this article, we will learn about enumerate() function and the purpose of “enumerate()” function in Python.
What is the enumerate() function?
Python’s enumerate() function accepts a data collection as a parameter and returns an enumeration object.
The enumeration object is returned in the form of key-value pairs. The key is the index corresponding to each item, and the value is the items.
grammar
enumerate(iterable, start)
parameter
iterable - The passed in data collection can be returned as an enumeration object, called iterable
start - As the name suggests, the starting index of the enumeration object is defined by start. If we omit this value, it will consider the first index to be zero since zero is the default value here
Usage of enumerate() function in Python
When to use the enumerate() function?
If you need the index of the iterated value, use the enumerate() function.
how to use?
The enumeration function assigns the index of each iterable value to the list.
Why use the enumerate() function?
When using iterators, we always need to know how many iterations have been completed, that is, the number of iterations.
However, we have a Pythonic way to determine the necessary number of iterations. The syntax is as follows -
enumerate(iterable, start)
How to use enumerate() function in Python?
It is important to note which data type will be used to store the enumeration object after returning.
Example
The following program uses the enumerate() function on a list to get a list of tuples with indexes -
# input list inputList = ["hello", "tutorialspoint", "python", "codes"] # getting the enumerate object of the input list enumerate_obj = enumerate(inputList) print(enumerate_obj) # converting the enumerate object into a list and printing it print(list(enumerate_obj))
Output
When executed, the above program will generate the following output -
<enumerate object at 0x7f4e56b553c0> [(0, 'hello'), (1, 'tutorialspoint'), (2, 'python'), (3, 'codes')]
Use the second parameter "starting index" of the enumerate() function
Example
The following program shows how to apply the enumerate() function to a list containing a second argument -
# input list inputList = ["hello", "tutorialspoint", "python", "codes"] # getting the enumerate object starting from the index 15 enumerate_obj = enumerate(inputList, 15) # converting the enumerate object into the list and printing it print(list(enumerate_obj))
Output
When executed, the above program will generate the following output -
[(15, 'hello'), (16, 'tutorialspoint'), (17, 'python'), (18, 'codes')]
In the above example, we added 15 as the second parameter in the enumerate() function. The second parameter will indicate the starting index of the key (index) in the enumerator object, so we can see in the output that the first index is 15, the second index is 16, and so on.
Python code for looping through enumerated objects
Example
The following program shows how to use a for loop to iterate through an enumeration object and print each element in it -
# input list inputList = ["hello", "tutorialspoint", "python", "codes"] # getting the enumerate object enumerate_obj = enumerate(inputList) # traversing through each item in an enumerate object for i in enumerate_obj: # printing the corresponding iterable item print(i)
Output
When executed, the above program will generate the following output -
(0, 'hello') (1, 'tutorialspoint') (2, 'python') (3, 'codes')
Use the enumerate() function on tuples
Example
The following program shows how to use the enumerate() function on a given input tuple -
# input tuple inputTuple = ("hello", "tutorialspoint", "python", "codes") # getting the enumerate object of the input tuple enumerate_obj = enumerate(inputTuple) print(enumerate_obj) # converting the enumerate object into the list and printing it print(list(enumerate_obj))
Output
When executed, the above program will generate the following output -
<enumerate object at 0x7fec12856410> [(0, 'hello'), (1, 'tutorialspoint'), (2, 'python'), (3, 'codes')]
Using the enumerate() function on strings
Iterate over string data using enumeration functions to determine the index of each character.
Example
The following program shows how to use the enumerate() function on a given input string -
# input string inputString = "python" # getting the enumerate object of an input string enumerate_obj = enumerate(inputString) # converting the enumerate object into the list and printing it print(list(enumerate_obj))
Output
When executed, the above program will generate the following output -
[(0, 'p'), (1, 'y'), (2, 't'), (3, 'h'), (4, 'o'), (5, 'n')]
Use another parameter in the enumerate() function
Example
The following program shows how to use another parameter in the enumerate() function -
input_data = ('Hello', 'TutorialsPoint', 'Website') for count, dataValue in enumerate(input_data,start = 50): print(count, dataValue)
Output
When executed, the above program will generate the following output -
(50, 'Hello') (51, 'TutorialsPoint') (52, 'Website')
Enumerate is a Python built-in function that returns an enumeration object.
This function returns an enumeration object.
