


Makes the given binary strings equal by repeatedly replacing two consecutive 0's with a single 1
In any programming language, a binary string is a collection of characters 0 and 1. At each stage, the binary string follows the approach that the string can only contain these two characters.
Characters in a continuous string refer to characters whose index difference is 1. Let us consider two indices, i and j, they are said to be continuous if |j-i| = 1.
In C, if two strings are equivalent, it means:
The corresponding characters in the two strings are the same.
The lengths of the strings are equal, and the characters at the corresponding indexes overlap.
Some examples to illustrate the problem statement are as follows -
ExampleExample
str1 - "10001"
str2 - "101"
solution-
str1 cannot be converted to str2 because in the process of converting str1 to create the equivalent string str2, we will get str1 as "1011" and str2 as "101".
Example 2 - Let us consider the following input −
str1 - "001"
str2 - "11"
solution-
str1 can be converted to str2 by changing the first two zeros to a 1.
Using character matching and string traversal in C can solve the following problems.
algorithm
Step 1 - Two pointers i and j are used to simultaneously iterate the strings s1 and s2 respectively.
Step 2 - If the characters at both indices match, we increment the i and j pointers.
Step 3 − If the characters are not equal, we check if the character at the i-th and (i 1)th index is '0', and the character at the j-th index Whether it is '1'.
Step 4 - If such a situation exists, conversion can be performed. The i pointer is incremented by two positions and j is incremented by one index position because both zeros are converted to ones.
Step 5 - If the characters are not equal and the above situation does not exist, the conversion cannot be performed.
Step 6 − If both pointers i and j successfully reach the end position, s1 can be converted to s2.
Example
The following code snippet takes two binary strings as input and checks whether the two strings can be equivalent by simple character replacement based on specified conditions
//including the required libraries #include <bits/stdc++.h> using namespace std; //convert consecutive 0's to 1 bool convertBinary(string s1, string s2){ //fetching the lengths of both the strings int len1 = s1.length(); int len2 = s2.length(); string temp =""; //maintaining counters of both the strings int i = 0, j = 0; //iterating over both the strings simultaneously while (i < len1 && j < len2) { //if both the characters are equivalent in nature //skip to next character if (s1[i] == s2[j]) { temp+=s1[i]; //incrementing both pointers i++; j++; } // if both characters differ else { // checking if '00' of s1 can be converted to '1' of s2 if(s2[j]=='1' && s1[i]=='0'){ //checking if i+1th index exists and is equivalent to 0 if(i+1 < len1 && s1[i+1]=='0'){ //conversion is possible //skip two 0's of s1 since converted to 1 in s2 temp+='1'; i += 2; j++; } else { return false; } } // If not possible to combine else { return false; } } } cout<<"Entered string2 "<<s2<<"\n"; cout<<"Converted string1 "<<temp<<"\n"; //check if both the strings are returned to end position if (i == len1 && j == len2) return true; return false; } // calling the conversion rate int main(){ string str1 = "100100"; string str2 = "1111"; //capturing result cout<<"Entered string1 "<<str1<<"\n"; bool res = convertBinary(str1, str2); if (res) cout << "First string can be converted to second"; else cout << "First string can't be converted to second"; return 0; }
Output
Entered string1 100100 Entered string2 1111 Converted string1 1111 First string can be converted to second
in conclusion
Since this method can effectively compare the input string character by character, the time complexity is O(min(string length)). String traversal is an important aspect of solving string problems.
The above is the detailed content of Makes the given binary strings equal by repeatedly replacing two consecutive 0's with a single 1. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
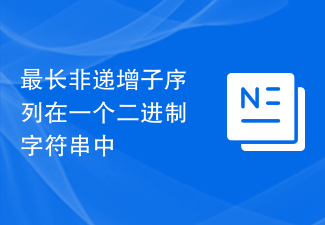
In this problem, we need to find the longest non-increasing subsequence of a given string. Non-increasing means that the characters are either the same or in descending order. Since binary strings only contain "0" and "1", the resulting string should either start with "1" and end with "0", or start and end with "0" or "1". To solve this problem, we will count the prefix "1" and suffix "0" at each position of the string and find the maximum sum of prefix "1" and suffix "0". Problem Statement - We are given a binary string str. We need to find the longest non-increasing subsequence from the given string. Example Input–str="010100"Output–4 illustrates the longest non-recursive