To pass an iterable object to an enumeration function, first try to retrieve the data from the enumeration object and then convert it to list(). As a result, as shown in the example above, it returns a list of tuples along with the iteration index.
in conclusion
This article introduces the application of Python enumerate() function in detail. We also learned how to use it with various Python data types and iteration. Additionally, we also learned how to modify the starting count or index of the enumerate() function.
The above is the detailed content of What is the purpose of the 'enumerate()' function in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


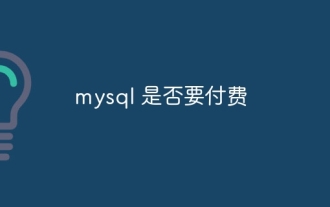
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
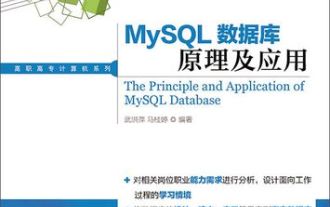
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
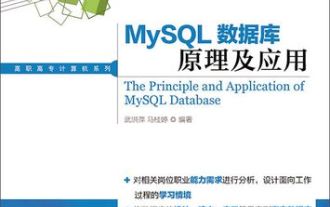
MySQL download file is corrupt, what should I do? Alas, if you download MySQL, you can encounter file corruption. It’s really not easy these days! This article will talk about how to solve this problem so that everyone can avoid detours. After reading it, you can not only repair the damaged MySQL installation package, but also have a deeper understanding of the download and installation process to avoid getting stuck in the future. Let’s first talk about why downloading files is damaged. There are many reasons for this. Network problems are the culprit. Interruption in the download process and instability in the network may lead to file corruption. There is also the problem with the download source itself. The server file itself is broken, and of course it is also broken when you download it. In addition, excessive "passionate" scanning of some antivirus software may also cause file corruption. Diagnostic problem: Determine if the file is really corrupt
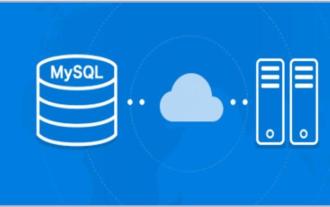
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
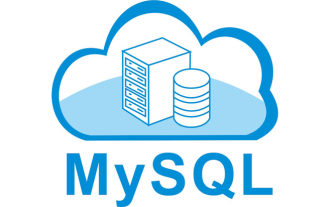
MySQL refused to start? Don’t panic, let’s check it out! Many friends found that the service could not be started after installing MySQL, and they were so anxious! Don’t worry, this article will take you to deal with it calmly and find out the mastermind behind it! After reading it, you can not only solve this problem, but also improve your understanding of MySQL services and your ideas for troubleshooting problems, and become a more powerful database administrator! The MySQL service failed to start, and there are many reasons, ranging from simple configuration errors to complex system problems. Let’s start with the most common aspects. Basic knowledge: A brief description of the service startup process MySQL service startup. Simply put, the operating system loads MySQL-related files and then starts the MySQL daemon. This involves configuration
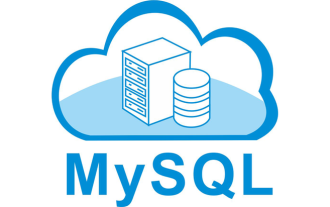
MySQL performance optimization needs to start from three aspects: installation configuration, indexing and query optimization, monitoring and tuning. 1. After installation, you need to adjust the my.cnf file according to the server configuration, such as the innodb_buffer_pool_size parameter, and close query_cache_size; 2. Create a suitable index to avoid excessive indexes, and optimize query statements, such as using the EXPLAIN command to analyze the execution plan; 3. Use MySQL's own monitoring tool (SHOWPROCESSLIST, SHOWSTATUS) to monitor the database health, and regularly back up and organize the database. Only by continuously optimizing these steps can the performance of MySQL database be improved.
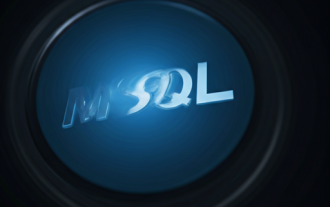
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
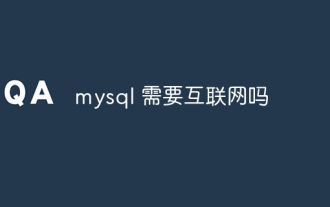
MySQL can run without network connections for basic data storage and management. However, network connection is required for interaction with other systems, remote access, or using advanced features such as replication and clustering. Additionally, security measures (such as firewalls), performance optimization (choose the right network connection), and data backup are critical to connecting to the Internet.