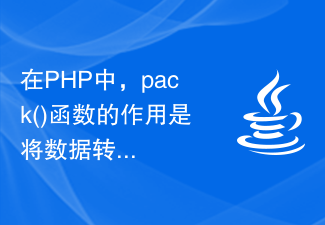
The pack() function packs data into a binary string. Syntax pack(format,args) Parameters format - the format to use. The following are possible values - a - NUL padded string A - space padded string h - hexadecimal string, low nibble first H - hexadecimal string, high nibble first c - signed char C - unsigned char s - signed short (always 16 bits, machine byte order) S - unsigned short (always 16 bits, machine byte order) n - unsigned short (always 16 bits, big endian byte order) v - unsigned short (always 16 bits, little endian byte order) i - signed integer (depends on machine size and byte order) I - None signed integer (depending on
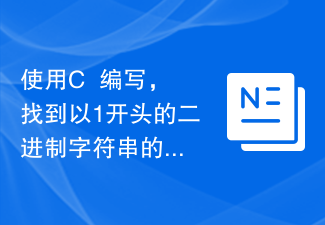
In the given problem, we are given a string consisting of 0 and 1; we need to find the total number of all permutations starting with 1. Since the answer may be a huge number, we take it modulo 1000000007 and output it. Input:str="10101001001"Output:210Input:str="101110011"Output:56 We will solve this problem by applying some combinatorial mathematics and setting up some formulas. Solution Method In this method we will count the number of 0's and 1's. Now suppose n is the number of 1's that appear in our string and m is the number of 0's that appear in our string
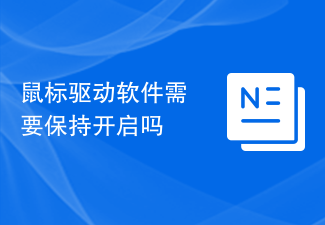
Should the mouse driver always be turned on? The mouse is one of the indispensable input devices in our daily use of computers. In addition to the quality of the hardware itself, the mouse driver is also key to the normal functioning of the mouse. However, many people have some questions about the role and necessity of the mouse driver, especially whether the mouse driver needs to be turned on all the time. First, we need to understand what the mouse driver does. A mouse driver is a software program whose main responsibility is to communicate with the operating system in order to identify and control mouse movements, clicks, and scrolling. The mouse driver can
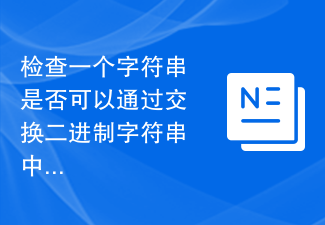
Problem Statement We have a string str and a binary string B. The length of both strings is equal to N. We need to check if we can make string str a palindrome string by swapping its characters multiple times on any pair of indexes containing unequal characters in string B. Example Example Input str=‘AAS’ B=‘101’ Output ‘YES’ The Chinese translation of Explanation is: Explanation We can exchange str[1] and str[2] because B[1] and B[2] are not equal. The final string can be 'ASA'. Input str=‘AASS’ B=‘1111’ and output ‘No’. The Chinese translation of Explanation is: Explanation that we cannot make the string palindrome,
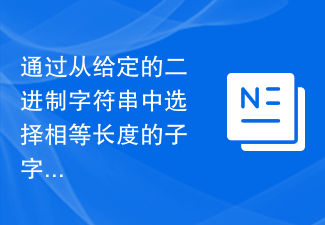
Given two binary strings str1 and str2 of the same length, we have to maximize the given function value by selecting substrings from the given strings of the same length. The given function is like this - fun(str1,str2)=(len(substring))/(2^xor(sub1,sub2)). Here, len(substring) is the length of the first substring and xor(sub1,sub2) is the XOR of the given substring, this is possible since they are binary strings. Example Input1:stringstr1=10110&stringstr2=11101Output:3 illustrates our
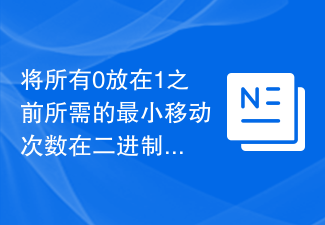
Problem Statement We are given a binary string str and we are required to remove minimum characters from the string so that we can place all zeros before ones. Example input str=‘00110100111’ Output 3 Description Here, we can achieve output 3 in two ways. We can remove arr[2], arr[3] and arr[5] or arr[4], arr[6] and arr[7] from the string. Input str=‘001101011’ and output 2 shows that we can delete arr[4] and arr[6] and put all zeros before 1. Input str=‘000111’ Output 0 means that in the given string, all zeros have been placed before 1, so we don’t need to start from
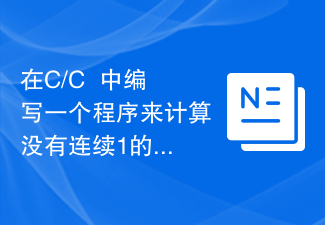
Here we will see an interesting problem. Suppose a value n is given. We must find all strings of length n in which there are no consecutive 1's. If n=2, the numbers are {00,01,10}, so the output is 3. We can use dynamic programming to solve it. Suppose we have a table 'a' and 'b'. where arr[i] stores the number of binary strings of length i that have no consecutive 1's and terminate with 0. Similarly, b is the same but ends in 1. We can add 0 or 1 if the last one is 0, but only add 0 if the last one is 1. Let's look at the algorithm to get this idea. Algorithm noConsecutiveOnes(n)-Begin&am
